Imagine again that you work in a bank. What you saw in part two was fine for treating each customer individually, but in reality, there's more than just one customer to analyze.
If you want to analyze several customers at once, you can imagine that you need a variable for each customer. For the names, this could look like this:
customerName1 = 'Marion Weaver'
customerName2 = 'Alberto Mendoza'
customerName3 = 'Katharine Tyler'
customerName4 = 'Isaac Steele'
# etc.
If you have 10 customers to analyze, wouldn't it be easier to store them all in a single variable that would contain all the information?
You're in luck! Python offers a structure, a class capable of storing multiple pieces of information as a kind of array. This structure is called a list. Let's see how to use it.
Declare a List to Store Your Items
Lists are objects that can contain a collection of objects of any type. We can have a list containing several integers (1, 2, 50, 2,000 or more, it doesn't matter), a list containing floats, a list containing strings, or even a list mixing objects of different types.
Lists are ordered objects, i.e., each item of the list is associated with a number corresponding to its order in the list. This number is called an index and it starts at 0 (not 1!). The first item is therefore associated with index 0, the second with index 1, etc.
Declaring a list is quite similar to the declaration of any variable seen so far: via a name to which we associate a list of items to be stored in this name.
For example, here is the list containing the names of four customers:
customerName = ['Marion Weaver', 'Alberto Mendoza', 'Katharine Tyler', 'Isaac Steele']
Now that your list is created, you can perform two basic operations:
Access a value at a given index
Change the value at a given index
In both cases, the code consists of the name of the variable followed by [
, the value of the index and ]
.
For example, if you made a mistake on the name of the first customer and you want to correct their name:
# assign the value 'Marianne Weaver' to the first name in our list
# it is index 0, because indices start at 0 in python!
customerName[0] = 'Marianne Weaver'
To print it, you can write the following line:
print(customerName[0])
Python also lets you use negative indices to access or modify an item. The index -1 corresponds to the last item of the list, -2 to the second last, and so on. You can also access an index range by using the :
operator. For example, 1:3
will let you access items two to four.
# print the last item
print(customerName[-1])
# access the second item to the 3rd
print(customerName[1:3])
# access all items from the beginning to the second
print(customerName[:2])
Here you have manipulated lists of strings, but you can do the same thing with the amount in each individual's account:
amountAccount = [10000, 150, 300, 1800.74]
For example, the following list is completely valid:
strangeList = [4, 10.2, 'Marion Weaver', ['another list', 1]]
# print the 4th item of the list
print(strangeList[3])
Try it for Yourself
Work with lists yourself in the following exercise.
You can find the solution here.
What should we do now if a new customer is added to our analysis? When I try to do customerName[4] = '...', it returns an error!
Don't panic! You can't access an index that doesn't already exist in a list in Python... however, lists have many methods that let you remedy this.
List Methods
Now consider that you want to list animals in order of cuteness (from cutest to "least" cute). We can easily start with a list of four animals: fox, koala, owl, and otter. These days, while browsing the internet, you will inevitably come across this image:
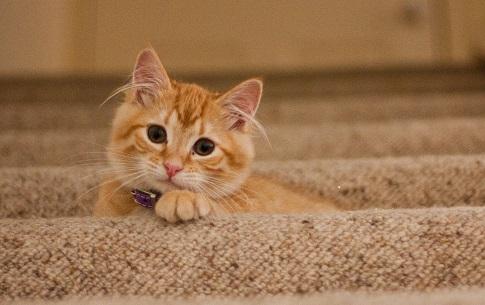
And now you want to add the cat to the first position!
The good news for our little cat is that the lists are fully editable, whether it's the number of items, their order, etc. Thanks to the different list methods, we can:
search for a specific item in the list.
add a new item at the end.
insert a new item at a specific index.
delete an item from the list.
Add Items to a List
You can create an empty list in Python and then add the items one by one via the append method:
list = []
list.append(7)
list.append(5)
print(list) # => [7, 5]
The first statement creates an empty list, very creatively named
list
.You then add the integer 7 to the end of the list. So Python will add it to index 0.
Finally, you add the integer 5, which will be stored at the next index, which is index 1.
Here are some other methods that are essential to know about lists:
insert
to insert a new item at a specific position. For example,list.insert(1, 12)
will insert the integer 12 at index 1, moving the old item 1 to index 2 and so on.extend
: similar to append, but with another list. This allows you to concatenate several lists together.remove
: searches for the given item in the list and deletes the first occurrence. For example, if you want to delete 5 from your list, you can use :list.remove(5)
.index
: this method lets you find the index of the first occurrence of an item to be searched for in our list;Keyword
del
to delete an item according to its index.
Now let's try out some of these methods:
list = []
list.append(7) # -> [7]
list.append(5) # -> [7, 5]
list.insert(1,12) # [7, 12, 5]
list[0] = 4 # -> [4, 12, 5]
list.remove(12) # [4, 5]
list.index(5) # prints 1
list.extend([1, 2, 3]) # [4, 5, 1, 2, 3]
del list[3] # [4, 5, 1, 3]
Let's break down these few lines:
The first three lines correspond to what was seen before.
