You know how to print text on the screen. Great! This is a start, but you will be able to do much more after you have seen what variables are in programming.
Understand What a Variable is
Variables are one of the concepts found in all programming languages. You might as well say that without a variable, you can't program, and that's not an exaggeration.
As mentioned in the video, think of a variable as a kind of box containing a value. This box is itself stored on a shelf among many others, in a gigantic warehouse. The location of each box is very precisely recorded, just as your computer records the exact location of your variable in its memory.
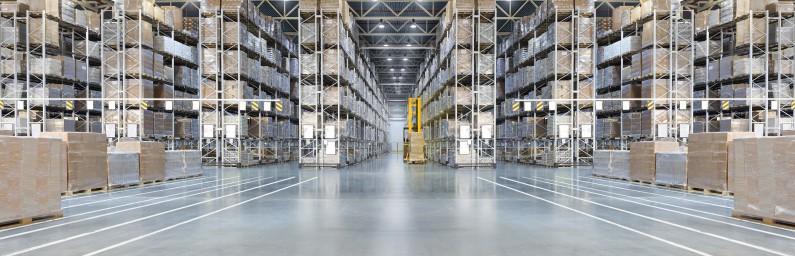
A value is what you will store in a variable. To return to the warehouse analogy, there are several boxes for storing different values. For example, if you work in a bank, you might want to store information about a customer in different boxes, such as their checking account balance and their savings account balance. We will also need to perform different operations on these boxes like emptying them, adding money, transferring the contents from one to another, etc. Variables will let you do this!
To get to the contents of each box, you will need to label them. The process is similar in programming: each variable is given a name.
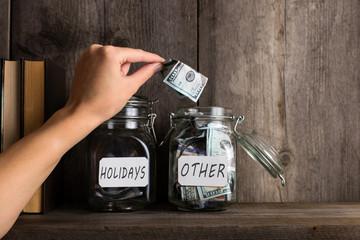
In the same way as with box labeling, the name of a variable must always represent its contents. Here are some general recommendations for choosing names for your variables:
Use clear variable names
It sounds tedious to do, but it is really beneficial for you and the people you will share your code with—it makes it easier to read and maintain your code. For example,savingsAccount
andcheckingAccount
are much more explicit names thanaccount1
andaccount2
.Use explicit variable names
Avoid abbreviations and acronyms, if possible, even if an abbreviation may seem obvious. For example,annualIncome
is better thanannualInc
.Follow a typographic convention
One of the most common typographic conventions is called camel case (also known as camel caps). It involves writing variable names containing several words without spaces or punctuation—the first word is written in lowercase, then each word is written with the first letter in upper case, as shown above.
Create a Variable
Before you can use it, you have to create your variable! You just have to associate a value with a name to create a variable; Python takes care of creating the right size ‘box’. Convenient, isn't it?
There are several types of variables in Python, much like in the physical world: text, numeric values, arrays, etc. Numeric variables are declared by associating a name with a numeric value. For example:
checkingAccount = 500
savingsAccount = 1000
You have declared two variables here, checkingAccount
and savingsAccount
, by storing the values 500 and 1,000, respectively.
Understand Operations Between Variables
As the name suggests, a variable can vary, or rather the value of a variable can change. You can do this through different operations. Considering the two variables previously declared in the last example, you could:
add some money to your savings account.
withdraw some from your checking account.
calculate how long it would take you to reach $5,000 if you save $500 every month.
calculate how much would be in your checking account if you added $30 every day for a week.
calculate how much would be in your checking account if you spent $10 every day.
etc.
