Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Model fine tunig bert
en python
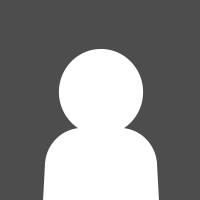
6 avril 2024 à 14:48:32
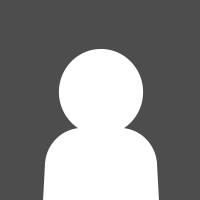
6 avril 2024 à 15:33:17
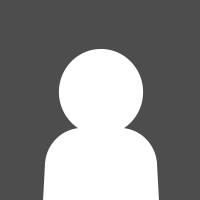
6 avril 2024 à 18:00:18
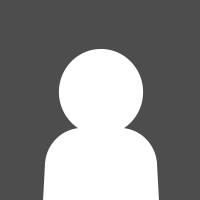
6 avril 2024 à 18:36:53
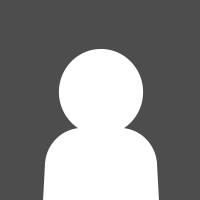
6 avril 2024 à 19:06:21