A place for everything and everything in its place
Ever get super busy, and when you finally have a moment to catch your breath, you look around your house and realize things have gotten a bit messy?
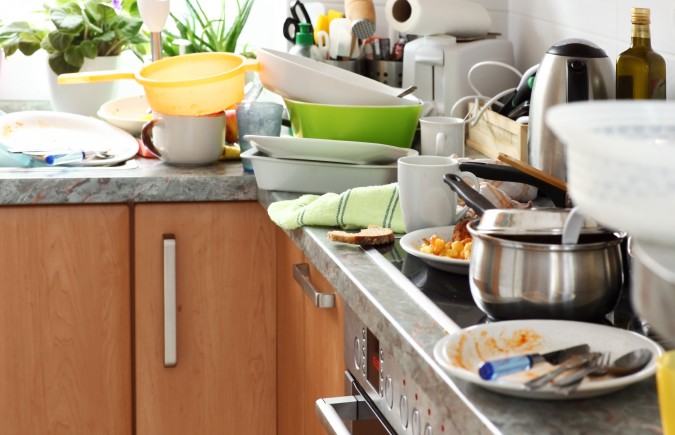
In other words, it’s time to do some housekeeping. It’s not everyone’s favorite thing to do, but taking a step back and tidying up is vital to keeping a clean and healthy codebase.
So far, you've learned a lot, and you've created new code along the way. Your Saas files are organized with grouped variables and components written in nice, neat BEM blocks. But you also have mixins, functions, and different blocks of code to scroll through whenever you need to find anything.
Luckily, Sass allows you to split code up, so you can categorize and organize it by moving it into individual files. You can put all of your variables into a single file. And all of your mixins in another. The same goes for layouts and components. Being able to open a specific file to easily locate a code block is going to save you time and headaches.
The Seven Dwarfs: the 7-1 file system
To organize all of these new files, you’re going to use what is known as the 7-1 file system. The "7" refers to seven thematic directories (which is programmer-speak for “folder”) you put your files in, which funnel into the “1”: a single .scss
file compiled into your site’s CSS style sheet.
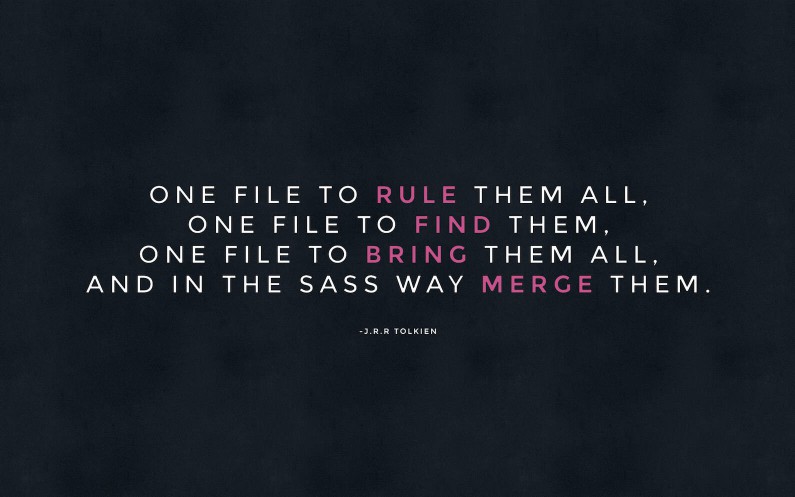
Let’s go ahead and create the following seven directories within our sass
directory, each of which represents a category of Sass code:
Base
Utils
Layout
Components
Pages
Themes
Vendors
A quick way of creating directories is to use the terminal within VS Code and the mkdir
command followed by the name of the directory that you want to create. You can create multiple directories at once by listing them out:
mkdir base utils layout components pages themes vendors
Now that you've created seven unique directories to store your code, let's break down what each is for:
The
base/
directory contains the files that define the foundation of your site, such as the typography and norms you want applied site-wide, likebox-sizing
.utils/
is where you store variables, functions, mixins, and%placeholders
for extensions (if you use them).layouts/
is where you store BEM blocks which contain things that can be reused, such as a form or header for large layouts.components/
is where you store BEM blocks that are more self-contained, such as buttons.
pages/
contains blocks of code that only apply to a single page. While you use buttons all over the site, your home page has a quote section and a project grid that isn’t used anywhere else. In other words,pages/
are rules specific to a single page and won't be reused elsewhere.themes/
is where you store thematic code, such as custom styling for Christmas or Halloween. This doesn't apply to the site we're creating.vendors/
is a directory for third-party library style sheets such as Bootstrap or jquery UI. It's essentially for any CSS that has originated from outside of the project. Using third party frameworks, such as Bootstrap, are common as they speed up site development with predefined style sheets for things like buttons and forms.
