Imagine that you have a block of code that you want to repeat several times in succession. You can of course store it in a function and call this function as many times as necessary. This would work, but it would be a bit like killing a fly with a bazooka: it works, but it is not necessarily the most optimal solution. Especially since, generally, we do not necessarily know in advance how many times we will need to repeat the said block of code.
Loops solve this problem! In programming, a loop is a structure that lets you repeat one or more statements, without having to rewrite them each time. There are two types of loops (for and while), which will be explained shortly.
Loop a Set Number of Times With the for Loop
For loops are used when you know in advance how many times an action will be repeated.
The for Loop on a Collection
The conventional use of loops in Python is to directly use the different values of a collection. Here is an example with a list:
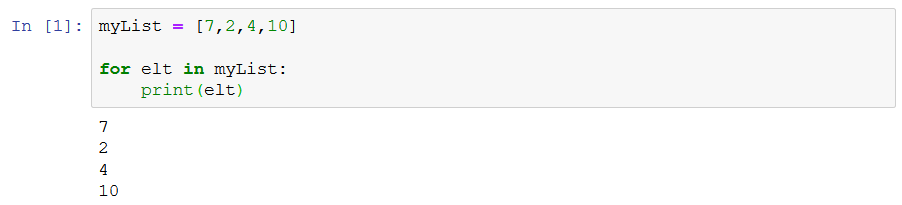
The printed result corresponds to each item in the list taken one by one. Let's take a detailed look at what has been achieved in the above code:
You have created a list:
myList
, containing four items: 7, 2, 4, and 10.The loop will store the first value of the list (in this case 7) in the
elt
variable.Then, the whole block of code associated with the for loop (defined via indentation... again!) is run with
elt
holding the first value. Here, this block just involves printingelt
.Once this is done,
elt
will take the second value of the list (in this case 2) and the block of code is re-run.The loop will continue until all the values in your list have been stored in the
elt
variable and the statements for the loop have been executed for each of them.
Here is a diagram to understand the Python logic behind the loop:
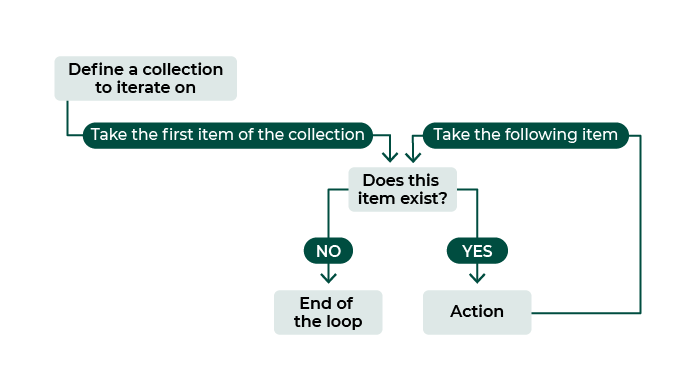
You can also iterate via a string! Remember that strings are also known as "character strings" because they represent a collection of characters, similar to a list.
myString = "Items"
for elt in myString:
print(elt)
In this case, elt
will successively take each character of your string.
The for Loop via an Iterative Integer Value
Quite often, you will find that you simply need to loop over a range of integer values, e.g. 0, 1, 2, 3…. This is the conventional loop you see most often in languages like Javascript or C++. Because Python wants to loop over a collection, you need to create a collection containing your range of integer values.
To do this, you will use the range(start, stop, step)
function, which will generate a collection of numbers according to three parameters:
start
: the first number of thesequence
.stop
corresponds to the last number of the sequence, non-inclusive. The function will generate numbers fromstart
tostop-1
.step
: the step between each generated number.
