What Is Broken Authentication?
Now we're onto the attack that has held the number two spot in security risks for web applications since 2013. Considering it held the third spot in 2010, OWASP demonstrates that authentication is frequently abused by malicious users.
So what is it and why is it such a popular attack?
Let’s say you have a site that requires you to log in with your username and password to access the pages that have information about your account. When you log in, the credentials are passed to the database. If the username and password match what is in the database, you will be authenticated for a session. A session is a specified amount of time that an authenticated user will have access to specific pages and activities on the application.
At any time in the authentication process, a malicious user can gain unauthorized access to the session. As attacks get more sophisticated, it is more important for web applications safeguard against them.
So what makes this kind of an attack so popular?
An unauthorized user can gain a lot of sensitive data when hijacking someone’s session.
So let’s go a little deeper into what these attacks look like.
Broken authentication can include automated attacks such as using a list in a brute force or credential stuffing attack. Brute force is done by trying as many username and password combinations as possible until getting the right one! When a malicious user has unauthorized access to a list of usernames and passwords, an attack called credential stuffing can be used to try all of the username and password combinations until this criminal authenticates.
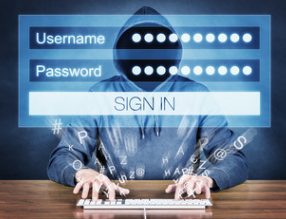
Why Can’t I Eat This Cookie?
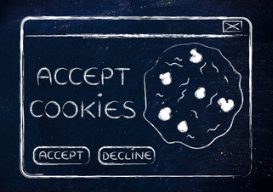
You may remember your browser warning you about a website requiring cookies for you to use it.
Well, I’m not sharing my delicious chocolate chip cookies with anyone so what is really going on here?
HTTP cannot save information on your browser, so a cookie is a saved third-party file that gives personalized access to the website user. Cookies were made to make your life easier, so you don't have to keep logging back in or entering things. They help a website remember what you have been doing on their site to help personalize the experience.
Tracking Cookies
Let’s say I was shopping for some gluten-free cookies on a website called koookiestore.com, and it downloaded a cookie onto my browser with an ID that identifies me. All of my activities on this site will be recorded on koookiestore.com via the cookie that holds my ID.
So, now I decided to go to funnikaaats.com, and suddenly I see all these ads showing me the EXACT gluten-free cookies I was looking at on koookiestore.com!
But only koookiestore.com can access the cookie information stored on its site, so how did funnikaaats.com find out that I like gluten-free snickerdoodles?
Here’s the trick! funnikaaats.com has embedded code for koookiestore.com inside its website so it can access your koookistore.com cookie. And now your obsession with funny cat videos is stored under that cookie ID in koookiestore.com.
This is how it looks:
Here are the koookiestore.com website and the cookie that connects you to both websites!
Now the koookiestore.com tracking cookie knows what cookies and cat videos you like.
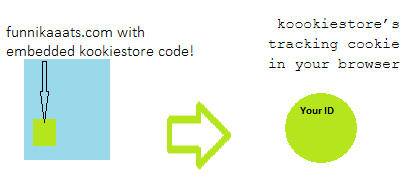
As you can imagine, it makes sense to call them tracking cookies. Let's talk about a different type of cookie that is used for broken authentication attacks.
Session Cookies
Session cookies hold an ID that identifies a session. Session management is important because it has caused some devastating data breaches.
Let's say you log into your bank's websites and are issued a session cookie that is stored in your browser and keeps you logged in until the cookie expires.
Each session gets a session ID, so authentication can also be hijacked if the malicious user guesses the right session ID.
Because browsers run on the HTTP protocol, websites do not have the ability to save authentication or session information unless they store them in a file on the browsers. That’s what session cookies are for.
Often times, the browser will save a cookie that contains the session information including the session ID, expiration date/time, and the authenticated path.
What on earth did all of that have to do with your web application?
You want keep hackers out of your cookie jar, so follow good coding practices! ;)
Protect Your Cookie Jar!
