Forums des Zéros
Une question ? Pas de panique, on va vous aider !
[Cube 3D][PyGame][Régulation des FPS (molette)]
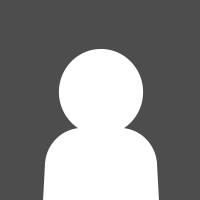
24 août 2014 à 12:10:43
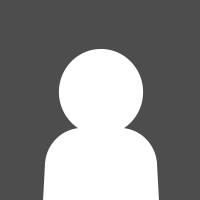
25 août 2014 à 11:33:32
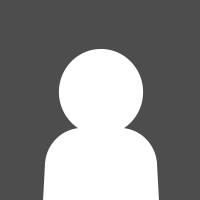
25 août 2014 à 13:00:41
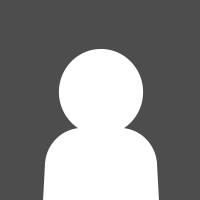
25 août 2014 à 15:40:34
Une question ? Pas de panique, on va vous aider !