Spot the Most Common Errors in a Django Project
Django is a powerful framework for creating websites and APIs. It can, of course, generate the standard Python exceptions. However, it also has its own list of exceptions, which you can find in its documentation.
The Django framework can be split into seven categories:
Main Django exceptions: These are the general exceptions specific to how Django works. For example, this could be an incorrect field, unused middleware, or a view that doesn’t exist.
URL resolver exceptions: These exceptions are raised if the user searches for an endpoint that doesn’t exist.
Database exceptions: These are raised when you’re working with databases (i.e., if you enter an incorrect value for a field).
HTTP exceptions: This kind of exception is raised when Django sends or receives incorrect requests via HTTP protocol (i.e., the user cancels a download).
Session exceptions: These are exceptions to do with Django’s session management. They may be generated if a session is deleted in an HTTP request just before another request tries to access the session.
Testing framework exceptions: These occur when Django’s test client detects errors (i.e., an overly long chain of redirects).
In the screencast below, you’ll see a few Django exceptions:
In the following screencast, we will focus on two classic errors, TemplateDoesNotExist
and PageNotFound
.
I strongly recommend reading the documentation. It will save you a lot of time. Like standard exceptions in Python, Django exceptions help you find the cause and type of bug.
Finally, you can also use Stack Overflow to help you—it’s a programmer’s best friend. Type in “Django,” and a list of the questions asked with this tag will appear. You can then browse through the answers and find some valuable information!
Spot the Most Common Mistakes in a Flask Project
Like Django, Flask has its own exceptions and the standard Python ones. When a Flask exception is raised, it generates a stack trace containing useful information. You’ll get more details if you set the variable debug to True
, like in line 11 of the image below:
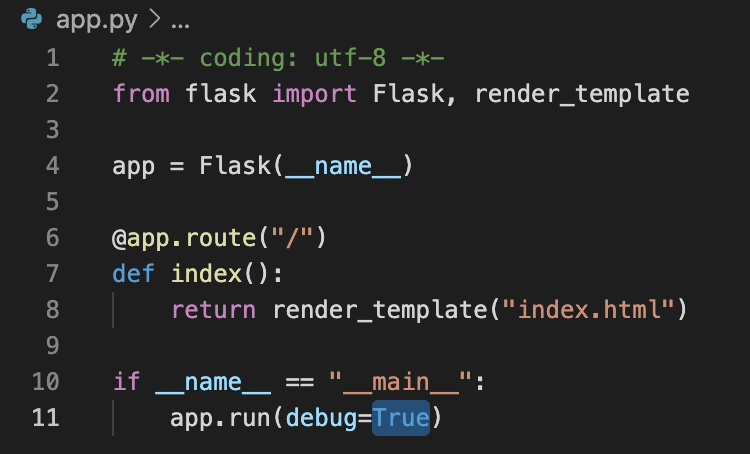
However, don’t forget to set it back to False
before deploying the application to production, as you only use the True
mode for the development phase. Users will panic if they see all these messages they don’t understand, and you may accidentally reveal information about your code that you would rather keep secret!
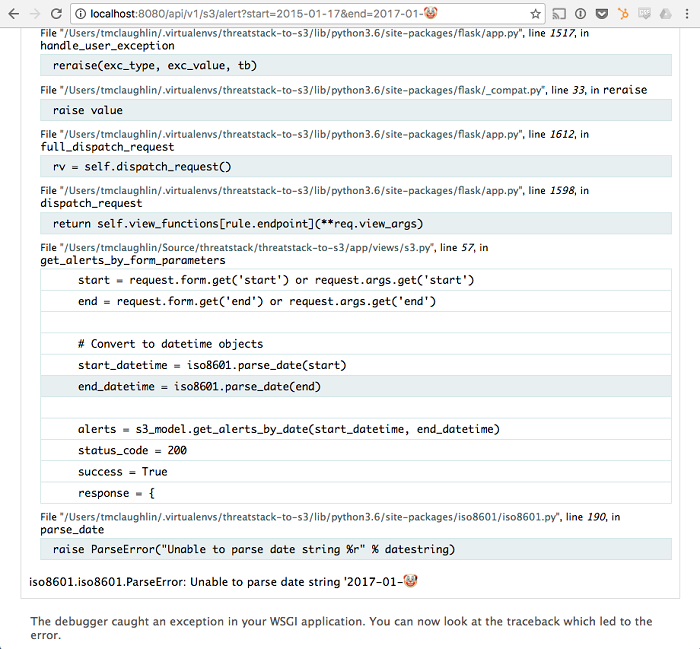
If you type “exception” into the search bar of the Flask official website, you’ll see a list of links explaining some of these errors.
