Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Multithreading Python
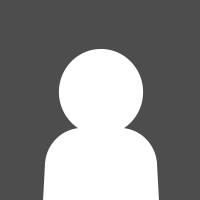
19 juillet 2019 à 12:01:25
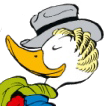
19 juillet 2019 à 13:24:49
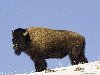
20 juillet 2019 à 11:41:26
https://zestedesavoir.com/contenus/?tag=python
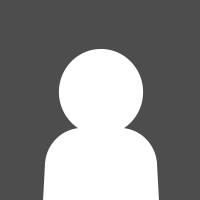
22 juillet 2019 à 9:44:09