In this chapter, we will discuss a common practice of software architecture and apply it to our TopQuiz application.
When you develop an Android application, you are free to apply any software architecture to your project. However, if you make a bizarre choice (or create your own), your code may be difficult for others to understand. If it's too tough to follow, your co-workers will stop talking to you.
That means you'll never know when there's cake in the office – ever. And on your end, you might have a hard time maintaining code written by someone else. So instead of eating delicious cake, you'll be crying tears of loneliness onto your keyboard.
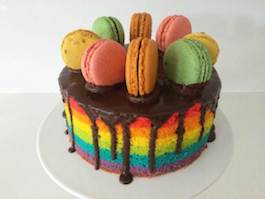
Most Android developers use a common architecture called MVC, or Model-View-Controller. This pattern is classic, and you will find it in the majority of development projects. It's not the only software pattern, but it's the one we'll study in this course and apply to our TopQuiz application.
MVC Pattern
The MVC pattern splits code into one of three MVC components. When you create a new class or file, you must know which component it belongs to:
Model: contains application data and business logic (the rules of the system). For example, user accounts, products you sell, a set of photos, etc. The model component has no knowledge of the interface. In TopQuiz, it will store questions and their answers.
View: contains everything that is visible on the screen and offers interaction to the user. In TopQuiz, this component is defined in the
activity_main.xml
andactivity_game.xml
files.Controller: This is the "glue" between the view and the model, which also manages the application logic. The controller reacts to the user's input and presents the data requested by the user. Where does it retrieve the data from? Yes, you guessed it: the model. In TopQuiz, we have two controllers: the MainActivity and GameActivity classes.
To make your code more modular and maintainable (your future colleagues will thank you, with cake), create 3 additional packages in Android Studio. Name them "Model," "View," and "Controller."
How do I create a subdirectory to organize my code?
Right-click the folder where you want to create a subdirectory and select New > Package. Name it something descriptive, like "controller." Move files to this new directory (e.g. Activity files), and validate by clicking the Refactor button. Android Studio will ask you to confirm your choice: click Do Refactor.
Application
When the user interacts with the application - for example, by answering a question - this is what happens:
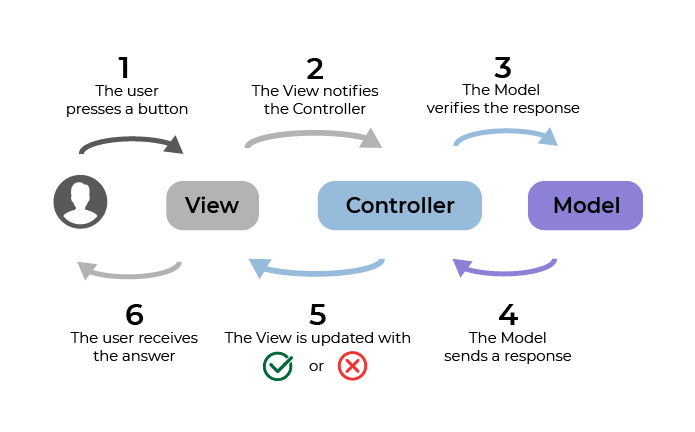
In step 3, the controller can update the model, if necessary - for example, if the user updates their application preferences.
Advantages
By applying the MVC pattern, you allow the majority of Android developers to readily understand your code. You also make it easier to reuse your code later. For example, you can export your application template as a library so that another developer can use it in their application or so that you can use it in your next app.
Disadvantages
You will have to create and maintain more files. I know, it's quite tempting to put everything in the same place, but this necessary evil is for everyone's benefit.
Let's Review With a Demo
You can see these steps in the video below:
Let's Recap!
MVC architecture is easy to use and integrate, so it works well for simple applications.
The Activity is the controller—it links up the view (the layout elements) and the business logic (the model).
In MVC, the model can be reused in other Activities to avoid code duplication.
So now you’ve discovered MVC architecture. In the next chapter, we’ll look at the model component of MVC, and how to define and implement it in our application.