Follow a Four-Step Method
Debugging is an essential skill for all developers by applying a methodology to find and eliminate bugs in a program. The key word is “methodology.” Debugging isn’t something you can make up as you go along—you need to be methodical about it and use your detective skills! 🤠 This is essential when several different bugs are involved, as is usual when a program crashes. See below for an effective methodology you can use.
Step 1: Describe the Problem as Clearly as Possible
This is where you describe the “what” and the “when” of the problem. For example, imagine you have a problem with your car. 🚘 To find out the “what” and “when,” you need to ask:
Is the problem coming from the tires (puncture, underinflation, bent rims, etc.) or the engine (flat battery, trouble with the ignition)?
When does the problem occur, and when doesn’t it?
Saying “it doesn’t work” isn’t enough. Here are some helpful questions to think about:
What exactly is wrong?
Where could the source of the problem be located?
Under what conditions does the bug appear?
Your Python interpreter will also help you by sending an error message containing more detail (we’ll return to this in the following chapters). Unfortunately, if your description of the problem isn’t clear, your colleagues or Google won’t be able to help you.
Here are two examples of requests for help. Which one best describes the “what” of the problem?
“My program has crashed. Can someone help me? Here’s the code…”
“I’m trying to retrieve information from the Twitter API via this address [URL] using the requests module version 2.25.1. I’m getting the following error message after a few seconds, but I don’t understand it. Can someone help me? Here’s the code…”
Did you guess right? OK, that was easy, but accurately describing your problem will be a huge help.
Step 2: Define a Test
One of my former managers used to say, “if it’s not tested, it doesn’t work.” It’s common sense! Think about it—if your program doesn’t pass the tests to prove it is functional, how can you be sure it works at all?
In a test, you can simulate example scenarios to ensure the program responds to them correctly. Testing is crucial before using the program, as it allows you to identify and anticipate potential bugs.
Testing is also helpful once the program is in operation. Using tests, you can ensure your fixes have solved the problem and not created another one.
Step 3: Come Up With Theories
This is where you need to become a detective and form hypotheses on the source of the bug. This step aims to make the “what” and “when” from the first step clearer by answering “why.” This stage is essential to finding the root cause of the bug. You don’t just want to deal with the symptoms—you need to find out why the problem is occurring to stop it from returning. Finally, you must find the real cause to solve the problem once and for all.
Going back to the program having trouble retrieving data from the Twitter API, you could ask:
Is the URL correct?
Is the API still working?
Does the most recent update of the requests module mean that it has to be used differently?
Do you definitely have an access token?
These questions should help you find the real cause. You can then test it in the following step.
Step 4: Resolve the Bug
You’ve formulated all the hypotheses you can think of on the bug’s origin. It’s time to resolve it by implementing a solution which will depend on the type of bug you’re dealing with (as you saw in the previous chapter). For example, you might need to:
Make changes to the behavior of the code;
Refactor the structure of the code;
Update modules or software.
However, just as there are different possible causes for a bug, several possible solutions may exist. You’ll need to implement one or more of these to resolve the bug.
Of course, there’s a good chance you’ll need to go through the four stages several times—as many as needed for your program to work properly. As you gain experience, you’ll get quicker and better at this.
You now have a scientific method for resolving bugs. Let’s make it even better by using two very effective problem-solving techniques that apply to IT—and life in general!
Apply the Five Whys Method
The 5 whys method is handy for getting to the root of a problem. As its name suggests, it involves asking “why” five times to analyze the situation in greater depth.
For example, a developer might ask, “Why am I not happy and productive?”. They may come up with the answer that they need to work on a beach rather than in an office. But a more detailed analysis of the situation will probably show that things aren’t that simple. To discover the real cause of their unhappiness and unproductiveness, they must ask “why” several times.
When you apply the 5 whys method, you get a cause and effect chain that can be linear, tree-shaped, or circular. Here are a few examples:
Linear

This is the classic configuration when using the 5 whys method.
Why am I late? Because I didn’t get up in time.
Why? Because I went to bed late.
Why? Because I was watching Master Chef.
