What is External Account Authentication?
Often, you go to a site and see an option to log in with a social media account. This type of login is known as external account authentication. It allows the application to retrieve your information from another verified account and use that data to set up your new user account on that site. This section explores how to go about using external account authentication to register and authenticate users in a .NET Core web application.
The process to set up and permit this type of authentication typically consists of three primary parts:
Create an app in the development space of the third-party login provider.
Use the
SecretManager
to store tokens assigned by the login providers.Configure the login providers for your application.
While the process is essentially the same, every provider is different, and each requires its own special way of setting up the authentication. We will explore just one provider’s process in this chapter. However, to learn about the processes defined for other common providers, please visit the following links:
Add Facebook Account Authentication
Let’s walk through the process of setting up Facebook account authentication for users in an MVC application. This requires you to complete the three steps described in the previous section, as they apply to Facebook, specifically:
Create a Facebook app.
Store the Facebook App ID and App Secret.
Configure the Facebook authentication.
Create the Facebook App
To create Facebook apps, simply navigate to https://developers.facebook.com/. If you are not already logged into Facebook, you’ll need to do so in the developer space. Once logged in, the right-most menu option will read My Apps, as shown in the figure below.
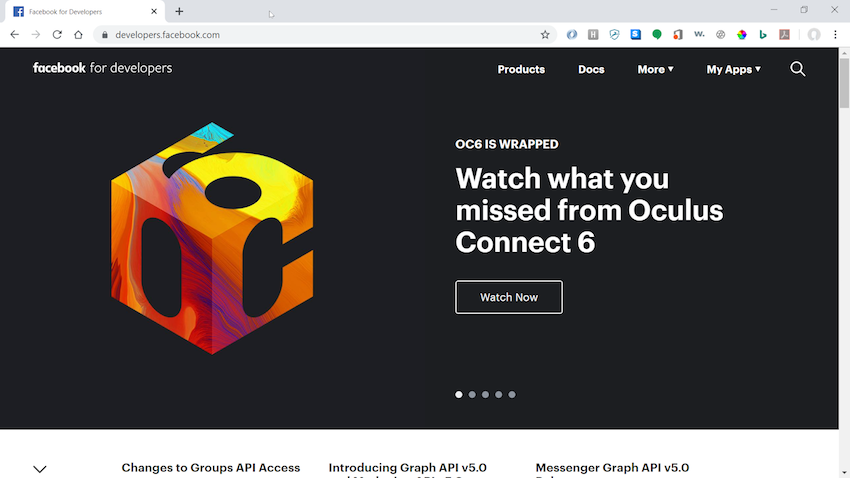
Clicking the My Apps menu item drops down your Facebook app menu, which shows all of the apps currently under your account, as well as an option to create a new app.
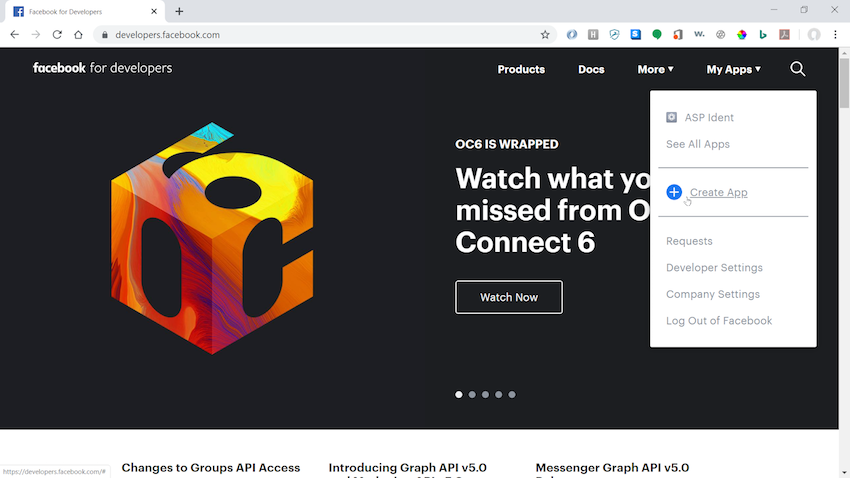
Clicking Create App brings up the following dialog:
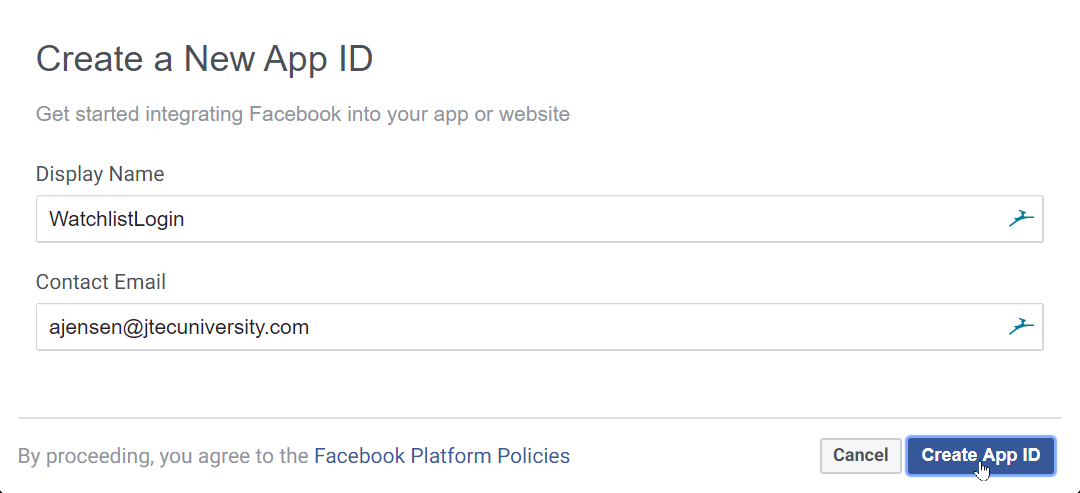
At this point, you must provide a display name for your Facebook app as well as a contact email, then click the Create App ID button. You’ll be prompted with a Recaptcha to verify your authenticity as a human, and then you’ll be taken to the dashboard for your new app.