In short, these are concrete problems that can be solved with Python. Each operation will use arithmetic operators:
+
: addition-
: subtraction*
: multiplication/
: division
The rules of arithmetic apply in Python, in particular the order of operations, but as in ordinary mathematics, you can use brackets to rearrange the order of the calculations. See how to do this in Python:
# add 100 to our savings (Yeah!)
savingsAccount = savingsAccount + 100
# remove 50 from our checkingaccount (Sniff)
checkingAccount = checkingAccount - 50
# calculate the number of days to save to reach 5000
numberDaysSave = (5000 - checkingAccount) / 500
# update our checkingaccount (again) after the daily gains/losses
checkingAccount = checkingAccount + (30 - 10) * 7
That makes a nice piece of code, doesn't it? If you pay attention, you will notice that there are different colors in different places. This is because your code is made up of comments and expressions:
Lines starting with a
#
are comments. They are used to document your code and help others understand it.The other lines of code (which can run operations, declarations, etc.) are expressions. They tell the computer what to do.
Here, each of the expressions assigns a value to a variable. The assignment operator is =
.
To the right of the assignment operator is the expression that will create/calculate a value.
To the left of the assignment operator, you write the name of the variable to which you will assign the corresponding value.
To summarize, to assign a value to a variable, you write an expression. This expression is built with the name of the variable, followed by the assignment operator = and finally the value to associate.
There are also other arithmetic operators that are a little more complex, but nevertheless useful, such as:
%
modulo : returns the remainder of the euclidean division**
power : raises a number to a certain power//
integer division: calculates the integer division (rounded down)
print(SavingsAccount % 500) # -> 100
# 1100 = 500 * 2 + 100, so 1100 % 500 = remainder = 100
print(9 ** 3) # -> 729, 9*9*9 = 729
print(SavingsAccount // 500) # -> 2
# 1100 = 500 * 2 + 100, so 1100 // 500 = integer division result = 2*
Try it for Yourself
Try to declare a variable from two other variables in the following exercise yourself.
You can find the solution right here.
Write Shorter Code with Simplified Operators
When you want to change a variable by changing the initial value via a basic operator, you can use a shorter version. In other words, you can use simplified operators! For example, rather than using the expression savingsAccount = savingsAccount + 100
to add $100 to your variable, you can use a kind of combined arithmetic/assignment operator +=
:
# long version
savingsAccount = savingsAccount + 100
# equivalent short version
savingsAccount += 100
Naturally, there is a version for each arithmetic operator seen previously:
-=
for subtraction*=
for multiplication/=
for division%=
for the remainder of the integer divisionetc.
Try it for Yourself
Try to use these simplified operators by yourself in the following exercise.
You can find the corrected version right here.
Go Beyond Arithmetic
In the example above, all variables are used to store numerical amounts of money (integer or decimal).
Are there other types of variables other than numeric ones?
Of course! In fact, it is possible to store any type of data in a variable.
So far, you have seen that a variable is made up of a name and a value that will automatically define its type. Yet, to return to your warehouse analogy, one can imagine that storing money, storing a book, or storing a car require different size boxes, or even containers. In Python, the storage space of a variable automatically adapts to its contents, almost by magic!
At this point, it is safe to assume that having only the ‘amount’ value stored in each of your customer's accounts will probably not be enough. We will most likely also need their name, their interest rate expressed as a percentage, their address, etc., with a new variable for each of these pieces of information. So we would need to store text in our variables.
You could declare these three variables as follows:
firstName = "Benjamin"
interestRate = 1.5
address = "15 19 Bloomsbury Way, Holborn, London, WC1A 2TH"
Note that you need to use double quotes (") to define strings—textual variables—in Python, otherwise you will get an error! You can however use single (') or double quotes to declare text variables as seen below:
address = '15 19 Bloomsbury Way, Holborn, London, WC1A 2TH'
# equivalent to
address = "15 19 Bloomsbury Way, Holborn, London, WC1A 2TH"
Let's Recap
In this chapter, you have learned the basics about variables:
A variable is made up of two parts: its name and its value.
Assigning a value to a variable is called an assignment.
The value of a variable can be changed.
The type of a variable depends on its value.
The names of your variables should be clear, explicit, and should follow a typographic convention.
In the next chapter, you will learn more about variable types!