What Is a List and Why Use it?
Apples, oranges, pears. 🍎🍊🍐 Dogs, cats, rabbits. 🐕🐈🐇 You use lists all the time in your everyday life, and it's no different in Python. You would need a list to store a collection of related objects you want to access later on.
In Python, you use square brackets []
to denote a list. The following code creates a list of different social media platforms and saves it to a variable called social_platforms
.
social_platforms = ["Facebook", "Instagram", "Snapchat", "Twitter"]
Now that the list is saved, you need to be able to access the elements inside.
Access Elements in a List
To access elements in a list, you use an index. Each element has an index related to it, depending on its position in the list. You get the value at that index with the following syntax: list[index]
, which will give you the value in the list at that index.
The important thing to note here is that in most programming languages, including Python, indices start at 0, not 1. So if you want to access the first element of the above list, you’d need to type:
>> social_platforms[0]
"Facebook"
To get the second element, type:
>> social_platforms[1]
"Instagram"
...and so on.
In Python specifically, you can also access elements backward using negative numbers. To get the last element of a list, use the -1 index.
>> social_platforms[-1]
"Twitter"
Access Characters in a String as Elements of a List
Strings can be indexed, too! In fact, Python strings are really just lists of characters. Each character is given an index from zero to the length of the string.
For example, the string language = "PYTHON"
, language[2]
would give you T
, because at index position 2 in the word "PYTHON" you find the character "T." Or, using inverse the index position, youwould use language[-4]
to access T
.
programming_language = "PYTHON"
>> programming_language[2]
"T"
>> programming_language[-4]
"T"
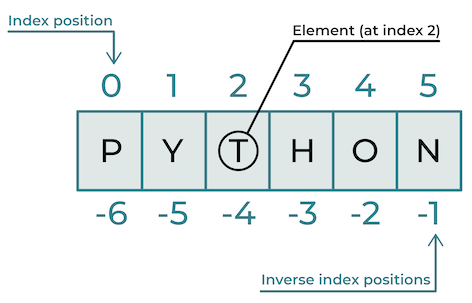
Append, Remove, and Sort Lists
Python makes it easy to do all sorts of operations on lists. Instead of creating a whole new list every time you want to add, remove, or sort it, you can call a list method. We’ll go over methods more later, but for now, all you need to know is that a method is a way to perform a specific operation on an item.
For example, if you want to add a social media platform to the end of the existing list, you can use the append()
method:
>> social_platforms.append("TikTok")
>> print(social_platforms)
["Facebook", "Instagram", "Snapchat", "Twitter", "TikTok"]
To remove a specific element from a list, you can use the .remove()
method.
>> social_platforms.remove("Snapchat")
>> print(social_platforms)
["Facebook", "Instagram", "Twitter", "TikTok"]
.remove()
only removes the first instance of the term that you input to remove.
To find out the length of the list, use the len()
method.
>> len(social_platforms)
4
The last method we'll look at is sort()
, which will sort the elements of a list. This will work alphabetically for lists of strings, and numerically for lists of numbers.
>> social_platforms.sort()
["Facebook", "Instagram", "TikTok", "Twitter"]
Discover Tuples
Tuples are another Python structure that can be used to store data, although instead of brackets []
, they are defined using parentheses ()
.
social_platforms_tuple = ("Facebook", "Instagram", "TikTok", "Twitter")
Many tuple properties are similar to those of a regular list. For example, both lists and tuples can be indexed. The main difference is that tuples are immutable (can't be modified), while lists are mutable (can be modified).
Here's a short list of opportunities to put your new list knowledge into practice! ✅ ✅ ✅
Level-Up: Store Groups of Data Using Lists
Context:
You will now explore the basics of lists in Python in this exercise. You will learn how to create lists, access their elements, modify them, and perform common operations such as adding, removing, and sorting. This knowledge of lists is essential for manipulating and organizing data efficiently in your Python programs.
Instructions:
Create a list named
fruits
containing the elementsapple
,banana
, andorange
.Add
kiwi
to thefruits
list.Remove
orange
from thefruits
list.Modify the second element of the
fruits
list topineapple
.Display the length of the
fruits
list.Sort the fruits listalphabetically and display it.
Once you have completed the exercise, you can run the following command in the VS code terminal pytest tests.py
Let’s Recap!
A list is a way to store multiple items together.
Access elements in a list through its index, which starts at 0.
You can add, remove, sort, and do more to lists through list methods.
Tuples are like lists but are defined by parentheses
()
instead of square brackets[]
and are immutable.
Now that you know what a list is and how to do common operations, we’ll move to another data type called dictionaries.