Not all parameters are necessary. For example:
for i in range(0, 5, 1):
for i in range(0, 5, 1):
print(i) # -> print from 0 to 4 by steps of 1 (end - 1)
for i in range(0, 5):
print(i) # -> prints from 0 to 4 also (default step is 1)
for i in range(5):
print(i) # -> prints from 0 to 4 also (default start is 0)
for i in range(0, 5, 2):
print(i) # -> print 0, 2 then 4
The for loop is perfectly suited when you have to perform an action a certain number of times known in advance or an action for each item of a collection. For all other cases, we can make a conditional loop: a loop that does not iterate through a collection, but according to a condition.
Loop According to a Condition with the while Loop:
The conditional loop is the while loop in Python.
As its name implies, the while loop will run as long as a condition is met. It is a kind of combination of a for loop and an if structure. The number of repetitions is not defined in advance, but via a condition to be fulfilled, as with an if. This is called a conditional loop.
The syntax is as follows:
while expressionLogic:
# block to execute
It can be interpreted as: as long as my logical expression is true, run the statement block.
Here's how it works:
The program checks that
expressionLogic
is equal toTrue
.If this is the case, the indented statements following the
:
are run. Once this is done, we return to step one.Otherwise, the program exits the loop without running the statements.
Try the example below:
numberTrees = 0
while numberTrees < 10:
numberTrees += 1
print("I planted", numberTrees, "trees")
print("I have a nice forest!")
This will produce the following result:
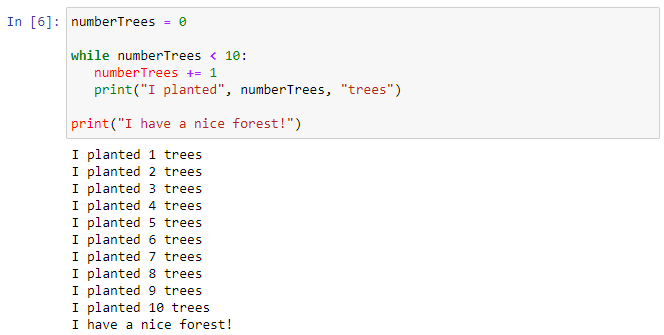
With each iteration, the numberTrees
is incremented by one. When the variable reaches the value 10, the expression numberTrees < 10
is no longer true! At this point, the loop ends and continues running the rest of the program in order. In this specific case, it prints: "I have a cool forest!"
Here is an example not to be reproduced at home (this one was made by a professional...):
theSunIsShining = True
while theSunIsShining:
print("Stay awake... forever!")
# the theSunIsShining never changes, so the condition is always true
# so we never reach this line
print("Time to go to sleep!")
This is a common mistake and unfortunately it can happen very easily. So be careful!
Try it for Yourself
Run a few loops yourself in the next exercise.
You can find the solution here.
Skip Some Statements Within Your Loop
Regardless of the type of loop, there will be situations where you will want to skip some of the iterations within your loop, or even terminate the loop prematurely.
For example, you want to repeat something 10 times, but skip (at least partially) when the value is 2 or 5. In Python, to force the start of the next loop iteration, use the keyword continue
:
for i in range(10):
# statements executed at each iteration
print(i)
if (i == 2) or (i == 5):
print("Special case")
continue
# statements not executed if i == 2 or 5
print("i != 2 & i != 5")
You can also decide to interrupt the loop, for example when looking for a particular item in a list. For this, you will use the break
keyword.
basket = ["apple", "orange", "banana"]
for fruit in basket:
if fruit == "orange":
print("I have an orange!")
break
Once the fruit has been found in your basket, you finish the loop.
Let's Recap
In this chapter, you have discovered two types of loops:
The loop for repeating an action a certain number of times, or according to a sequence: the for loop.
The loop that allows you to repeat an action as long as a condition is true: the while loop.
There is a common mistake that you must not make with the while loop: the infinite loop!
You can choose to skip certain loop iterations via the continue keyword.
The cycles of the loop can be interrupted via the break command.
Now that you have seen how to control your program flow, you can broaden your horizons with the discovery of modules and libraries!