Forums des Zéros
Une question ? Pas de panique, on va vous aider !
[C#] Meilleur approche pour l'envoi de requête web
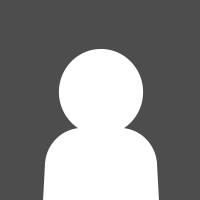
20 août 2019 à 1:04:05
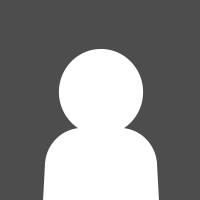
30 août 2019 à 13:57:54
Je recherche un CDI/CDD/mission freelance comme Architecte Logiciel/ Expert Technique sur technologies Microsoft.