To communicate with a computer, the first thing we need is a language. Of course, we know that humans have many different languages, which work in different ways. There are many different language families, which are groups of languages derived from a common ancestral language—the Indo-European language family (including English, French, and Spanish), the Sino-Tibetan languages (including Mandarin), the Semitic languages (such as Arabic), etc.
These languages often share much more than an alphabet. Maybe you can see where I’m going with this! For example, we use verbs when we want to talk about an action, and nouns to talk about objects or people (a chair, a picture, a girl, etc.).
Programming languages use a very similar logic. In this course, we’ll be looking at structured programming, which is one of the most well-known programming paradigms.
In structured programming, we break down problems into microtasks, which are modular and independent of each other. That’s exactly what we’re doing here!
Use Variables Like Nouns
A variable is a way of referring to an object. In everyday language, it’s what we’d call a “name.” After all, a word is just a label given to an object so we can talk about it! A variable has a value, and a word which allows us to access this value. You can either read the value or change it.
We need to create two variables in our program to count the number of moves and the player’s score, which we’ll initialize to zero at the start of the program.
You can declare all of the variables in a specific section of the pseudocode to provide an overview of the different variables the program contains. This goes just before the keyword Start
, like this:
DeclareVariable algorithm
Variable
moves ← 0
score ← 0
Start
[type pseudocode here]
End
To go further, let’s assume each move is worth a certain number of points (described below). Let’s see how the variables can be updated in this table, which sums up the different possibilities:
Action | Variable | Value added |
a move | moves | +1 |
a move to the right | score | +10 |
a move to the left | score | +20 |
a move up | score | +10 |
a move down | score | +20 |
Using the maze below, let’s try to create an algorithm to get to the end. We need to take into account each update of each variable.
Here’s the pseudocode:
Variable algorithm
Variable
moves ← 0
score ← 0
Start
Move right
moves ← moves + 1
score ← score + 10
Move up
moves ← moves + 1
score ← score + 10
Move up
moves ← moves + 1
score ← score + 10
Move left
moves ← moves + 1
score ← score + 20
End
At the end of the game, the moves
variable contains the value of four (four moves x one point per move), and the score
variable contains the value of 50 (one move left x 20 points per move left, plus two moves up x 10 points per move up, plus one move right x 10 points per move right).
Use Functions Like Verbs
Now let’s see how to make it simpler to update the two variables by grouping the instructions together in the same block. I’m sure you’ll have noticed that we had to write the instructions to change the values of the two variables several times.
We’re going to use what we call a function in programming. This is a block which groups together several actions. You can reuse this block as many times as you want by simply stating its name. A function can also be seen as a machine that takes in data, processes it, and outputs other data. It’s a sort of mini algorithm.
When a function is “called,” the program leaves the section of code where it was and starts to execute the first line inside the function.
We’re now going to create our function based on the following criteria:
Name |
|
Input parameters |
|
Instructions |
|
Output parameters | No output parameters |
We can describe this function in pseudocode like this:
Function update_moves_score(moves, score, move_points)
Start
moves ← moves + 1
score ← score + move_points
End
As you can see, there’s no difference from the description of an algorithm in pseudocode, except that we’ve added the input parameters in brackets. You can add the output values (if there are any) before the end of the function, with the keywordReturn
.
We’re going to use the update_moves_score
function to modify the previous algorithm, like this:
Variable algorithm
Variable
moves ← 0
score ← 0
Start
Move right
update_moves_score(moves, score, 10)
Move up
update_moves_score(moves, score, 10)
Move up
update_moves_score(moves, score, 10)
Move left
update_moves_score(moves, score, 20)
End
Over to You!
Context
Let’s go back to our maze.
Take a look at the following pseudocode for the player’s moves around the maze:
Moves algorithm
Variable
moves ← 0
score ← 0
Start
Move up
Move up
Move right
Move left
Move down
Move down
End
In this exercise, you’re going to add a function to calculate the score based on the number of moves made.