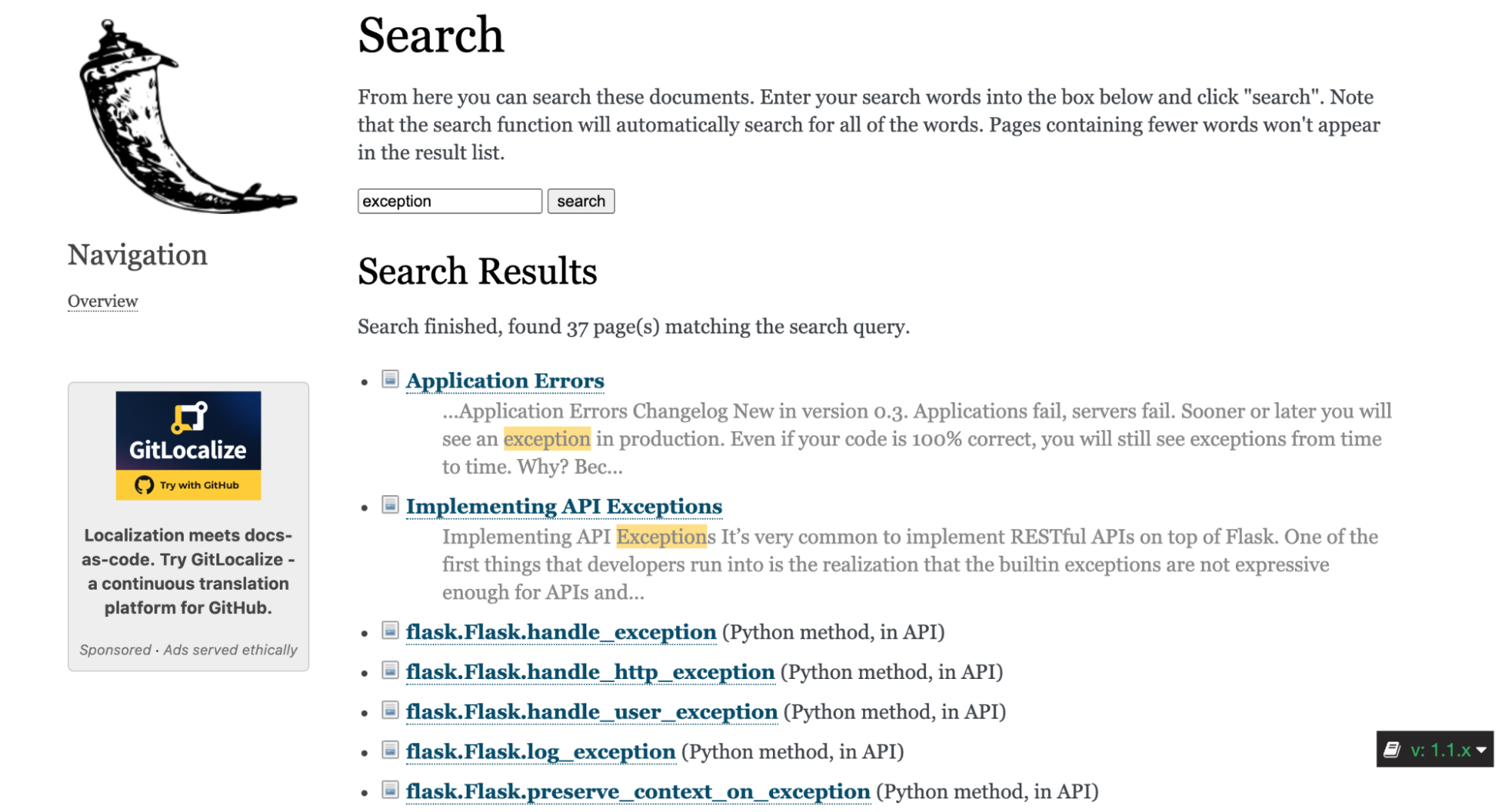
These are some of the classic errors:
Internal Server Error: This common error happens when an unhandled error occurs in a route.
routing_exception: If the correspondence between the program’s routes and the URL fails, this exception is raised. It’s a NotFound type exception.
Method Not Allowed Error: This exception is often raised because you’ve only authorized the GET method, not the POST method, in the route decorator.
Let’s look at a few Flask errors in the screencast below:
In the following screencast, we will look at the most common errors you'll encounter when programming using Flask, including error 405. You’ll also see how to generate your own errors and catch them for redirection to a specific template.
The Flask Stack Overflow page contains many frequently asked questions. Here are two of them:
I can’t receive data from an HTML form via Flask.
Flask isn’t working on port 80.
Look at the answers on Stack Overflow—there’s a lot of helpful information.
Over to You!
You’ve just seen some of the most common errors. Thousands of others exist! The Stack Overflow website will help you find answers to your questions. However, why not try answering questions from fellow debuggers? It’s a good habit to get into, and you’ll notice the difference in your debugging skills. 😉
Let’s Recap!
Django and Flask have specific exceptions in addition to the standard Python exceptions.
The Django and Flask documentation contains explanations of the causes of the most common exceptions.
Stack Overflow provides answers to help resolve the most common bugs in Flask and Django.
Now you know how to read and understand errors; it’s time to get rid of them once and for all! But first, test what you’ve learned in this part with a quiz!