Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Cercle en Java
Sujet résolu
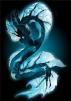
24 septembre 2011 à 14:53:58
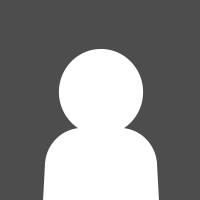
24 septembre 2011 à 16:56:07
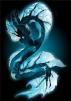
24 septembre 2011 à 19:00:16
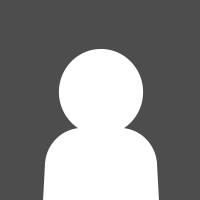
24 septembre 2011 à 20:56:53
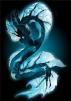
25 septembre 2011 à 11:36:49
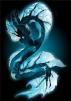
26 septembre 2011 à 22:41:10
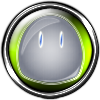
26 septembre 2011 à 22:52:50
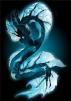
26 septembre 2011 à 22:57:07
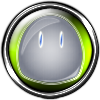
26 septembre 2011 à 23:11:31
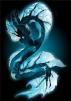
26 septembre 2011 à 23:17:49
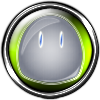
26 septembre 2011 à 23:25:50
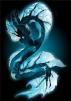
26 septembre 2011 à 23:40:41
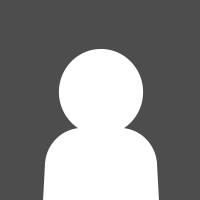
27 septembre 2011 à 20:19:28
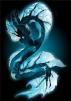
27 septembre 2011 à 20:36:02