Install and use a Python Package
Once you have located a Python package that you want to make use of, you can use the command pip install <package>
to install it. We are going to make use of the matplotlib
package - a package that helps you draw graphs using Python.
Let’s do it together, step by step. 😊
First, create a file called generate_graph.py
and put in the following contents:
import matplotlib.pyplot as plt
plt.figure()
plt.plot([1, 2, 3, 4, 5])
plt.show()
Now, try running the command python generate_graph.py
from your command line. If the command executes successfully, and the script generates a graph then great - you already have the matplotlib
package installed.
If the script doesn’t execute successfully then you may well be getting an error message similar to the following:
~ → python generate_graph.py Error processing line 1 of /Users/george/anaconda3/lib/python3.7/site-packages/matplotlib-3.0.2-py3.7-nspkg.pth: Traceback (most recent call last): File "/Users/george/anaconda3/lib/python3.7/site.py", line168, in addpackage exec(line) File "<string>", line1, in <module> File "<frozenimportlib._bootstrap>", line 580, inmodule_from_spec AttributeError: 'NoneType' object has no attribute 'loader' Remainder of file ignored Traceback (most recent call last): File "generate_graph.py", line1, in<module> import matplotlib.pyplotasplt Module Not Found Error: No module named 'matplotlib'
If this is the case, you need to install the matplotlib
package using pip install matplotlib
:
→ pip install matplotlib Collecting matplotlib Using cached https://files.pythonhosted.org/packages/4a/5c/ced2e398aad26b24a7beaa2b22830811dfc953c73a0b3b930e26c12d1d19/matplotlib-3.2.2-cp37-cp37m-macosx_10_9_x86_64.whl Requirement already satisfied: numpy>=1.11 in ./anaconda3/lib/python3.7/site-packages(frommatplotlib)(1.18.4) Requirement already satisfied: kiwisolver>=1.0.1 in ./anaconda3/lib/python3.7/site-packages(frommatplotlib)(1.0.1) Requirement already satisfied: python-dateutil>=2.1 in ./anaconda3/lib/python3.7/site-packages(frommatplotlib)(2.7.5) Requirement already satisfied: cycler>=0.10 in ./anaconda3/lib/python3.7/site-packages(frommatplotlib)(0.10.0) Requirement already satisfied: pyparsing!=2.0.4,!=2.1.2,!=2.1.6,>=2.0.1 in ./anaconda3/lib/python3.7/site-packages(frommatplotlib)(2.3.0) Requirement already satisfied: setuptools in ./anaconda3/lib/python3.7/site-packages(fromkiwisolver>=1.0.1->matplotlib)(40.6.3) Requirement already satisfied: six>=1.5 in ./anaconda3/lib/python3.7/site-packages(frompython-dateutil>=2.1->matplotlib)(1.12.0) Installing collected packages: matplotlib Successfully installed matplotlib-3.2.2
Now, if you try and run your generate_graph.py
script using python generate_graph.py
, it should execute successfully and the following graph should appear:
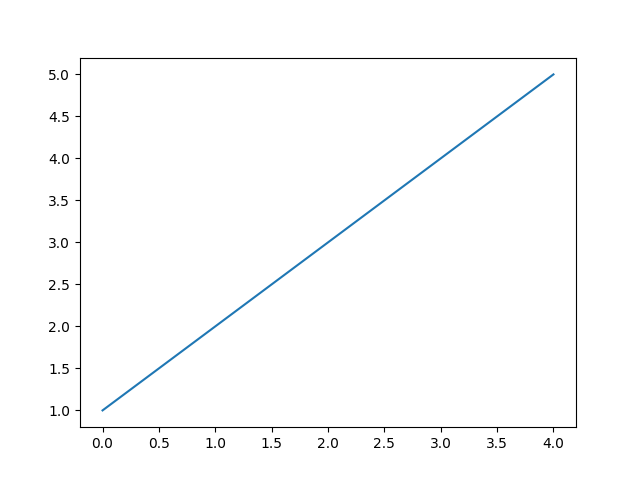
Congratulations, you have successfully installed and used your first Python package, matplotlib
. 😄
To double-check your installation of matplotlib
, use the following screencast:
Check which packages you have installed
To check which Python packages you have installed you can run two different commands in your terminal, pip list
or pip freeze
.
