Forums des Zéros
Une question ? Pas de panique, on va vous aider !
[PyQt4] Signaux, Slots et Drag&Drop
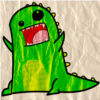
2 novembre 2011 à 18:01:55

2 novembre 2011 à 20:10:30
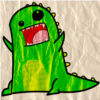
2 novembre 2011 à 20:20:38
Une question ? Pas de panique, on va vous aider !