What Is a Dictionary?
Sometimes you might need to represent more complex data than numbers, strings, or lists.
A dictionary is a data structure that stores data in key-value pairs. An example of a key and a value is campaign_manager: "Spencer Smith"
where campaign_manager
is the key, and Spencer Smith
is the value.
Dictionaries are denoted by curly braces {}
at the beginning and end. Each key-value pair has a colon :
in between the key and value and a comma ,
at the end. Each dictionary has to have unique keys within it.
In the diagram below, the defined dictionary has three items, and each item is a key-value pair.
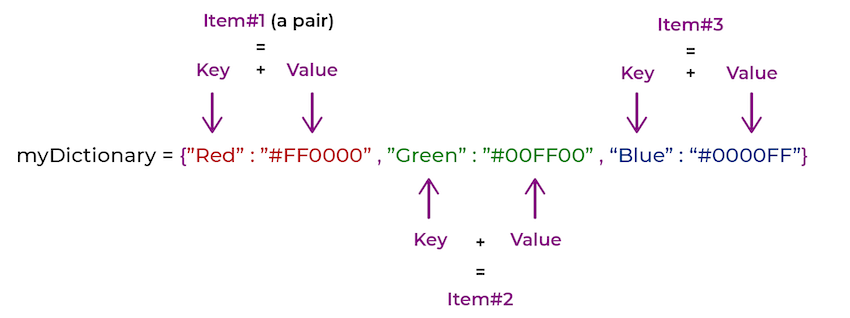
Create a Dictionary
Let’s say you want to store information about a new campaign for a dog food company. You’d probably need to save the campaign name, start and end dates, campaign manager, and the names of relevant influencers. You can save all of that information in one variable using a dictionary.
To store all the information, you could save a dictionary like so:
new_campaign = {
"campaign_manager": "Spencer Smith",
"campaign_name": "We love dogs campaign",
"start_date": "01/01/2020",
"end_date": "01/01/2021",
"relevant_influencers": ["@MyDogLover", "@DogFoodFavorites"]
}
You can also create a new dictionary with just empty curly brackets {}
or the dict()
function and add key-value pairs in after:
conversion_rates = {}
conversion_rates['facebook'] = 3.4
conversion_rates['instagram'] = 1.2
conversion_rates = dict()
conversion_rates['facebook'] = 3.4
conversion_rates['instagram'] = 1.2
Access a Value in a Dictionary
To access the different values, you can use the key for any one of the key-value pairs.
>> new_campaign['campaign_manager']
"Spencer Smith"
>> conversion_rates['facebook']
3.4
Give it a try in the activity below! 😁
Use Common Operations With Dictionaries
Just like lists, there are many methods (or operations) built into Python that makes it easy to interact with data in dictionaries.
Add a Key-Value Pair
To add a key-value pair to a dictionary, just add a new key to the existing dictionary. If the key already exists, setting a value will overwrite the existing key. The following code creates a dictionary called golden_doodle_facts
and saves information about the mass and origin of the Goldendoodle dog breed.
golden_doodle_facts = {
"mass": "30-35 lbs.",
"origin": "United States"
}
To add a new key-value about the scientific name of Goldendoodles, add:
golden_doodle_facts['scientific_name'] = "Canis lupus familiaris"
Now golden_doodle_facts
is:
golden_doodle_facts = {
"mass": "30-35 lbs.",
"origin": "United States",
"scientific_name": "Canis lupus familiaris"
}
If you were to write golden_doodle_facts[“mass”] = “100 lbs.”
, that would overwrite the existing value, so that:
>> golden_doodle_facts["mass"]
"100 lbs"
Delete a Key-Value Pair
To delete a key-value pair, you can use the del
keyword and the key you want to delete. To delete the “origin”
key-value pair from golden_doodle_facts
, type:
>> del golden_doodle_facts[“origin”]
>> print(golden_doodle_facts)
{ "mass": "30-35 lbs.",
"scientific_name": "Canis lupus familiaris"}
What is a keyword?
Certain words are part of the Python language and can’t be used when naming variables. Examples are del
, if
, and else
. Such words are known as reserved words or keywords.
Check for the Existence of a Specific Key
You can use the in
keyword to check whether a specific key exists in a dictionary. To do this, specify the key you want to search for, the keyword in
, and the name of the dictionary variable to search. The result will be a boolean indicating whether or not the key is in that dictionary. For example, if you want to search if the key “mass” exists in your golden_doodle_facts
dictionary, type the following:
>> "mass" in golden_doodle_facts
True
>> "breed" in golden_doodle_facts
False
Level-Up: Store Complex Data With Dictionaries
Context:
Now that you have become familiar with some operations, practice manipulating dictionaries using all the methods seen previously. 😁
Instructions:
Create a dictionary called
fruits
with keysapple
,banana
, andorange
, and valuesred
,yellow
, andorange
.Add the key
kiwi
with the valuegreen
to thefruits
dictionary.Access the value corresponding to the key
banana
and store it in a variable calledbanana_color
.Modify the value associated with the key
apple
togreen
.Remove the key
orange
from thefruits
dictionary.Display the remaining keys in the dictionary.
Once you have completed the exercise, you can run the following command in the VS code terminal pytest tests.py
.
Let’s Recap!
A dictionary is a way to store key-value pairs that represent a larger object.
You can create a dictionary using curly braces
{}
and put all the key-values in at first, or add them over time.Each key in a dictionary has to be unique.
Part 1 Summary
Congratulations! You've reached the end of Part 1 of this course and can now create data, the basic building blocks of Python code. You've accomplished a lot!
You've used variables to store information as data in Python code.
You've used data types to classify different kinds of data: integers, floats, strings, and booleans.
You've used lists and tuples to store related data.
You've used dictionaries to store complex data.
You’re well on your way to becoming a Python programmer! Now it’s time for a quiz to test your skills at creating data in Python. Then in Part 2, you'll learn to manage program logic.