pip list
lists all of the packages you have installed (and their dependencies) in a human-readable format:
→ pip list Package Version Location -------------------------------------------------------------- alabaster 0.7.12 anaconda-client 1.7.2 anaconda-navigator 1.9.6 anaconda-project 0.8.2 appnope 0.1.0 appscript 1.0.1 asn1crypto 0.24.0 aspy.yaml 1.3.0 astroid 2.1.0 astropy 3.1 atomicwrites 1.2.1 attrs 18.2.0 awscli 1.16.168 Babel 2.6.0 backcall 0.1.0 backports.os 0.1.1 backports.shutil-get-terminal-size1.0.0 bcrypt 3.1.6 ...
pip freeze
lists the packages you have installed (but not their dependencies) in a format suitable for storing in a file (often called a requirements.txt
file) - something that we will cover in much more detail at a later stage. To demonstrate:
→ pip freeze alabaster==0.7.12 anaconda-client==1.7.2 anaconda-navigator==1.9.6 anaconda-project==0.8.2 appnope==0.1.0 appscript==1.0.1 asn1crypto==0.24.0 aspy.yaml==1.3.0 astroid==2.1.0 astropy==3.1 atomicwrites==1.2.1 attrs==18.2.0 awscli==1.16.168 Babel==2.6.0 backcall==0.1.0 backports.os==0.1.1 backports.shutil-get-terminal-size==1.0.0 bcrypt==3.1.6 ...
Another useful command with pip
is pip show <package(s)>
, which shows you useful information about one or more packages that you have installed. For example, to get more information about the requests
package that I have installed I would run the following command:
→ pip show requests Name: requests Version: 2.23.0 Summary: PythonHTTPforHumans. Home-page: https://requests.readthedocs.io Author: KennethReitz Author-email: me@kennethreitz.org License: Apache2.0 Location: /Users/george/anaconda3/lib/python3.7/site-packages Requires: urllib3, idna, certifi, chardet Required-by: Sphinx, conda, conda-build, anaconda-project, anaconda-client
I can use pip show
to get information about multiple packages by separating the package names with a space:
→pip show requests numpy pandas Name: requests Version: 2.23.0 Summary: Python HTTP for Humans. Home-page: https://requests.readthedocs.io Author: Kenneth Reitz Author-email: me@kennethreitz.org License: Apache2.0 Location: /Users/george/anaconda3/lib/python3.7/site-packages Requires: idna, certifi, chardet, urllib3 Required-by: Sphinx, conda, conda-build, anaconda-project,a naconda-client --- Name: numpy Version: 1.18.4 Summary: NumPy is the fundamental package for array computing with Python. Home-page:https://www.numpy.org Author: Travis E. Oliphantetal. Author-email: None License: BSD Location: /Users/george/anaconda3/lib/python3.7/site-packages Requires: Required-by: tables, seaborn, scikit-learn, PyWavelets, pytest-doctestplus, pytest-arraydiff, patsy, pandas, odo, numexpr, numba, mkl-random, mkl-fft, matplotlib, h5py, datashape, Bottleneck, bokeh, bkcharts, astropy --- Name: pandas Version: 0.23.4 Summary: Powerful data structures for data analysis, timeseries, and statistics Home-page: http://pandas.pydata.org Author: None Author-email: None License: BSD Location: /Users/george/anaconda3/lib/python3.7/site-packages Requires: python-dateutil, pytz, numpy Required-by: seaborn, odo
If you are looking for a bit more information about installing packages and using them in scripts, or if you haven’t quite managed to use pip
to show or list your packages, check out the following screencast for some extra tips:
Exercise
You are working as a marketeer. Your manager has recently been trying to learn Python because they think that they will be able to use it to automate some repetitive tasks. They have sent you a Python script that they want you to try and run, but have just popped into a meeting and aren’t around.
Download this script and try to run it from the command line using Python.
If it executes successfully the first time around, then great, you don’t need to do anything else! If it doesn’t, however, your job is to investigate which packages you need to install from PyPI, install them, and run the script successfully!
Let’s Recap!
pip install <package>
is used to install Python packages from the command line.Once you have installed a Python package, you can
import
it into a Python script and make use of its functionality.pip
has different commands available that you can use to view which packages you currently have installed.
Now that you have had a go at installing and using Python packages, let’s focus a little more on the different methods for installing packages and modules and when we should use them.