Now that you’ve learned how to build the scaffolding for your page layouts using the Bootstrap 5 grid, it’s time to start adding components to the structure!
One essential component of any website is navigation, so it’s a good place to start.
Add Navigation to Your Website
The framework has a powerful and responsive navigation bar. You can implement it using a nav
element with a .navbar
class.
<nav class="navbar">
...
</nav>
Navbars also need a .navbar-expand
class for responsive collapsing of the menu on smartphones and small screens. The .navbar-expand
class acts on the viewport width at which the navbar is expanded or collapsed. The navbar breakpoint can be changed by adding a class modifier { -sm , -md , -lg , -xl , -xxl }
to the .navbar-expand class, like this: .navbar-expand-md
.
For the moment, let’s add the navbar-expand
class without a size-related class modifier. You’ll see how to collapse the navbar for smaller screens later.
<nav class="navbar navbar-expand">
...
</nav>
For most websites, branding is displayed along the main navigation bar. Bootstrap has a .navbar-brand
class, which serves this purpose in the navigation bar component. You can add the name of the website to the element provided for this as follows:
<nav class="navbar navbar-expand">
<a class="navbar-brand" href="...">First name Last name</a>
</nav>
The basic formatting of the brand isn’t very original, so let’s use the Bootstrap 5 utility classes to make it more interesting!
In this case, add the .text-uppercase
and .fw-bold
classes to your a.navbar-brand
. Then, wrap the name in a span tag to give it other layout properties. To do this, add these classes to your span element: .bg-primary
.bg-gradient
.p-1 .rounded-3
and .text-light
:
<a class="navbar-brand text-uppercase fw-bold" href="/index.html">
<span class="bg-primary bg-gradient p-1 rounded-3 text-light">John</span> Doe
</a>

Ta-dah! The logo is customized without typing a single line of CSS!
Now let’s take a look at navigation links. The navigation elements should be put in an unordered list containing the elements <ul>
, <li>
and <a>
, according to the classes shown in this code snippet:
<nav class="navbar navbar-expand">
<div class="container">
<a class="navbar-brand text-uppercase fw-bold" href="/index.html">
<span class="bg-primary bg-gradient p-1 rounded-3 text-light">John</span> Doe
</a>
<ul class="navbar-nav">
<li class="nav-item active">
<a class="nav-link" href="#johndoe">About me</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#skills">Skills</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#portfolio">Portfolio</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#contact details">Contact details</a>
</li>
</ul>
</div>
</nav>
You might have noticed that the first <li>
element in this code snippet includes an .active class. This element defines one of the navigation elements as the “active” element, i.e., the one currently selected. Create your navbar’s HTML in a way that ensures only one of the navigation items has this class.
There’s also another element you should be familiar with by now: div.container
. It does exactly the same as everywhere else it’s used on the page—in this case, it contains the navbar
elements.
Your navbar is now customized with the name of the website and the navigation links. Now let’s add some color!
To change the background and text colors in the navigation bar, you can add CSS styles created from scratch. However, you can also apply Bootstrap background color utility classes to elements. These are particularly useful at the prototyping stage, as they allow you to adjust the background of an element (not just the navbars!) using the Bootstrap themes.
As you saw in the previous chapter, the navbar background is light gray. You can use the utility class .bg-light
to change its color:
<nav class="navbar navbar-expand bg-light">
...
</nav>
The classes .navbar-light and .navbar-dark
are other practical tools for managing Bootstrap colors. These classes adjust the color of elements in the navbar, such as the brand and navigation links, to provide a contrast with the chosen background. When you use a navbar in a dark color, you should add the .navbar-dark class, and when you use a light-colored navbar, add the .navbar-light
class.
Here’s how to do it:
<nav class="navbar navbar-expand bg-light navbar-light">
…
</nav>
After all the changes made to the header section and the navbar, your home page prototype should look like this:

It’s starting to take shape! Nice work!
Create Responsive Navigation
Now you have a basic navbar configuration — it’s time to make sure it’s responsive. Since Bootstrap is a mobile-first framework, and the navbar classes used so far in the code don’t include any class modifiers for size, the navbar will look the same for all screen sizes.
When you add content such as logos and extra links to the navbar, it will end up being too big for the smallest screen sizes. To make it responsive to different screen sizes, you need to collapse it for smaller screens and expand it for larger screens.
