What Is MVC?
You've just discovered the SOLID principles, but before we dive into the details, you need to know how to organize your code to make these principles easy to work with. Luckily, there is MVC!
MVC is a software architecture approach. It divides the responsibilities of the system into three distinct parts:
Model: holds the state information of the system.
View: presents the model information to the user.
Controller: makes sure that user commands are executed correctly, modifying the appropriate model objects, and updating the view objects.
Why Is Using MVC Architecture a Good Practice?
Before Microsoft introduced ASP.NET MVC in 2009, developers used web forms to build .NET applications. These web forms were based on code-behind pages that contained functions for each event on the page called server controls.
In simple terms, you had a web page for the user interface (UI) and a class to store the activity needed for that page. The goal was to separate UI from code instead of having it all together on a page.
It used ViewState to maintain state across requests, often resulting in large blocks of data transferred between the client and the server. If you looked at the HTML code in the web page, you would see this block of encrypted code at the top of the page, which is the ViewState.
If not used correctly, these blocks could reach hundreds of KB and frustrate visitors waiting for a page to render. Other issues included:
The page lifecycle was very complicated and caused ViewState errors if not used correctly.
Because of the server controls, developers also had a difficult time modifying HTML in the server controls. Also, Microsoft’s HTML and CSS didn’t follow web standards.
Even though the code-behind gave the impression of separation of UI and code, it never really was a complete separation because you still had to mix presentation code with business logic.
Lastly, web forms came out before automated testing took off. It’s nearly impossible to create any automated testing of web forms.
Once Microsoft adopted the MVC pattern, they then created ASP.NET MVC. The MVC pattern had been around for years in desktop software development, and for Web development, the only language around at the time that implemented MVC was Ruby on Rails.
When ASP.NET MVC was released, it addressed many of the problems found in web forms with the following advantages:
Finally! Separation of concerns using the single responsibility principle (SRP). You will learn more about this principle in later chapters. It is probably the best part of MVC because it keeps your code neat, tidy, modular, easy to read, and maintainable.
HTML & CSS control: No more server controls. You use HTML and CSS any way you want.
Unit testing: It was easier to create unit tests to check your code because you no longer had ViewState and other request/response objects that hindered writing tests.
BetterSearchEngineOptimization: Web form URLs had the .aspx extension where MVC URLs do not have any; for example: http://localhost/Customer/List. By having custom URLs, search engines recognize your application better.
No ViewState. With no ViewState, pages rendered much faster, thus giving a much better application performance.
Like I stated in #1 above, the separation of concerns in the MVC pattern is the best feature. In the diagram below, you can see how the model, view, and controller communicate with each other:
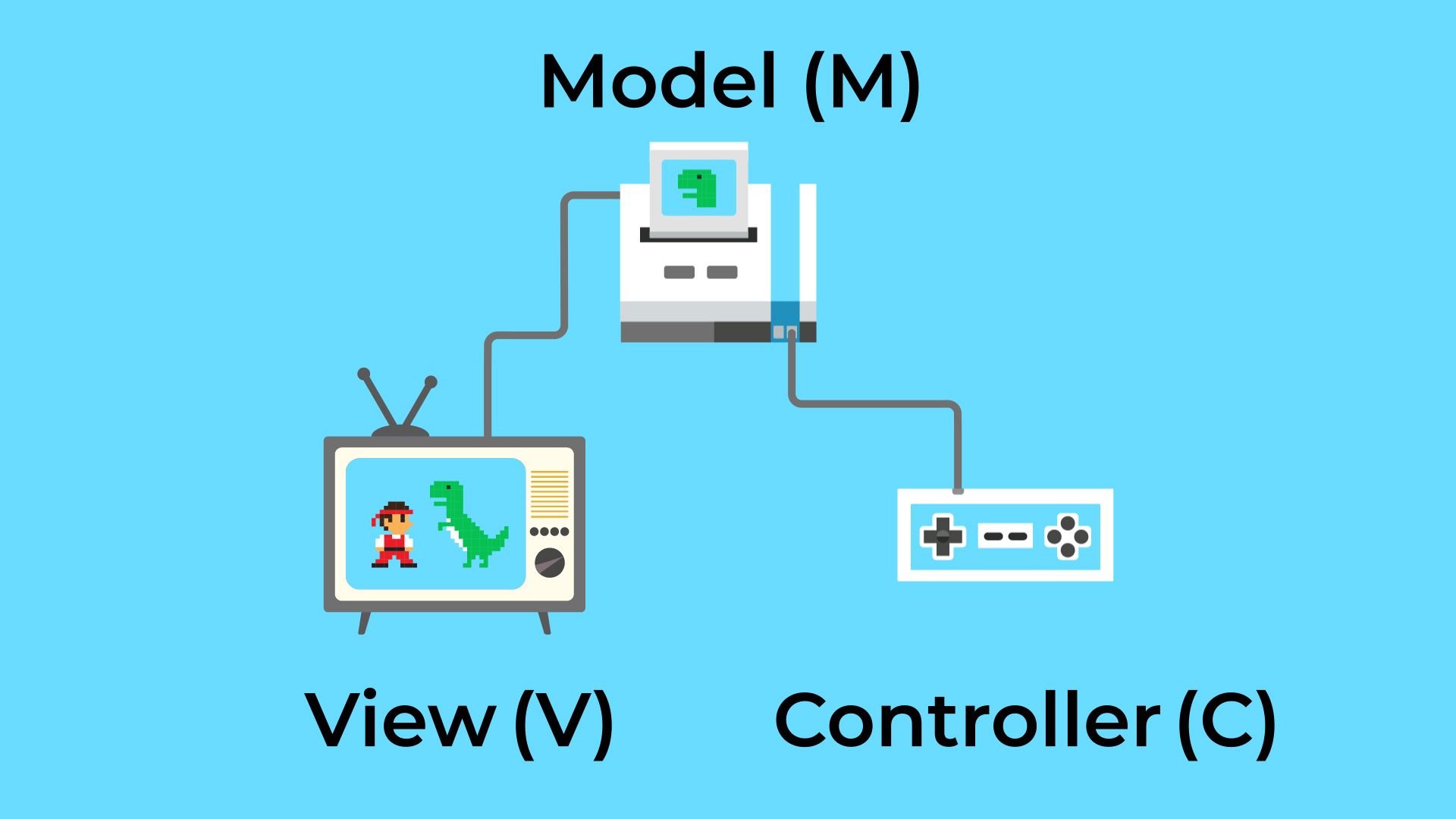
What Goes in the Model (M)?
