What's the purpose of integration testing?
Testing your .NET applications manually to ensure the system works as expected from end-to-end is a difficult, time-consuming task for a single person to accomplish.
Even unit testing, which can be automated, falls short of your complete testing needs. As you've seen in previous chapters, unit tests are generally intended for testing specific components in isolated test environments.
But software doesn't tend to operate in isolated environments. Most software needs to interact with third-party components, such as web servers, databases, and others.
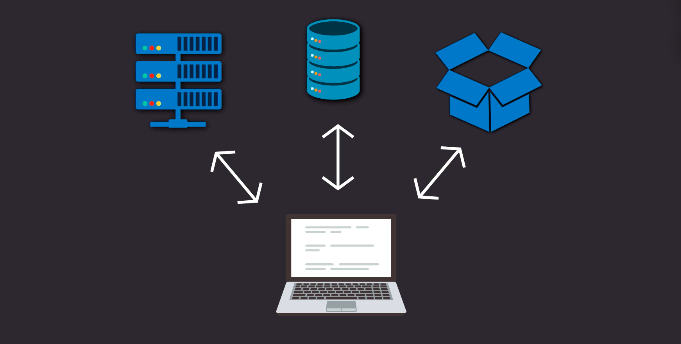
The next step in covering your code is to use grouped tests to ensure that your unit tests are working at a higher level of integration.
That's where integration tests come into play.
An integration test evaluates the individual test interactions under a delimited test context with its own set of test cases. Verifying that the different parts of the application work correctly when grouped together allows you to automate the way you test your application on a broader scale.
Set up an integration test project
Because of the nature of the components involved, integration tests might take more time to develop, maintain, and execute than regular unit tests. The earlier they are configured and made available to the team, the better.
Generally, for ASP.NET Core for Web API-oriented applications, your system can interact with the real ledgers in your system. Testing on this level involves ensuring your API will work end-to-end in the hands of your clients (as a desktop or mobile app or as another integrated component) and will send data across the network through HTTP-based requests, parameters, content-types, headers, and security considerations.
Integration tests in ASP.NET Core are implemented with the following:
A test project that contains and executes a particular set of integration tests over a specific set of components to be covered, including database, web requests, file-system, and some others depending on the stack used.
The test project contains references that allow you to run the component under testing as a test web host, with an actual client to handle requests and responses.
Both of them can be run by using a test to provide test results (successful or failed).
To test your ASP.NET applications, you can take advantage of the benefits of .NET Core. By wrapping your server and Web API as part of a web host that can be executed inside a test context and called from test suites for integration testing, your testing environment can help you to test and double check it works as expected.
Create a simple ASP.NET Core Web API
Let's use a very simple Web API in .NET Core for our CalculatorProgram. This means running our program on a server that can communicate with the internet, allowing broad use between web, mobile, and other technologies.
By using ASP.NET Core, we can use a controller class that becomes responsible for dealing with the HTTP calls hitting our web server, so they can interact with our CalculatorProgram (which works as a model) and return a data representation, commonly a JSON result (aka, a view). Having a controller connected to a model and returning a view representation is commonly known as the MVC Pattern (model-view-controller), which is broadly used in ASP.NET Core.
For our CalculatorProgram, having a very simple ASP.NET Core project will allow us to serve data from our web server and perform the calculator operations defined in the calculator core logic. So we'll have a web server in charge of running calculator logic through the web, as follows:
using CalculatorProgram;
using Microsoft.AspNetCore.Mvc;
namespace WebCalculator.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class CalculatorController : ControllerBase
{
[HttpGet, Route(nameof(Sum))]
public decimal Sum(decimal x, decimal y)
{
return new Calculator().Sum(x, y);
}
}
}
Use xUnit for integration testing
Testing against controllers and MVC in ASP.NET helps keep your code organized and following a highly testable pattern. In the context of integration tests, controllers in your codebase can be instantiated, called, and consumed in different manners depending on their return values and content-specific types.
The next level of testing that you can have in your Web API is to validate the correctness of the data being sent back and forth between server and client (a client being a web or mobile application, or even another server).
To achieve that, ASP.NET Core provides a test host.
A test host is a set of test-oriented architecture and specific components that allow you to interact with your Web API and server in a test-driven way and with production-oriented behavior. A test host can be added to integration test projects and used to host ASP.NET Core applications, serve requests, and execute as a real web host. By separating the unit tests from the integration tests in different projects, you can ensure that components in your infrastructure can be tested granularly. This gives you more control over what is actually tested.
Add the TestHost package to your integration test project
Let's create an integration test project as part of our solution. Let's create a new class library called "IntegrationTests." It will have its own folder and project structure so it can run separately from other solution items and test projects.
Now that our integration test project is configured, we can start by creating a reference to our WebCalculator and set up the "test context" for the components under testing. This test context is based on the host test framework called "TestHost" of the Microsoft.AspNetCore
namespace (Microsoft.AspNetCore.TestHost
), which can be added by editing the IntegrationTests.csproj file in VisualStudio. Also, we will add the xUnit, the xUnit Runner (xunit.runner.visualstudio
), and Microsoft.NET.Test.Sdk
to the project as NuGet packages.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netcoreapp2.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.NET.Test.Sdk" Version="15.3.0-preview-20170628-02" />
<PackageReference Include="Microsoft.AspnetCore.Mvc" Version="2.0.2" />
<PackageReference Include="Microsoft.AspNetCore.Diagnostics" Version="2.0.0.0" />
<PackageReference Include="xunit" Version="2.2.0" />
<PackageReference Include="xunit.runner.visualstudio" Version="2.2.0" />
<PackageReference Include="Microsoft.AspNetCore.TestHost" Version="2.0.2" />
<ProjectReference Include="..\WebCalculator\WebCalculator.csproj" />
</ItemGroup>
</Project>
After you've installed the Microsoft.AspNetCore.TestHost package in the test project, you can create and configure a TestServer to use in your integration tests. You can also automate the process of checking/calling the Web API to examine content and perform HTTP calls later in this course.
For the purpose of enabling and developing our TestHost, we are ready to write our first integration test!
using System.Net.Http;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.TestHost;
using WebCalculator;
namespace IntegrationTests
{
public class TestContext
{
private TestServer _server;
public HttpClient Client { get; private set; }
public TestContext()
{
SetUpClient();
}
private void SetUpClient()
{
_server = new TestServer(new WebHostBuilder()
.UseStartup<Startup>());
Client = _server.CreateClient();
}
}
}
Let's recap!
While unit testing is intended for testing isolated components, integration testing allows you to test grouped components and their true interactions, taking into account web servers, HTTP calls, databases, and other elements of your system.
You can take advantage of ASP.NET Core tooling to perform integration testing in Visual Studio, NuGet, and existing testing frameworks to have specialized and dedicated test suites for your integration testing needs.
You can take advantage of .NET TestHost in your integration tests. By using a server test host, you can transparently test your components as part of an integration test based on xUnit without having to deploy your Web API to a server and immediately get results from test suites, facts, and assertions for building robust integration testing code.