For the portfolio website, we want the navigation to look like the image below:
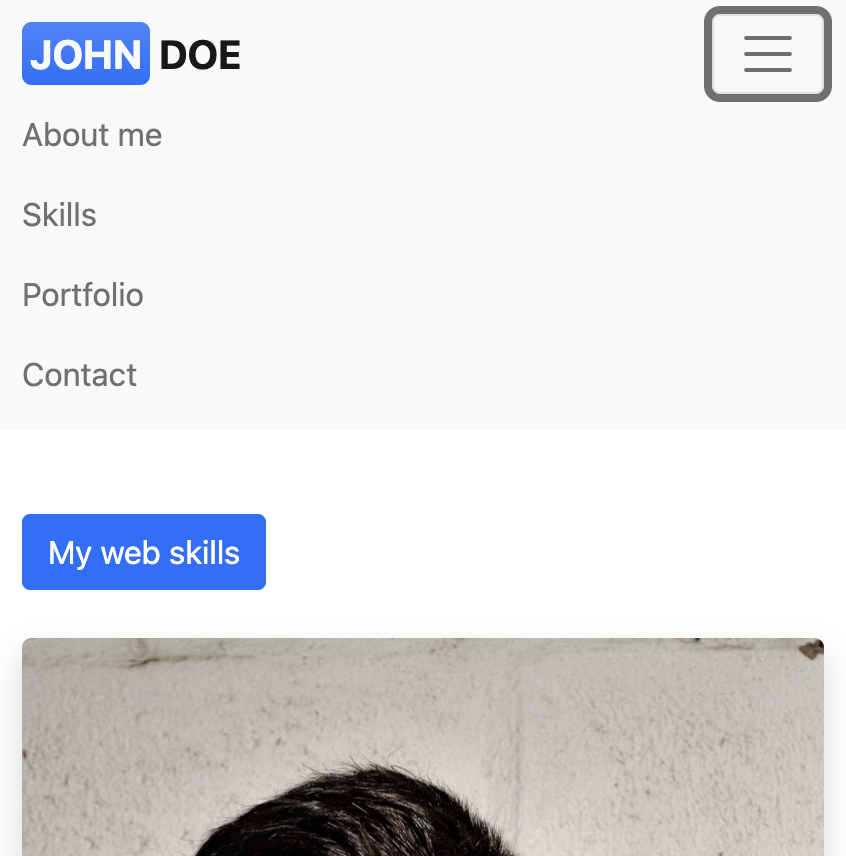
You can draw a few conclusions from the responsive navigation for your website above:
When the width of the viewport is large or above, the navigation menu is expanded and displays all of the content.
When the viewport is smaller than the breakpoint used (
-sm|-md|-lg|-xl|-xxl
) the navigation menu is hidden, and a “toggle navigation” button is displayed, which expands or collapses the menu.
It might seem like a lot to learn, but fortunately, Bootstrap makes it easy.
The first step in implementing responsive navigation is to use the size-related class modifier on the .navbar-expand
class of the <nav>
element. Remember that class modifiers in Bootstrap work upwards. Using the .navbar-expand
class without a class modifier will apply the class to all sizes from extra-small upward.
To make the navigation responsive in the way specified above, you need to apply the class modifier that expands the navbar menu for large screens and above. So, you’ll need to change it to .navbar-expand-lg
:
<nav class="navbar navbar-expand-md bg-light navbar-light fixed-top">
...navbar content...
</nav>
Next, you need to add the toggle navigation button, which displays or hides your website’s navigation menu. For responsive navigation, add a button just after the <a>
element with the class .navbar-brand
, with the following attributes and content:
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
As you can see, the button has a .navbar-toggler
class and data attributes such as data-bs-toggle=
" collapse
" and data-bs-target=
" #navbarNav
". These attributes allow you to target the right <div>
element using the class .collapse
and the ID #navbarNav
without writing a single line of JavaScript.
To do this, add the classes .collapse and .navbar-collapse to the <div> , which wraps the list of navigation links.
Finally, add the ID attribute with a value that matches that of the data-bs-target
attribute of the toggle button—in this case, navbarNav
:
<div class="collapse navbar-collapse justify-content-end" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" href="#johndoe">About me</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#skills">Skills</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#portfolio">Portfolio</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#contact details">Contact details</a>
</li>
</ul>
</div>
You might be wondering what the class justify-content-end
is; it’s another Bootstrap 5 utility class that allows you to add the property flex justify-content: flex-end
.
Bootstrap navigation is in flex
display by default, which means that you can use flex
properties on the child nav
elements to position them on the right or left, or in the center.
Over to You!
Your mission for this chapter is to make your portfolio website’s navigation responsive:
Make the menu responsive for medium screens and smaller.
Configure your menu to stay at the top of the page when scrolling down.
Put the button that displays the mobile menu on the right.
Use flex utility classes to position your elements.
Let’s Recap!
The class
.navbar
allows you to add a navigation bar to a website.You can change the color of navbars using the classes
.navbar-light
and.navbar-dark
.You need to add a class modifier to
.navbar-expand
to make the navbar responsive.Implementing a responsive navigation component displays or hides the menu, depending on screen size.
You can customize the typography using the Bootstrap 5 utility classes.
You can add icons with Bootstrap 5.
The homepage is starting to take shape with the new UI components you’ve learned how to implement! Now let’s take a look at some of the Bootstrap 5 components and how to implement them in your portfolio website.