Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Besoin de conseils
Debutant en C++
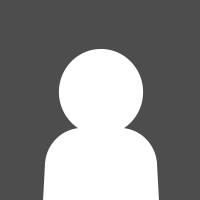
20 septembre 2007 à 13:10:53

20 septembre 2007 à 13:30:38

20 septembre 2007 à 16:10:55
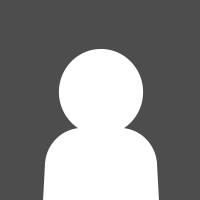
20 septembre 2007 à 22:27:27
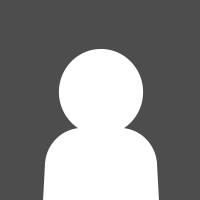
20 septembre 2007 à 22:41:08
C++: Blog|FAQ C++ dvpz|FAQ fclc++|FAQ Comeau|FAQ C++lite|FAQ BS| Bons livres sur le C++| PS: Je ne réponds pas aux questions techniques par MP.
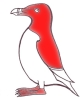
23 septembre 2007 à 15:33:01