Forums des Zéros
Une question ? Pas de panique, on va vous aider !
[FAIT][Défis] #2 : Le jeu de la vie !
SDL
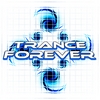
10 septembre 2011 à 15:35:00
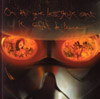
10 septembre 2011 à 17:38:48
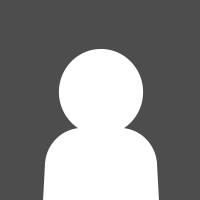
10 septembre 2011 à 17:59:27

10 septembre 2011 à 19:30:20
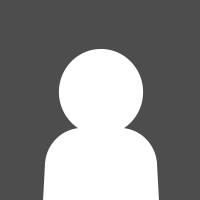
11 septembre 2011 à 0:45:33

11 septembre 2011 à 1:07:12
Zeste de Savoir, le site qui en a dans le citron !
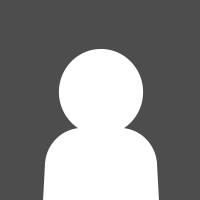
11 septembre 2011 à 1:10:22

11 septembre 2011 à 10:22:37
Staff désormais retraité.
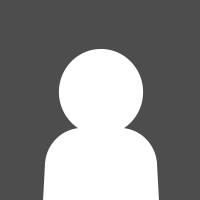
11 septembre 2011 à 10:33:45

11 septembre 2011 à 10:34:47
Staff désormais retraité.
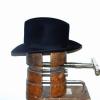
11 septembre 2011 à 14:56:46

11 septembre 2011 à 15:09:46
Staff désormais retraité.

11 septembre 2011 à 15:31:35

11 septembre 2011 à 15:51:57
Staff désormais retraité.

11 septembre 2011 à 15:54:46

11 septembre 2011 à 16:35:19

11 septembre 2011 à 16:39:01
Staff désormais retraité.
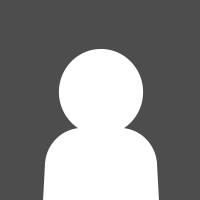
11 septembre 2011 à 17:18:13
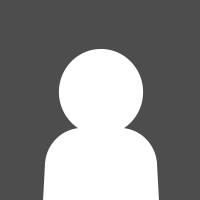
11 septembre 2011 à 17:41:14

11 septembre 2011 à 18:46:03
Staff désormais retraité.

11 septembre 2011 à 19:25:32
Zeste de Savoir, le site qui en a dans le citron !
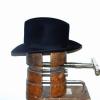
11 septembre 2011 à 20:03:46

12 septembre 2011 à 12:12:07

12 septembre 2011 à 12:27:53

12 septembre 2011 à 12:32:14
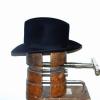
12 septembre 2011 à 14:07:55

12 septembre 2011 à 14:23:48
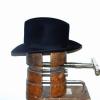
12 septembre 2011 à 14:40:04
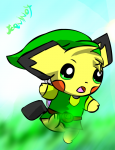
12 septembre 2011 à 14:44:20

12 septembre 2011 à 14:48:13