Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Fine_tunig_bert_for_Text_classification
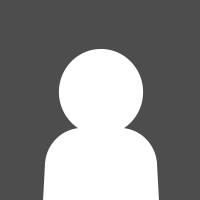
6 avril 2024 à 14:41:29

8 avril 2024 à 11:15:13
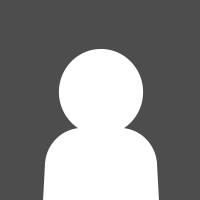
12 avril 2024 à 21:29:40
Une question ? Pas de panique, on va vous aider !