Spring Boot is known for being configurable, and that includes actuator endpoints! You can customize and enrich the existing endpoints as well as create completely new ones from scratch! How cool is that? 🤓 It's simpler than you might expect. Let's see how it's done.
Create a /release-notes
endpoint
When a new version of a software is released, a document is usually also released that details the list of new features and bugs that were fixed in that version. This document is called release notes. Our client Tom has one last requirement. He wants to see the release notes easily by just hitting an endpoint. Actuator framework seems to be a good fit for implementing this requirement. Let's make it happen!
The overall process is similar to creating an MVC controller method. We will create a class with a specific method, and then an annotated method which will return the actual response content. So create a class called ReleaseNotesEndPoint
, then annotate it with @Component
and @Endpoint(id="release-notes")
. The id is the URL path of the endpoint. Then create a method that returns the actual release notes text. You need to annotate with @ReadOperation
.
package com.openclassrooms.watchlist.actuator;
import org.springframework.boot.actuate.endpoint.annotation.Endpoint;
import org.springframework.boot.actuate.endpoint.annotation.ReadOperation;
import org.springframework.stereotype.Component;
@Component
@Endpoint(id="release-notes")
public class ReleaseNotesEndpoint {
String version10 = "** Version 1.0 ** \n\n"
+ "* Homepage added \n"
+ "* Item creation form added \n"
+ "* View the watchlsit page added \n";
@ReadOperation
public String releaseNotes() {
return version10;
}
}
The endpoint is created, but is not exposed yet, so let's expose it like we did the other standard endpoints:
management.endpoints.web.exposure.include=info,health,beans,env,metrics,httptrace,release-notes
Now go to the http://localhost/actuator/release-notes
URL in your browser and see the release notes.
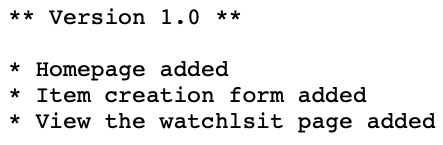
Make /release-notes
navigable
We can always return the full history of release notes, but that could get unnecessarily big. So let's give the user the option of just seeing the release notes of a certain version. Do you remember /metrics
endpoint from the last chapter? It was a navigable endpoint that you could get more details about a section of data. Let's do the same thing for our /release-notes
endpoint.
We are going to add another method to our class, which will receive the version as a parameter. Based on that, it will return release notes of that version. The parameter should be annotated with @Selector
. Before that, create another class attribute called version11
, and add some features to it that we added later to the application.
package com.openclassrooms.watchlist.actuator;
import org.springframework.boot.actuate.endpoint.annotation.Endpoint;
import org.springframework.boot.actuate.endpoint.annotation.ReadOperation;
import org.springframework.boot.actuate.endpoint.annotation.Selector;
import org.springframework.stereotype.Component;
@Component
@Endpoint(id="release-notes")
public class ReleaseNotesEndpoint {
String version10 = "** Version 1.0 ** \n\n"
+ "* Home page created\n"
+ "* Adding a new item form added\n"
+ "* View the watchlist page added\n\n\n"
;
String version11 = "** Version 1.1 \n\n"
+ "* Reading from OMDb API added \n"
+ "* Actuator endpoints added \n";
@ReadOperation
public String releaseNotes() {
return version11 + version10;
}
@ReadOperation
public String selectReleaseNotes(@Selector String selector ) {
if ("1.0".equals(selector)) return version10;
else if ("1.1".equals(selector)) return version11;
else return releaseNotes();
}
}
Now if you go to http://localhost/actuator/release-notes
, you see the full release notes. However, if you go to http://localhost/actuator/release-notes/1.1
, you will see the release notes of version 1.1.
Let’s recap!
In this chapter, you saw how to:
Create a completely new actuator endpoint from the scratch by:
Creating a class
Annotating the class with
@Component
and@Endpoint(id="NAME")
To return a result, create a method then add
@ReadOperation
.
Make a new endpoint navigable by adding a method with
@selector
parameter.
You're almost to the end! Now it's time for a quick recap! 😎