Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Requêtes python à API dans boucle infini
Sujet résolu
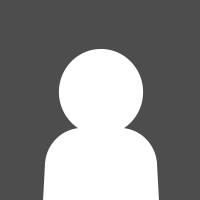
28 novembre 2021 à 19:14:16
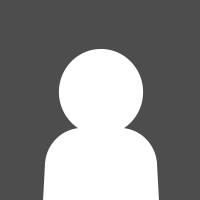
29 novembre 2021 à 15:54:06

29 novembre 2021 à 16:51:14
Une question ? Pas de panique, on va vous aider !