Forums des Zéros
Une question ? Pas de panique, on va vous aider !
opencv
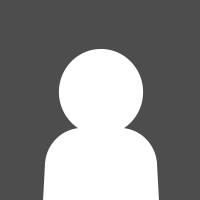
14 avril 2018 à 19:42:50
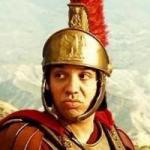
15 avril 2018 à 11:29:59
Seul on va plus vite, ensemble on va plus loin ... A maîtriser : Conception BDD, MySQL, PHP/MySQL
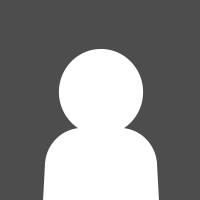
16 avril 2018 à 21:59:44
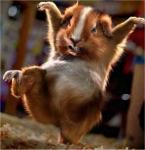
16 avril 2018 à 22:48:29
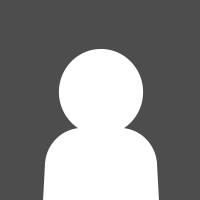
22 avril 2018 à 17:13:51