The simplest explanation of the model is that it’s the container that transfers information between the view and the controller. Because the model doesn’t depend on either the view or controller, it can be tested independently.
The model shouldn’t contain any business logic, but only handle validation and formatting rules for its public properties.
Here’s a C# example of a model:
namespace MVCExample.Models
{
public class StudentViewModel
{
public int StudentId { get; set; }
public string StudentName { get; set; }
public int Age { get; set; }
}
}
What Goes in the View (V)?
The view is what the visitor sees; the user interface (UI). The view takes the model that was passed and displays it to the visitor. In turn, a visitor can modify the model like in a form and return it to the controller.
ASP.NET MVC uses a view engine called Razor, where you can mix HTML tags and server-side code. Razor uses the @ character instead of the <% %> found in web forms. These pages are saved with the file extension .cshtml for C# and .vbhtml for Visual Basic pages.
Here’s an example of an Index.cshtml view page:
@model List<StudentViewModel>
<h2>Index</h2>
<p>
@Html.ActionLink("Create New", "Create")
</p>
<table class="table">
<tr>
<th>
@Html.DisplayName("Name")
</th>
<th>
@Html.DisplayName("Age")
</th>
<th>
</th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(m => item.StudentName)
</td>
<td>
@Html.DisplayFor(m => item.Age)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.StudentId }) | @Html.ActionLink("Details", "Details", new { id=item.StudentId }) | @Html.ActionLink("Delete", "Delete", new { id = item.StudentId })
</td>
</tr>
}
</table>
The image below displays how the view renders to the visitor:
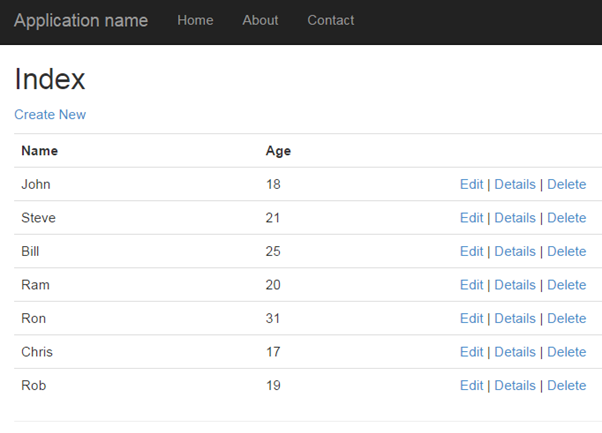
What Goes in the Controller (C)?
The controller is where all the real work happens. It handles the incoming URL request using action methods to create the model, retrieve data, populate the model, and return it to the view.
In ASP.NET MVC, every controller class name must end with the word “controller" and inherit from the Controller
class. For example, the controller for the student page must be StudentController.
Also, if you return just View(model)
, the name of the .cshtml page must be the exact name as the method you are returning from. In this instance, Index
must return to Index.cshtml.
Here’s an example of a C# controller:
using Microsoft.AspNetCore.Mvc;
using MVCExample.Models;
namespace MVCExample.Controllers
{
public class StudentController : Controller
{
// GET: Student List
public IActionResult Index()
{
//populate a model with a list of all students from a Service class
List<StudentViewModel> model = Service.GetAllStudents();
//return the model with the view
return View(model);
}
}
}
Let’s Recap!
You gain better code separation with the MVC architecture.
The model is the data passed between the controller and view.
The view is the page shown to the user in their browser.
The controller handles a request to retrieve data and populate the model.
Before we move on, let's check your understanding of MVC with a quick quiz.
In the next part of the course, we'll dive deeper into the SOLID design principles and begin applying them one by one.