Forums des Zéros
Une question ? Pas de panique, on va vous aider !
utilisation du printf scanf
Bataille navale et d'autres jeux dans le futur
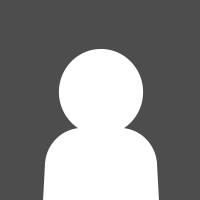
26 décembre 2023 à 10:33:03
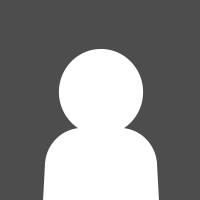
26 décembre 2023 à 11:28:46
...
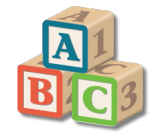
26 décembre 2023 à 11:58:59
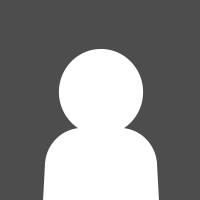
26 décembre 2023 à 13:20:01
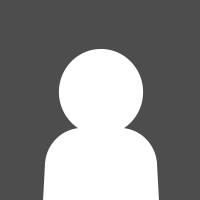
26 décembre 2023 à 16:04:41
...
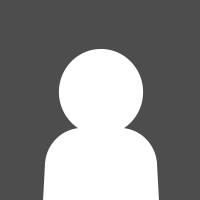
26 décembre 2023 à 16:23:59
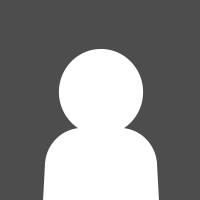
26 décembre 2023 à 16:27:40
...
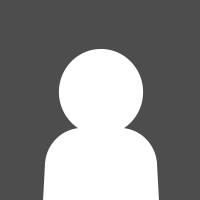
26 décembre 2023 à 16:34:00
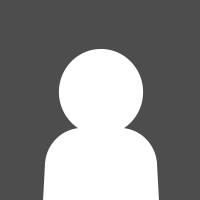
26 décembre 2023 à 16:41:51
...