Creating a hierarchy in your code
Wouldn’t it be awesome if you could write your CSS like you write your HTML? Rather than a long list of CSS selectors, you could indent your elements and modifiers within their parent block, like this:
.nav {
padding-right: 6rem;
flex: 2 1 auto;
text-align: right;
.nav__link {
display: inline;
font-size: 3rem;
padding-left: 1.5rem;
.nav__link--active {
color: #001534;
}
}
}
Having a visual hierarchy not only makes things much easier to read, but it also helps to keep everything contained. By forcing an object to exist within relative blocks, it makes it much easier read and maintain your codebase; rather than scrolling around a document for random elements from a block of code, you simply need to locate that particular block and all of its relative elements will be there with it.
Meet your new best friend: CSS preprocessors! With preprocessors, you can write your code in a more visually coherent manner through features like nesting. Nesting means you place selectors within one another, creating a hierarchy, just like in HTML! Check out some styling written using nesting within a preprocessor, which is on the left, and the resulting CSS on the right:
|
|
Don’t concern yourself too much with the syntax just yet, as we’ll be getting to that shortly. 😇 Instead, notice how the nested code produces a very standard looking CSS file. While you want the benefit of coding in a more convenient syntax, web browsers still require your styling to be provided in a normal old CSS file. Preprocessors give you the best of both worlds by compiling its syntax into standard CSS for browsers to read and render.
In addition to a much nicer syntax, you also have access to functionality that you’d find in other common programming languages, such as variables:
|
|
Variables allow you to store often repeated values, such as colors and measurements, into a single element and reuse it throughout your code. Imagine that you’ve used a shade of green hundreds of times throughout a site, and now need to change it to red. With variables, you’d only have to change it once, and it would update everywhere the variable has been used. One change is easier and cleaner than hundreds of them!
Loops, which automate repetitive tasks such as creating a bunch of color modifiers, save you a lot of tedious coding while maintaining a much smaller codebase to manage:
|
|
Logical operations allow you to write a single block of code that you can use in different circumstances and have it react accordingly, such as determining the font color based on a background color. For example, if the background color is dark blue, make the font white. Over time, this means a smaller, more concise codebase, which, of course, is easier to maintain.
|
|
Meet the players
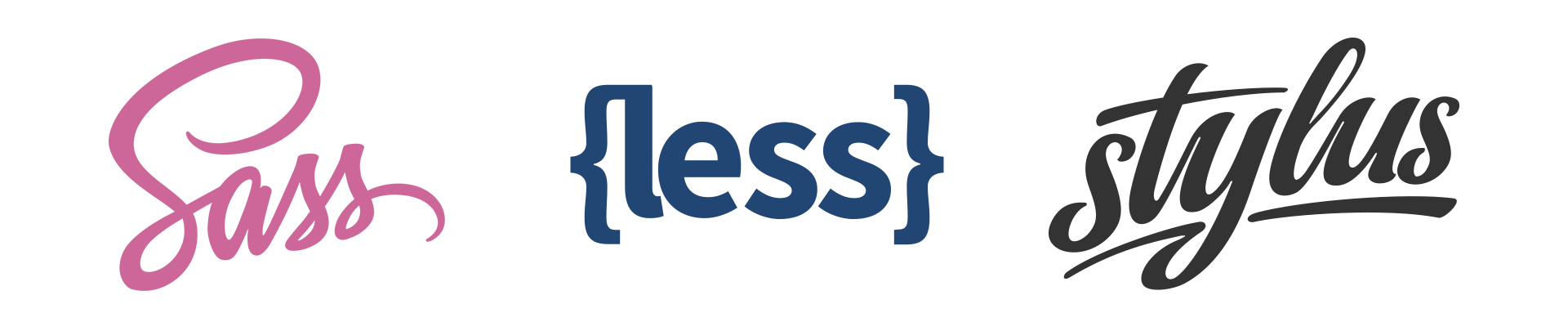
There are a lot of CSS preprocessors out there, but the three most prominent are Sass, Less, and Stylus. And, truly, there’s very little that separates them. There are slight differences in syntax and functionality, however, on the whole, they all do the same thing in a very similar manner.
In this course, we’ll be using Sass, but, more importantly, you’ll be learning the concepts and processes of using a CSS preprocessor, which can be applied to Less and Stylus as well.
So, why Sass? As I said earlier, they are all really similar tool sets. However, you are far more likely to run into Sass in a professional environment, and it only makes sense to learn with the tool that you are most likely use in your day to day life.
Coming up next, we’ll dive into Sass by learning its syntax and the tools you’ll use to write it.
Let's recap!
CSS preprocessors allow you to nest your code, creating a more easily read hierarchy and allowing you to bundle units of code together.
There’s a whole bunch of preprocessors out there, but the most common is Sass, which stands for Syntactically Awesome Style Sheets.
Beyond nesting, preprocessors open the door to using programmatic functionality to create a more maintainable codebase while reducing the amount of tedious code you need to write.