You then add 12 to index 1. The value that was in position 1 is moved to position 2.
You then replace the value at index 0 with 4.
With the
.remove()
method, you remove the integer 12 from our list.You then ask for the index of the first item 5 in our list (here in second position, so return 1).
You add the list
[1, 2, 3]
after our initial list.And, finally, you delete the item located at position 4 in our list.
In the end, this leaves you with the final list: [4, 5, 1, 3]
.
Keep Control of Your List
The len()
function lets you retrieve the size of your list:
list = [1, 2, 3]
len(list) # will print 3
The len
function is used a lot, especially when you need to scan the different items in a list with a loop, as you will see in the next chapter!
Try it for Yourself
Take a more in-depth approach to working with lists in the following exercise.
The solution is right here.
Use Dictionaries
Now, let's come back to our problem with the bank's customer names and associated accounts. With the above method, you would need two lists - a list of customer names and a list of account balances. Each time a new person is added to our data, their name and bank account balance would be added to the corresponding lists.
Dictionaries are another type of object, similar to lists, but which will let you do this with a single variable! Indeed, a dictionary is a list of items organized via a system of keys. With a real dictionary, you look up a word to access its definition. In programming, this word corresponds to the key and the definition to the value associated with it. This is called a key-value pair. So, we could have:
Marion Weaver | Alberto Mendoza | Katharine Tyler | Isaac Steele |
10000 | 150 | 300 | 1800.74 |
Here, the first row represents the keys and the second row represents the corresponding values.
Each key in a dictionary must be unique. Strings are generally used to define keys, but this is not a requirement, per se.
Declare a Dictionary
Lists and dictionaries are declared in a similar way, except that a dictionary uses curly brackets instead of square brackets, and key-value pairs must be declared:
accounts = {'Marion Weaver': 10000, 'Alberto Mendoza': 150, 'Katharine Tyler': 300, 'Isaac Steele': 1800.74}
print(accounts['Alberto Mendoza']) # -> 150
The last line will print the value associated with the key "Alberto Mendoza" which is 150.
Manipulate the Items of a Dictionary
Here are the operations frequently carried out with dictionaries:
Access the value of an item
Add a new item (a new key-value pair)
Delete an item via its key
A value can be accessed or modified using the same notation as with lists. With dictionaries, unlike lists, this notation even lets you add items.
Let's see this in action in the following example:
accounts['Marion Weaver'] -= 2000 # I subtract 2000 from David's account
accounts['Kristian Roach'] = 1000 # I add a new individual in my dictionary
print(accounts['Kristian Roach']) # I print the value of Kristian's account
Finally, you can delete an item via the pop()
method by specifying the key of the item you want to delete.
accounts.pop('Alberto Mendoza') # deletes Alberto Mendoza from our dictionary
Finally, in the same way as with lists, you can use the len()
function to see how your dictionary grows in size:
len(accounts) # -> 4
Understand Immutable Tuples
The last type of collection we will look at are tuples. These are very similar to lists:
They are ordered objects, so we can access the different items stored in a tuple from their index.
You can store any kind of object in a tuple.
The main difference is that once a tuple has been declared, it cannot be modified. It is then said that it is immutable.
We can't modify a tuple? So what's the point of it?!
They might not seem to provide much benefit at first sight, but they can be used:
when you want to make sure that data is not modified within a program.
to return several values from a function. Indeed, we didn't address this point when we talked about functions, but it is possible to return several values... with a tuple!
to declare several variables in one line.
Declare a Tuple
Tuples are declared in a very similar way to lists, except parentheses are used instead of square brackets:
my_tuple = (1, 2, 3, 'a', 'b')
Manipulate Tuples
As explained above, tuples are ordered objects, so we can use indices to select the items of a tuple:
print(my_tuple[1]) # -> 2
print(my_tuple[4]) # -> 'b'
You can also declare several variables at the same time from a tuple:
a, b = (1, 'apple')
print(a) # -> 1
print(b) # -> 'apple'
However, you will get an error if you try to modify your tuple in any way, as you can see below.
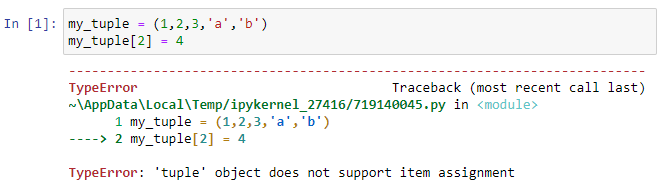
Let's Recap
In this chapter, you have learned all the basics of using Python’s different built-in "collection types":
Lists: an ordered, editable collection where each item is associated with an index
Dictionaries: an unordered, editable collection where each item is associated with a key
Tuples: an ordered, non-mutable collection , where each item is associated with an index
The most common actions performed with lists and dictionaries are:
Access an item
Add an item
Delete an item
Modify an item
Count the number of items stored
We can perform these different actions via methods.
The type to use depends on the task at hand. As you progress in your career, you will be able to better identify the structure that is best suited to your situation!
We will now see how to organize our code via conditional structures.