Inheritance Hierarchies
If a class can have a parent, it makes sense that that parent class can have a parent, too! This is where inheritance hierarchy comes in as there are multiple levels of inheritance. At the top of the hierarchy is the base class, Person, as illustrated below:
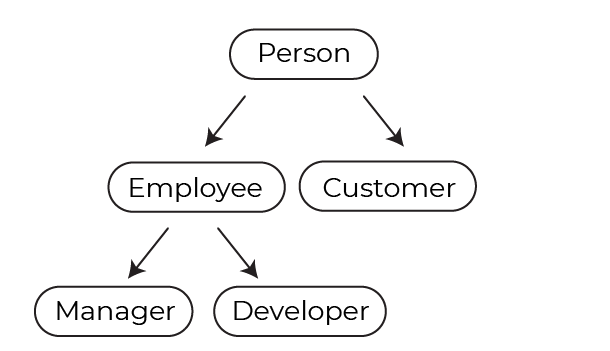
In this hierarchy, you have Employee and Customer as subclasses of Person; Manager and Developer are subclasses of Employee. As Employee inherits from Person, and Manager inherits from Employee, you’ll find that attributes from Person will end up a part of Manager, too!
Your project might contain an employee hierarchy and a separate record of the items the company sells. Remember that object-orientation and inheritance are about the relationships between concepts.
But don’t all classes inherit from object in Python? Won’t they share a common base class anyway?
Yes! We tend to leave object off of inheritance diagrams, though, because it’s not that useful to include. We’ll talk more about modeling and designing object-oriented solutions to problems in Part 3.
Multiple Inheritance
How is multiple inheritance different from an inheritance hierarchy?
Multiple inheritance involves one class having multiple parent classes. In an inheritance hierarchy, you have multiple levels of inheritance - where a class has a parent that has a parent.
Multiple inheritance has a bad reputation in object-oriented programming spaces - systems using it can be difficult to understand. Not only that, but some programming languages don’t implement it well, which causes problems.
Multiple inheritance is more often than not an incorrect solution to your problem, but there are still some situations when it is still the best available. In any case, you might run into multiple inheritance in the workplace, so we’ll cover it here.
Here’s an example:
class Cat:
def meow(self):
print(“Meow!”)
class Talker:
def say(self, to_say):
print(tosay)
class TalkingCat(Cat, Talker):
pass
salem = TalkingCat()
salem.meow()
salem.say(“Hello!”)
Here you have a Cat class and a Talker class, both of which our TalkingCat class inherits from. That means that a TalkingCat, like our Salem here, can both meow and say things!
The pattern used here is often called a Mixin - because you “mix in” some required functionality. If you’ve programmed in other languages, you might be familiar with interfaces, traits, or typeclasses - which are similar but different concepts.
Wait, what happens if two parent classes implement the same method? Which one wins?
This is one of the main problems with multiple inheritance - called the diamond problem (or deadly diamond of death). Each language that uses multiple inheritance has a different solution - Python’s is called the MRO or Method Resolution Order.
Simply put, the MRO of a class is the order of places Python will look for a method definition. It can get quite complicated - and if you’re interested in more details, you can find them in the Python documentation.
Generally speaking, though, Python will look in the left-most parent class first. So in the TalkingCat example above, Python will look in Cat first, then Talker.
Your Turn: Apply Multiple Levels of Inheritance to a Python Program
Context:
In this part of the exercise, we are introducing the concept of subclassing and method overriding. Subclassing allows us to create specialized versions of existing classes, while method overriding enables us to redefine the behavior of methods inherited from parent classes. Let’s go !
Instructions:
Let’s assume that an image can either be a GIF or a JPG. Implement these subclasses of Image, then override the display method in each to also include a reference to what type it is.
Let’s Recap!
A parent class can have a parent.
When there are multiple levels of inheritance, there is an inheritance hierarchy.
A class can also inherit from multiple parent classes, called multiple inheritance.
Now that you have an understanding of different types of inheritance, you can start to use objects in your code - including collections.