Programming languages are a careful compromise between being easy for humans to understand and computers to interpret.
And as a Python developer, your code has two responsibilities:
Explain to the computer what actions to take.
Explain to other developers what actions are taking place.
Why do other developers need to know what my code is doing? It’s the computer that has to follow the code, not them!
Code is read much more often than it’s written! After you’ve written the code, other developers will usually need to use your classes and functions, extend them to allow new functionality, and fix bugs.
Imagine you’re looking through a Python project and encounter this function:
def check_for_adjustment(value):
return (value % 4 == 0) and (value % 100 != 0 or value % 400 == 0)
What does it do? Is it working properly? Can we use it for ourselves?
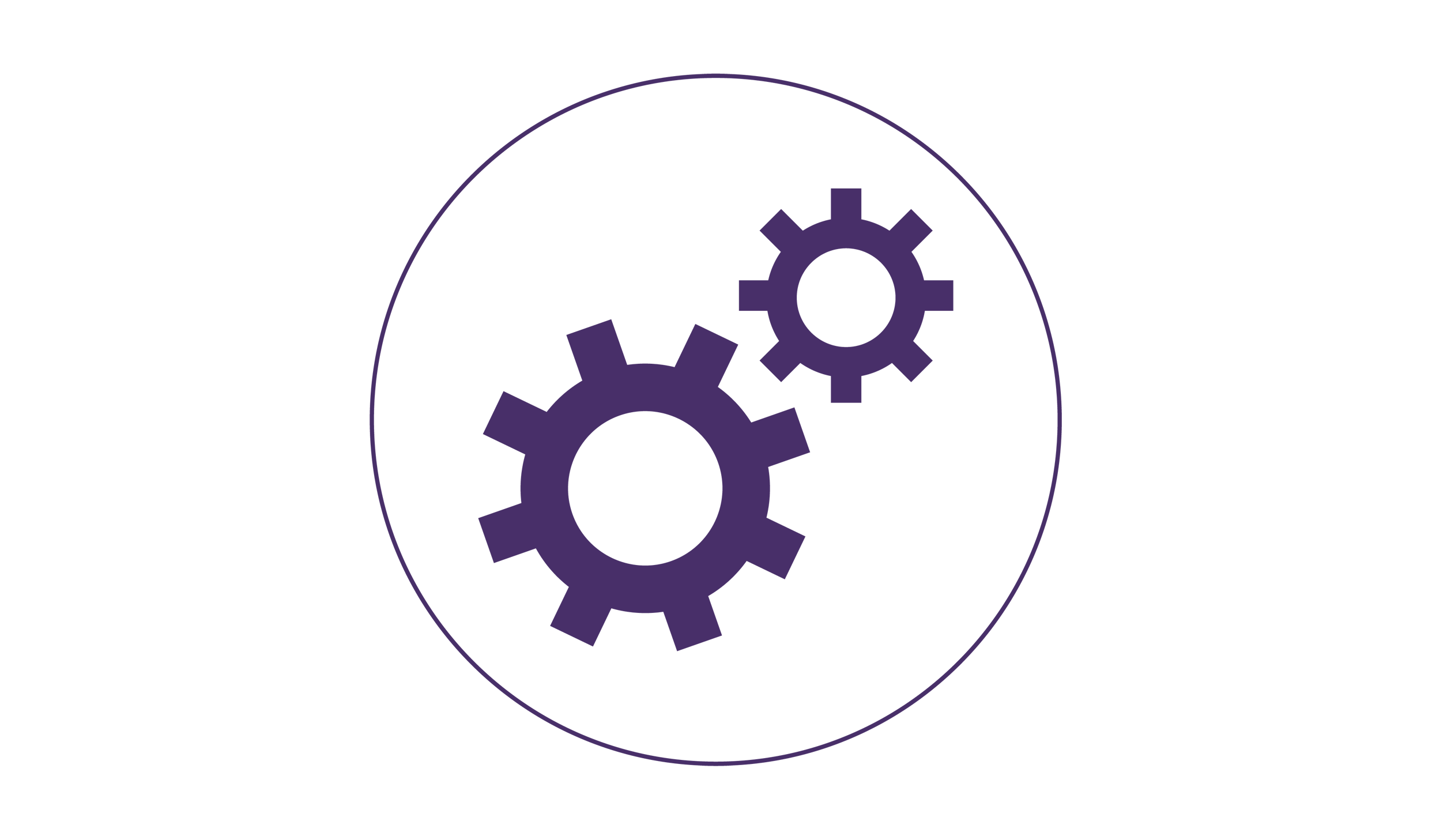
The developer who wrote this has chosen unhelpful names for the function and variables. Maybe this would have been clearer:
def is_leap_year(year):
# Leap years are all multiples of 4
if year % 4 == 0:
# Centuries are not leap years except 1600, 2000, 2400, etc.
if year % 100 == 0:
return (year % 400 == 0)
else:
return True
return False
These functions are equivalent to the computer, but the second version is much more developer-friendly. Now you can see at a glance what the function does (it has a clear name), and it doesn’t fry your brain trying to figure out the logic! 🤯
The PEP 8 Style Guide
Luckily, most code that you read in Python has been written in a way that allows human developers to skim it and know what to do with it quickly. 🎉
Such code follows the PEP 8 style guide, a long list (over 7,000 words!) of suggested practices for Python developers, created in 2001, written by Guido van Rossum, Barry Warsaw, and Nick Coghlan, and occasionally updated to reflect developments in the language.