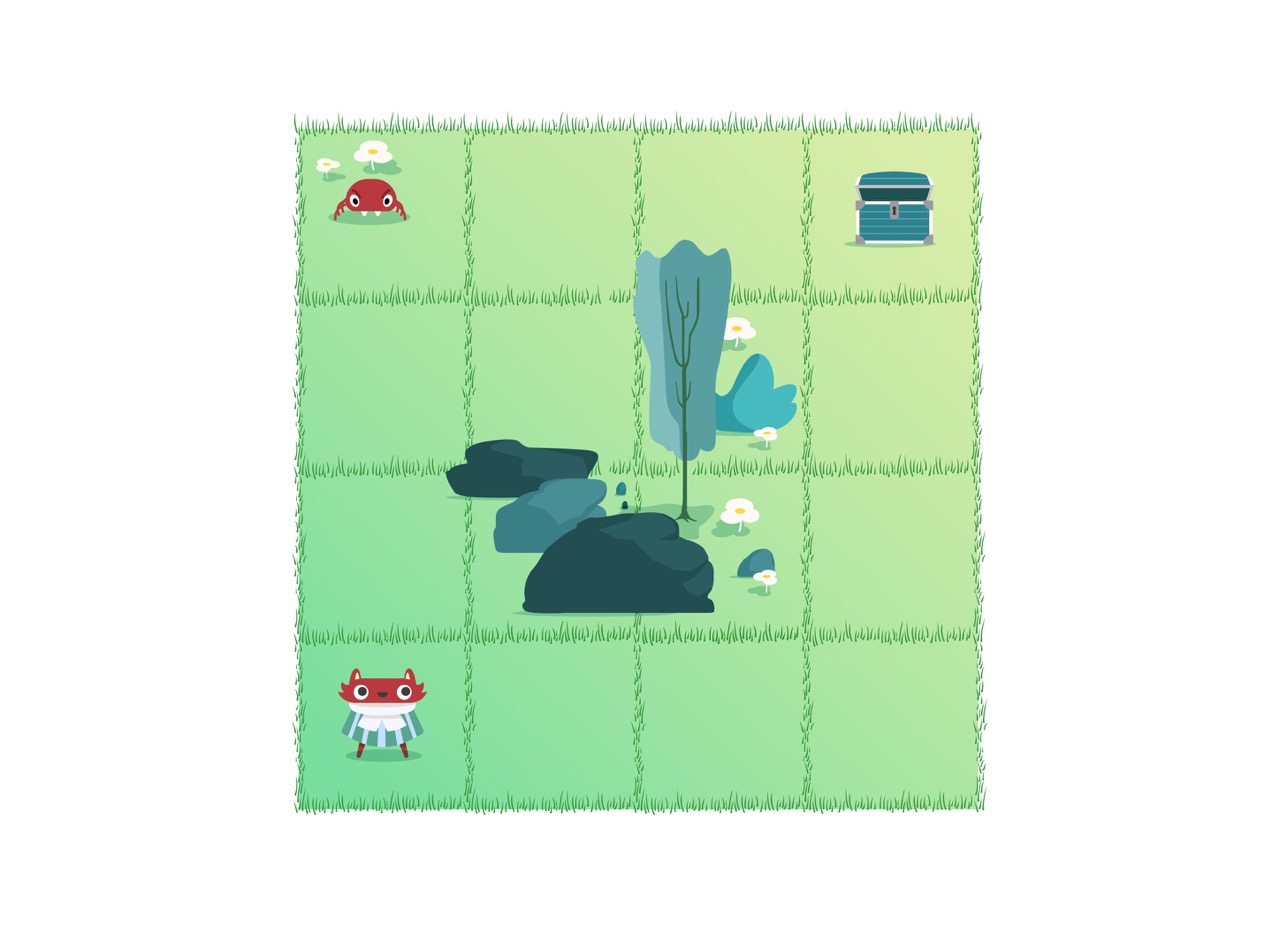
Instructions
Take the pseudocode above and adapt it according to the following instructions:
Each move increases the
moves
variable by one.The
score
increases depending on the direction of movement:left:
50 points/number of moves
right:
70 points/number of moves
up:
25 points/number of moves
down:
90 points/number of moves
If the player lands on the same square again, the score is decreased by 25 points.
If the player goes back to Start, the
score
is reset to zero.
Your Mission
Create a program taking into account all of the above instructions. If you like, you can write the current value of the
moves
andscore
variables next to each instruction.Create a function to update the
moves
andscore
variables.Create a function to reset the
score
variable to zero.Adapt your algorithm to include the two new functions.
Check Your Work
Here are the four steps to follow to achieve the final result of this exercise:
First Step: Create the Moves Algorithm
Moves algorithm
Variable
moves ← 0
score ← 0
Start
Move up
moves ← moves + 1
score ← score + 25
Move up
moves ← moves + 1
score ← score + 25
Move right
moves ← moves + 1
score ← score + 70
Move left
moves ← moves + 1
score ← score + 25 - 25 (The player goes back to the same square)
Move down
moves ← moves + 1
score ← score + 90 - 25 (The player goes back to the same square)
Move down
moves ← moves + 1
score ← score + 90 - 25 (The player goes back to the same square)
score ← 0 (The player goes back to the start so the score is = 0)
End
Second Step: Create the Function to Update the Moves and Score Variables
Function update_moves_score (moves, score, move_points)
Start
moves ← moves + 1
score ← score + (move_points / moves)
End
Third Step: Create the Function to Reset the Score
Function reset_score(score)
Start -
score ← 0
End
Fourth Step: Adapt the Algorithm With the Two Functions Created Above
Moves algorithm
Variable
moves ← 0
score ← 0
Start
Move right
update_moves_score(moves, score, 25)
Move up
update_moves_score(moves, score, 25)
Move up
update_moves_score(moves, score, 70)
Move down
update_moves_score(moves, score, 50 - 25)
Move down
update_moves_score(moves, score, 90 - 25)
Move left
update_moves_score(moves, score, 90 - 25)
reset_score(score)
End
Let’s Recap!
Most programming languages share a similar basic structure.
Variables are used to store information to reference and use in a computer program.
A function is simply a piece of code which you can reuse, rather than writing it out over and over again.
Functions generally take data as an input, process it, and return a result.
You’ve discovered the world of variables and how to simplify your program by breaking it down into functions. Now it’s time to test your knowledge with a quiz in the following chapter. Good luck!