Tree-shaped
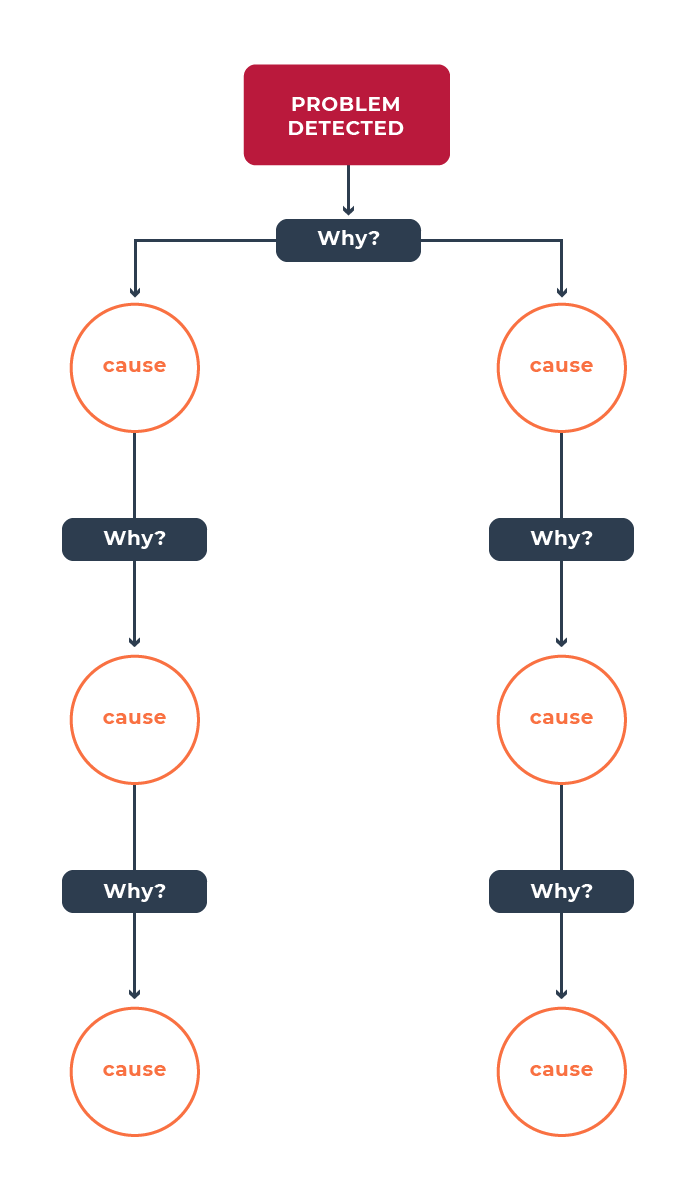
Each branch corresponds to a cause. The branches should be limited to the most relevant explanations to keep it clear.
Why has the car run out of gas?
Branch 1: Because neither my wife nor I filled it up. Why? Because we both thought the other was going to do it.
Branch 2: Because the gas station ran out of fuel. Why? Because the truck bringing it had an accident.
Circular
In this case, the cause and effect chain goes around in a circle. Therefore, to solve the problem, you must break the chain.
Why can’t I sleep? Because I’m stressed.
Why? Because I can’t sleep.
Why? Because I’m stressed.
Let’s go back to the Twitter API example and try to answer the 5 whys:
Why did the program crash? Because I don’t have an access token.
Why? Because I didn’t read the API documentation stating that it’s mandatory.
Why? Because I don’t know the technical vocabulary necessary to search the documentation.
Why? Because I haven’t taken the time to learn it.
Why? Because I’m so busy at work that I haven’t had a spare moment to do it yet!
What did you notice? You can see several underlying problems, including the ability to read the documentation. Throughout your career, you’ll have to read a lot of documentation on using APIs, modules, frameworks, objects, etc., which will be full of technical vocabulary! 😝
The developer needs to address all the different causes, especially the underlying problem. Otherwise, this sort of bug will repeat. Think back to the last time a program you were running crashed, and try to use the 5 whys to analyze the situation.
Use the 80-20 Principle
The 80-20 principle, or the Pareto principle, explains that 80% of results stem from 20% of causes. This principle is widely referred to because it applies to many different areas.
When applied to resolving bugs, the Pareto principle suggests that 20% of the causes are responsible for 80% of bugs. Therefore, it’s essential to list the potential causes and rank them by how often they appear.
You could survey your colleagues and ask, “Which bugs have you encountered most often, and when?”