Seven thousand words is too much! How am I supposed to remember all those rules?
Don’t worry - you won’t have to remember all the rules! Some PEP 8 parts are relatively obscure, so I’ve picked out the most important ones to cover in this course.
In the first part of this course, we’ll look at the most important rules in the guide (and the reasoning behind them). You’ll even see how to use a neat tool called a linter to check how well your code matches PEP 8!
Let’s get started!
Naming Conventions
As you saw above in the leap year example, it’s super helpful to anyone reading your code if you choose sensible names for your variables, classes, and functions. Also, PEP 8 recommends the following:
Write variable names in lowercase with underscores:
variable = 6
my_other_variable = 7
Write constant variables in uppercase with underscores:
CONSTANT = 6
MY_OTHER_CONSTANT = 7
Write class names in title case without punctuation:
Class
MyOtherClass
Write module names in lowercase without underscores:
import openpyxl
import numpy
Novels are written with consistent capitalization and punctuation to make them easier to read. Even if your code isn’t intended as light bedtime reading, you’ll be making things better for future developers by following these rules.
In Python, you don’t create variables whose value can’t be changed. But if you intend that a value be kept constant, then simply writing it in ALL_CAPS tells other developers not to write code that changes it. And because of PEP 8, they should understand. 🙂
It would be best if you also avoided abbreviations such as:
def mltply(first, second):
return first * second
This is partly because it might be confusing, but more practically because if some developer is searching through the codebase looking for a function that multiplies numbers, they’ll search for something like “mult,” which would return multiply_numbers
, but not mltply
.
Comments and Documentation
As you saw above in the leap year example, sometimes it’s useful to leave a comment to explain parts of your code that might not be immediately obvious.
# Centuries are not leap years except 1600, 2000, 2400, etc.
However, it’s also possible for comments to be distracting and unhelpful:
my_variable += 10 # Add 10 to my_variable
What does the wisdom of the Python community say about this in PEP 8?
Write in full sentences using English (unless it’s internal code for a team that prefers another language).
Avoid comments that contradict the code.
But why would anyone do that?
It would be silly to do that intentionally, sure. But with unnecessary comments, someone could update the code, but not the comment. Then you end up with something like:
my_variable += 40 # Add 10 to my_variable
And this leads to the next points:
Keep the comments up-to-date when the code changes.
Avoid inline comments as they look untidy.
Place a single space between the hash symbol and the text (so it looks different to code that’s been commented out.
Indent comments to the same level as the subsequent line of code for readability.
# Correctly-indented comment
def foo(arg):
# Correctly-indented comment
pass
# Incorrectly-indented comment
def foo(arg):
# Incorrectly-indented comment
pass
Documentation Strings (“Docstrings”)
Have you ever seen comments like this?
def multiply(first, second=1):
"""Multiply two numbers.
Keyword arguments:
first -- the first multiplicand
second -- the second multiplicand (default 1)
"""
return first * second
print(multiply(13, 77))
print(multiply.__doc__)
The yellow lines are docstrings - special comments written at the start of a function or class and used for documentation purposes. For example, docstrings allow automated tools to access the text, such as to present a pretty list of all your functions and what they do.
For open-source projects, and projects with large teams, documentation is important as it allows other real humans (yes, like you!) to understand the code without delving into the source code.
Hang on - I thought that comments didn’t affect the text? How can these tools access my docstrings?
Docstrings at the start of a function or class are special, and can be accessed using the __doc__
attribute:
print(multiply.__doc__)
When to Use Docstrings
If it’s for a project that won’t be using automated documentation, then there’s no need! When I’m doing personal projects or work for non-technical clients, I never write them, as they wouldn’t get used!
But if it’s an open-source project, or with a team that uses this documentation feature, then you should add docstrings to everything public, but not private classes or methods.
Are we still talking about Python? You can declare variables and methods as public/private in Java and C++, but is it possible in Python?
Indeed that’s not how Python works - so there’s a danger that people could use your private “helper” functions when you don’t want them to.
So the official PEP 8 recommendation is to write docstrings only for public classes and methods that you want people to use, but nothing else.
Then if you want to modify or remove them later, you won’t have to worry about breaking other people’s code that relies on them!
Let’s Recap!
The PEP 8 style guide contains many recommendations to help Python developers write code that is understandable by each other.
Variables, classes, and modules should be given helpful names and written in the suggested style.
Comments should be used in moderation, kept up-to-date, and indented the same as the following line of code.
Docstrings are special comments that can be accessed with
__doc__
.Classes and methods that are intended to be private should not have docstrings.
Next chapter, you'll see how to layout a Python file in a way that’s friendly for people reading it!