Cleaning house
Now that you have all of these directories, what do you do with them? Let’s begin cleaning things up by moving all of the variables into their own file. In the utils
folder, make a file named _variables.scss
:
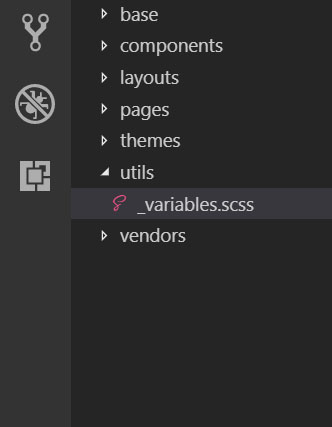
Now you have a file for your variables nested in the utils/
directory. The name makes sense; a file named variables to store variables. But… what’s up with that underscore?
When you split code up, the individual files are all part of a larger codebase, so Sass calls them partials. To tell Sass that a file is a partial, you need to prefix it with an underscore ( _ ).
So, we have a file for our variables, now let’s cut them from our main.scss
file and paste them into our new variables file:
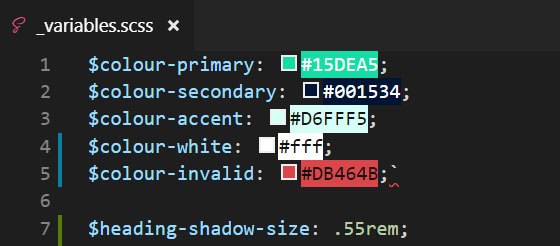
And when you look at the compiled CSS, you'll see an error!
error sass/main.scss (Line 16: Undefined variable: "$colour-secondary".)
Sass is saying that we’re using undefined variables in the code; we’ve split our variables into their own partial, but haven’t told the main.scss
file where to find them! Before you can use code that’s been split into a partial, you need to import it back into the main codebase by using the@import
keyword.
@import "./utils/variables";
To import variables, you need to type @import
followed by a set of quotes. Inside of the quotes, type out the path to the variables partial. The leading period (.
) of the path tells Sass that the partial’s path starts in the same directory asmain.scss
. Then/utils/
leads Sass to the utils directory, where it will find the variables partial.
And if you check out the compiled CSS, you see that the variables are working as if they were still in the original file:
body {
box-sizing: border-box;
background: #001534;
}
Perfect!👌
Cleaning your form block
Now let’s do the same for the form block! Inside layouts/
, make a new partial named _form.scss
, then cut and paste the form block frommain.scss
. We’re placing it in the layout directory, because it’s a larger component that is largely defined by its layout rules:
.form {
width: 100%;
padding-bottom: $grid-gutter;
&__heading {
width: 100%;
color: $colour-white;
@include heading-shadow;
background: $colour-primary;
line-height: 5rem;
padding: $grid-gutter;
}
&__field {
& label {
color: $colour-accent;
display: block;
font-size: map-get($txt-size, label);
line-height: map-get($txt-size, label);
padding-top: $grid-gutter;
}
& input {
width: 100%;
background: $colour-secondary;
@include border;
padding: $grid-gutter;
color: $colour-accent;
}
& textarea {
width: 100%;
color: $colour-primary;
background: $colour-secondary;
@include border;
outline: none;
padding: $grid-gutter;
margin-bottom: $grid-gutter*0.5;
&:active, &:focus {
color: $colour-accent;
}
}
}
}
With the form block in its own partial, we must now import it into our main file to make use of it:
@import "./utils/variables";
@import "./layouts/form";
Note that we have placed the form import after the variables. The order that you import partials into the main file is the order that Sass will compile all of its contents. If we imported the form partial before the variables, we’d get a compile error, because the variables used inside of the form partial haven’t been defined yet!
As a rule, you want to import your files in the following order directory/partial order:
Utils:
Variables
Functions
Mixins
Placeholders
Vendor sheets (if you have them)
Base
Components
Layout
Pages
Themes
Once all of the code has been split into proper partials and imported, themain.scss
file should only contain imports; rulesets will be contained in their appropriate partials:
@import "./utils/variables";
@import "./utils/functions";
@import "./utils/mixins";
@import "./utils/extensions";
@import "./base/base";
@import "./base/typography";
@import "./components/buttons";
@import "./layouts/header";
@import "./layouts/nav";
@import "./layouts/container";
@import "./layouts/form";
@import "./pages/work";
@import "./pages/about";
@import "./pages/project";
Now finding a chunk of code is as simple as glancing at your main.scss
file and finding where it’s located! Easy to read, write, and maintain. That’s the coding trifecta right there!
Next, we’ll get Sass up and running on your own computer!
Let's recap!
The 7-1 file system has seven thematic directories which funnel back into one main .scss file.
The 7 thematic directories are:
Base
Utils
Layout
Components
Pages
Themes
Vendors
In Sass, individual files are called partials. These start with an underscore.
Import partials back into the main
.scss
file by using the@import
keyword.In Sass, partials are compiled in the same order they are imported.