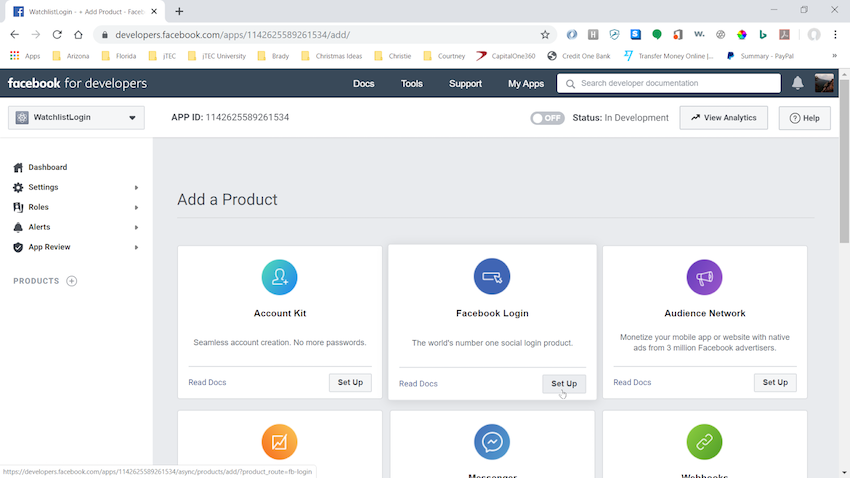
On this page, click the Set Up button on the Facebook Login card.
The Quickstart wizard displays a page asking you to choose a platform, but bypass this for now. Instead, click the Settings link in the menu on the left side of the page:
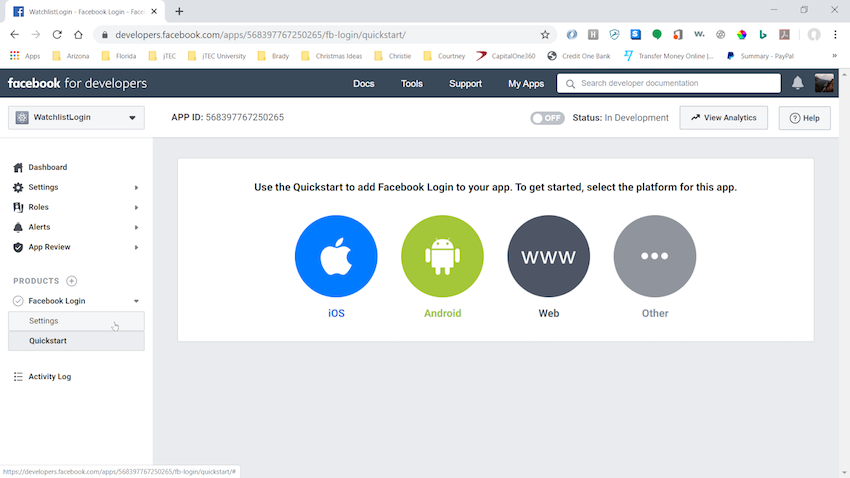
You are then presented with the Client OAuth Settings page:
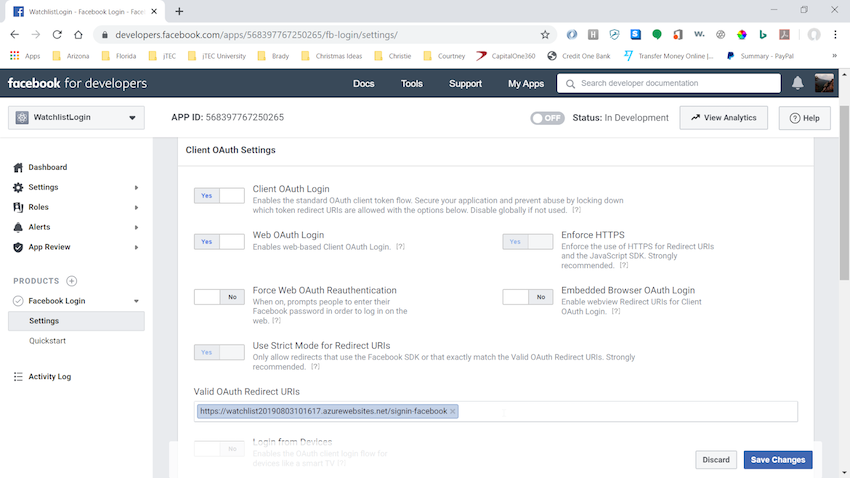
Scroll down until you see the Valid OAuth Redirect URIs text field. This is where you enter all of the URIs that will be using this app for login purposes. Enter your development URI for your application (in the example above, I’ve used the URI for my Watchlist app on Azure from the MVC course), and include /signin-facebook appended to the URI. The /signin-facebook URI is the default callback for the Facebook authentication provider, so later we’ll configure the Facebook authentication in your project to handle requests at this route.
After entering the appropriate URIs, click the Save Changes button, then click the Settings > Basic link in the navigation menu at the left side of the page.
Make note of the App ID and App Secret displayed on this page. The App Secret is initially hidden, but you can show it by clicking the Show button in the App Secret text box. You’ll save both of these values into your .NET Core project in the next section.
That’s pretty much all there is to setting up a Facebook app for login. Most third-party services follow a similar pattern with similar terminologies, so once you learn one, you can figure out the others.
Now let’s turn back to your project and configure it to use your third-party Facebook login.
Store the Facebook App ID and App Secret
ASP.NET Core has an excellent tool for securely storing sensitive settings like your Facebook App ID and App Secret. These settings are saved to your application configuration using the Secret Manager, which is invoked through the Package Manager Console window.
In the Package Manager Console, run the following commands to store the App ID and App Secret for your Facebook login app using the Secret Manager:
