Forums des Zéros
Une question ? Pas de panique, on va vous aider !
[Android] Problème de Dialog Box
Erreur lors de l'apparation d'une Dialog Box depuis une autre classe
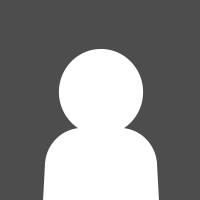
31 mars 2013 à 15:41:33
Une question ? Pas de panique, on va vous aider !