Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Problème avec XNA
Plusieurs cibles en meme temps
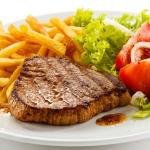
24 mars 2012 à 21:22:08

25 mars 2012 à 12:29:01
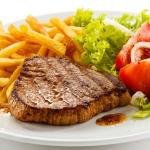
25 mars 2012 à 16:14:46
Une question ? Pas de panique, on va vous aider !