Three kinds of error
In JavaScript, as with many other programming languages, you generally differentiate between three types of error.
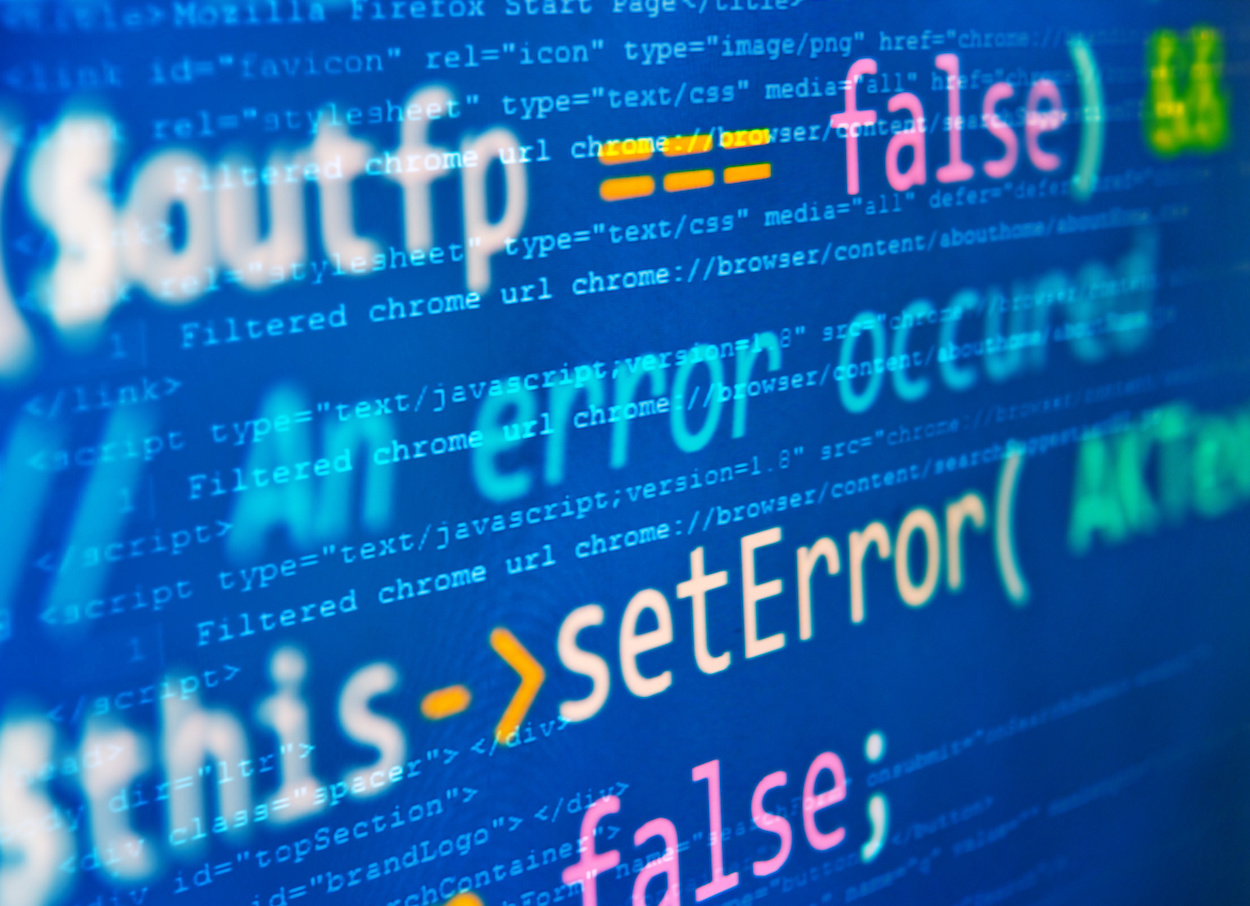
Syntax errors
Syntax errors (or parsing errors) happen when you have miswritten something in your code. It could be a forgotten bracket or curly brace (or one too many!), or a misspelled else
or switch
, for example. These are generally relatively easy to fix (although you may spend a long time chasing the odd missing parentheses!), and many text editors or IDEs automatically highlight syntax errors.
Here are a few examples of syntax errors — can you spot them?:
if (seatsRemaining) {
passengersBoarded+;
}
while (seatsRemaining > 0 {
passengersBoarded++;
}
if (seatsRemaining > 0) {
passengersBoarded++;
} else {
passengersStillToBoard = 0;
Logic errors
Logic errors are a bit more vicious. They arise when you have mistakes in your program logic. Here are a few examples:
Assigning the wrong value to a variable.
Mixing up conditions in
if
statements.Writing lines or blocks of code in the wrong order.
The best-case scenario with logic errors is that your program has some unexpected behavior; the worst case is that it crashes completely! Logic errors are also harder to find and fix because the code isn't wrong, it simply doesn't do what you want it to!
Runtime errors
Runtime errors are a bit different. They tend to arise when something unexpected happens in your app, often related to outside resources (network connections, physical devices, etc.) or human input/error. However, there are situations where you know, in advance, where this kind of error is likely to occur. In these situations, you can implement code for error handling — this way, an error will not crash your program, and can be corrected for.
One way of handling potential errors is to use an if
/else
statement to check for valid data:
if (dataExists && dataIsValid) {
// use data here
} else {
// handle error here
}
You can also use try
/catch
blocks to try code that might potentially throw an error, and catch any error that occurs:
try {
// error-prone code here
} catch (error) {
// react to error here
}
As you gain experience in JavaScript development, you will learn where errors can occur, and how to prepare for and handle them properly.
Let's recap!
In this chapter, you learned the three different types of programming error:
Syntax errors — errors in the usage of JavaScript: typos, missing brackets, wrong number of quotes, etc.
Logic errors — mistakes in the application of program flow, like erroneous conditions in
if
statements or forgetting to increment the index in a loop, potentially leading to an infinite loop.Runtime errors — errors which are often caused by outside resources like networks, databases, or users; they can be handled by being aware of where they may occur, and using error handling techniques like
try
/catch
blocks.