I want to introduce some best practices to keep your web applications safe from cookie theft. You see, someone can create or modify your cookie and try to create an unauthorized session!
Ensure cookies are encrypted in transmission via HTTPS.
Don’t store plaintext credentials in your cookie!
Set an expiration for your cookie/session.
What Can You Do to Prevent Malicious Authentication?
Sometimes you don’t realize how simple some solutions can be. One way you can start is by being proactive!
Require your users to have a strong password!
It is also best practice to require users to change their password regularly in case of credential stuffing.
Implementing an account lockout from too many failed attempts can prevent brute force attacks!
If your application has some default username/password combinations, ensure they are deleted from the database.
Require multi-factor authentication for users to log in.
Fortunately, there are frameworks to help implement secure code more easily regardless of the language you use:
ASP .NET Core Identity framework can be integrated into your web application to customize your authentication needs. Adding the ASP.NET Core IdentityServer allows you to use the secure coding techniques for token authentication including multi-factor authentication!
Ruby has gems like omniauth that can be implemented for authentication.
Java has javax.security.auth, and the Java Authentication and Authorization Service (JAAS) API, that can set up your authentication the right way!
PHP has PHPSec to manage security and sessions.
Protect Your Input!
Here is an example of sanitizing input before it is passed to the database
//Remove all characters from the email except letters, digits and !#$%&'*+-=?^_`{|}~@.[]
echo filter_var($dirtyAddress, FILTER_SANITIZE_EMAIL);
One good practice for input validation is to sanitize all input by removing irrelevant characters. This also increases your chance of preventing injection attacks.
Remember when we talked about limiting characters like the equal sign and single quotes?
In Java, you can create a method to hash the password on input in the Spring framework.
First, you will create an object for the encryption algorithm, and salt it.
MessageDigest md = MessageDigest.getInstance("MD5");
md.update(salt);
Then you will input your password using the salted object to assign the formatted hash to hashedPassword
.
byte[] hashedPassword = md.digest(passwordToHash.getBytes(StandardCharsets.UTF_8));
No matter what language you code in, there are several solutions available that can allow you to add security to your authentication mechanism!
Best practices for session management and tokens are also important because it is another form of authentication. Session cookies and tokens can make your sessions vulnerable to hijacking.
Session IDs can be guessed, applied in the URL for instant authentication, and exposed due to default naming conventions based on its framework.
Here are a few pointers to get you started when coding sessions:
Don’t put your session ID in your URL.
Give your session ID a 64-bit entropy.
Limit the length of the session ID.
Hard code restrictions on the session.
Change the default session ID name.
PHP has a library called the SessionManager with functions that can be used to validate sessions with restrictions.
Here is an example of the preventHijacking()
function in SessionManager used to limit a session to a specific host and IP address. If the host and IP address are not the same, it will not authenticate.
static protected function preventHijacking()
{
if(!isset($_SESSION['IPaddress']) || !isset($_SESSION['userAgent']))
return false;
if ($_SESSION['IPaddress'] != $_SERVER['REMOTE_ADDR'])
return false;
if( $_SESSION['userAgent'] != $_SERVER['HTTP_USER_AGENT'])
return false;
return true;
}
Know Your Level of Protection
If I implement all these rules, will my web application be safe?
No matter how many security layers and features you add to your code, there are always attacks you won't be ready for.
Stay apprised of new vulnerabilities so you can secure your application for future attacks.
So... can I completely prevent broken authentication with these techniques?
Long story short... Nope.
Your application is never 100% safe.
However, you can prevent common attacks, and reduce your attack surface by 80%. Those aren't bad odds.
We’ve looked at securing code from injection and broken authentication, but we aren’t done yet! Let’s look at how you can protect your sensitive data from exposure.
Let’s Recap!
Broken authentication may happen when a web app isn’t coded to secure cookies.
Use input validation and limit login tries.
Brute force and credential stuffing tools are common.
Keep session IDs secure by encrypting them.
Limit session times.