Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Comportement calendrier en JQuery
mois précédent - mois suivant
Sujet résolu
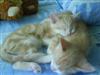
31 octobre 2010 à 11:45:31
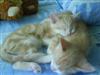
1 novembre 2010 à 12:25:05

3 novembre 2010 à 14:41:41
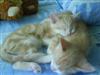
3 novembre 2010 à 14:46:17