dotnet user-secrets set "Authentication:Facebook:AppId" "<your App ID>" --project "<path to your project’s .csproj file>"
dotnet user-secrets set "Authentication:Facebook:AppSecret" "<your App Secret>" --project "<path to your project’s .csproj file>"
When each command finishes, you’ll see a success message such as the following displayed in the console window:
Successfully saved Authentication:Facebook:AppId = <your App ID > to the secret store.
Configure Facebook Authentication
Now that the App ID and App Secret are safely stored, you need to configure the Facebook authentication service for use. Do this in the ConfigureServices method in the Startup.cs file:
services.AddDefaultIdentity<IdentityUser>()
.AddDefaultUI(UIFramework.Bootstrap4)
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddAuthentication().AddFacebook(facebookOptions =>
{
facebookOptions.AppId = Configuration["Authentication:Facebook:AppId"];
facebookOptions.AppSecret = Configuration["Authentication:Facebook:AppSecret"];
});
Notice that the AppID and AppSecret configuration strings must exactly match those defined when you saved the values to the Secret Manager. If the strings don’t match, the values won't be retrieved.
You should now be able to run your application, click Log in, and see an option to sign in with Facebook displayed on the right-hand side of the page.
Let’s Recap!
In this chapter, you learned that authentication with external accounts is made relatively simple in .NET Core and that the process is fairly similar for all third-party authenticators, typically requiring three basic steps:
Create an app in the development space of the provider.
Store tokens assigned by the provider in the
SecretManager
.Configure the provider for the application.
You also learned the specifics of creating a Facebook app under your personal Facebook developer account for use with Facebook account authentication in an MVC application. You are now ready to add additional authentication options to your application, such as Twitter, LinkedIn, Github, Google, and Microsoft.
In the next chapter, you’re going to learn how to control access to application pages and data using role and policy-based security methods.