Since the beginning of this course, you have used different functions, such as the print()
function of the different cast functions like int()
or str()
. We will now take the time to define what a function is, what it is used for and how you can create your own functions—you are going to find out everything there is to know about functions!
Discover Functions
During your data analysis, you will regularly have to use groups of statements several times for a very specific purpose. One of the fundamental principles for any computer programmer is to get maximum results for minimum effort (there is even a saying that a good programmer is a lazy programmer!). It is thanks to this somewhat "lazy" but very effective principle that the idea of functions came about. Functions can group several statements in a block which will be called using a name.
Functions are not specific to Python; they are present in all computer languages. They can:
reuse a portion of code already written just by stating the function name—so you don't have to rewrite the whole portion of code each time.
simplify code and make it more readable!
There are many pre-existing functions in Python! In addition to those already seen, there are, for example:
len()
: a function that returns the length of an item. Do you remember strings? Using this function on a string tells you how many characters the string contains.type()
: lets you print the type of a variable.pow(a, b)
: lets you calculate a to the power of b. It is equivalent to writinga**b
.abs()
: returns the absolute value of a number.
Here are some examples to illustrate the use of these functions:
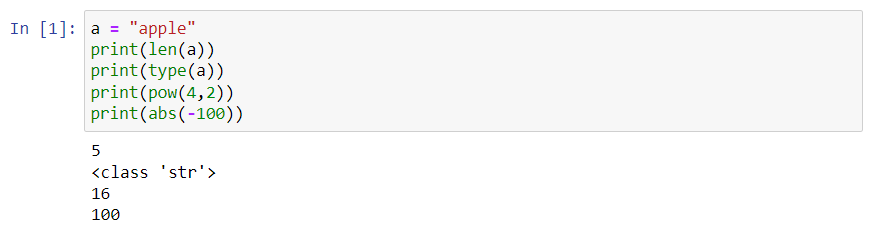
Write Your Own Functions
Now suppose you are asked to develop a program involving geometry. You have to use a lot of triangles for which you have the length of the three sides, and you want to print their perimeter. You could, of course, do this for each triangle by hand, but remember: you want maximum results for the least amount of effort. So, you are going to create a function that will print the perimeter of a triangle according to the length of its sides!
A function is defined via the keyword def
followed by the name of the function. All the statements associated with this function will then be written after the colon.
def functionName():
# statements
# that can go
# on several
# lines
Note that all the statements associated with your function are offset in the code: this is called indentation. Python is a block structured language: each group or block of program must be indented. For example, the set of indented statements following the :
will only be available in functionName
. Indentation starts the block, and "de-indentation" ends it (the first non-indented line will therefore no longer be included in functionName
).
Now define a function that would answer your problem:
def printPerimeter():
dimension1 = 6
dimension2 = 4
dimension3 = 3
perimeter = dimension1 + dimension2 + dimension3
print(perimeter)
printPerimeter() # => 13
This function is correct, but not entirely useful: not all of your triangles will have the exact same dimensions.
Define the Parameters
To overcome this limitation, you must make your function accept external numbers. You can do this by defining parameters.
In Python, parameters, just like the name of the function, are defined when the function is written. This is how it would look with the above example:
def printPerimeter(dimension1, dimension2, dimension3):
perimeter = dimension1 + dimension2 + dimension3
print(perimeter)
Parameters are variables defined during the declaration of the function, specified inside brackets. Now you can use your function with any existing triangle:
printPerimeter(10, 11, 4) # => 25
printPerimeter(2, 2, 3.5) # => 7.5
Each value is assigned to a parameter, in the order in which they were defined. For example, in the first test:
the variable
dimension1
will have a value of 10.the variable
dimension2
will have a value of 11.the variable
dimension3
will have a value of 4.
The function will then perform all the operations specified in the body of the function (i.e., the indented lines) with these values.
Parameters are variables declared in a function. The values that are passed as parameters are called arguments.
So, that's great, you've added some features to your function!
Now, what can I do with the result?
Often, when you use a function in a code, you expect an answer that you can reuse to move forward in the code. This answer can be provided via the value returned by a function.
Define a Return Value
To define a return value, you must explicitly use the return keyword at the end of your function.
You could change your printPerimeter
function into calculatePerimeter
which will return the perimeter of a triangle, according to the length of its three sides, so that it can be reused afterwards:
def calculatePerimeter(dimension1, dimension2, dimension3):
perimeter = dimension1 + dimension2 + dimension3
return perimeter
Once you have defined your function, you can use it as many times as necessary:
perimeter1 = calculatePerimeter(6, 4, 3)
perimeter2 = calculatePerimeter(10, 3, 11)
print("The perimeter of my first triangle is", perimeter1, "and that of my second is", perimeter2)
And if you analyze these lines, you will realize that every time you use the print
function, you send the items to be printed as parameters.
Use the Help Function if You Forget
In your experience in data analysis, you will often remember the name of a function, but not necessarily what it does or its arguments, etc. Don't panic! The help
function is there for that! If you run help(functionName)
, this will print the documentation of this function, summarizing:
its purpose.
recommendations for use.
the list and description of the parameters.
sometimes even examples.
Here is an example with the power function (pow
) seen above:

Phew, you've learned a lot in very little time. What progress!
Let's Recap
In this chapter, you have seen that:
functions can have parameters and return values.
a return value is the result of running the function. The return value is returned to the code that called the function, to be used as needed.
the parameters are the data necessary for a function to be run and generate a result.
parameters are variables defined by a name. Parameters are specified in the function declaration.
when using a function, you pass it different values as parameters. These values are called arguments.
you can use the help function to print the documentation of a given function.
In the next chapter, you will discuss the concept of object-oriented programming and what it means for you in Python.