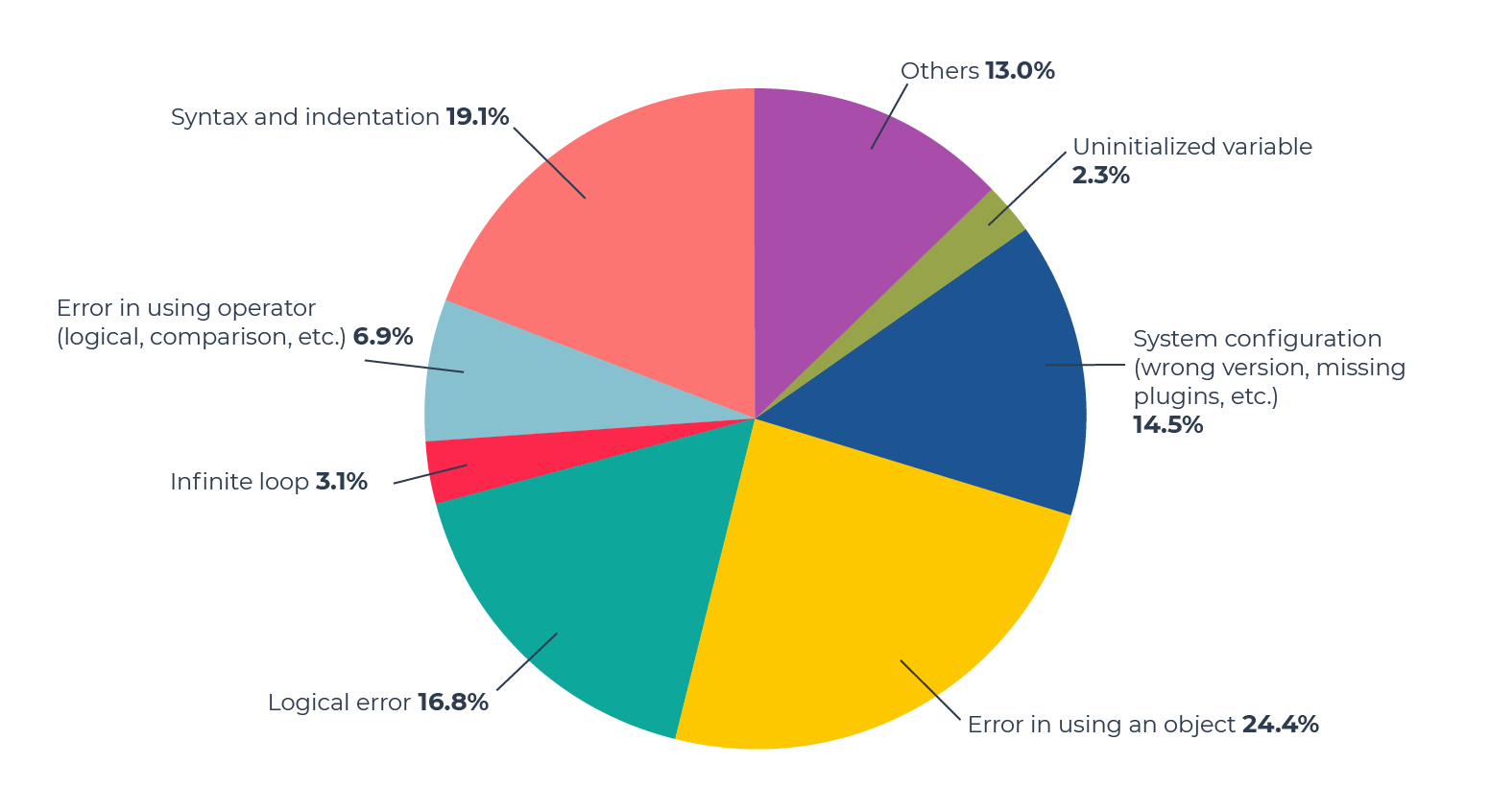
You can also look at the most common questions on forums such as Stack Overflow to find out where the most common 80% of bugs originate.
If you remember this and focus on the most common and plausible 20% of causes, you’ll save time, money, and sanity!
Let’s Recap!
Debugging is a methodology for finding and resolving bugs. It’s composed of four steps:
Clearly describe the what and when of your bug.
Create a test to check whether the program (or part of the program) is working correctly.
Come up with hypotheses on the bug’s origin, remembering that there may be more than one cause.
Resolve the bug by focusing on the cause, not just its consequences.
The 5 whys method helps you get to the root of the problem by asking “why” several times.
According to the Pareto principle, 80% of bugs result from around 20% of the programmer’s actions.
Now you’re equipped with all the key information, let’s get practical and install a debugging environment. Are you ready?