Forums des Zéros
Une question ? Pas de panique, on va vous aider !
Calculatrice
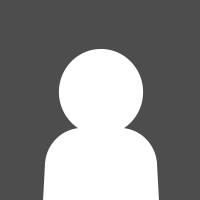
10 février 2022 à 22:20:01
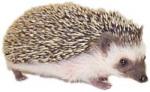
11 février 2022 à 4:04:33
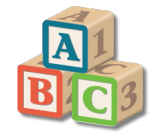
11 février 2022 à 10:34:28
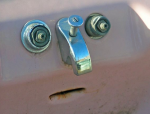
11 février 2022 à 11:17:20
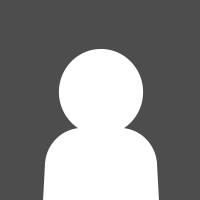
11 février 2022 à 11:25:56
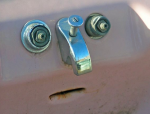
11 février 2022 à 15:51:37
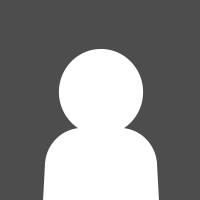
12 février 2022 à 19:14:53
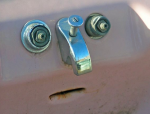
13 février 2022 à 8:56:34