You've probably heard the term object in a programming context before. But what does it really mean? Let's start by looking at some real-world objects, like pens, books, smartphones, computers, etc.
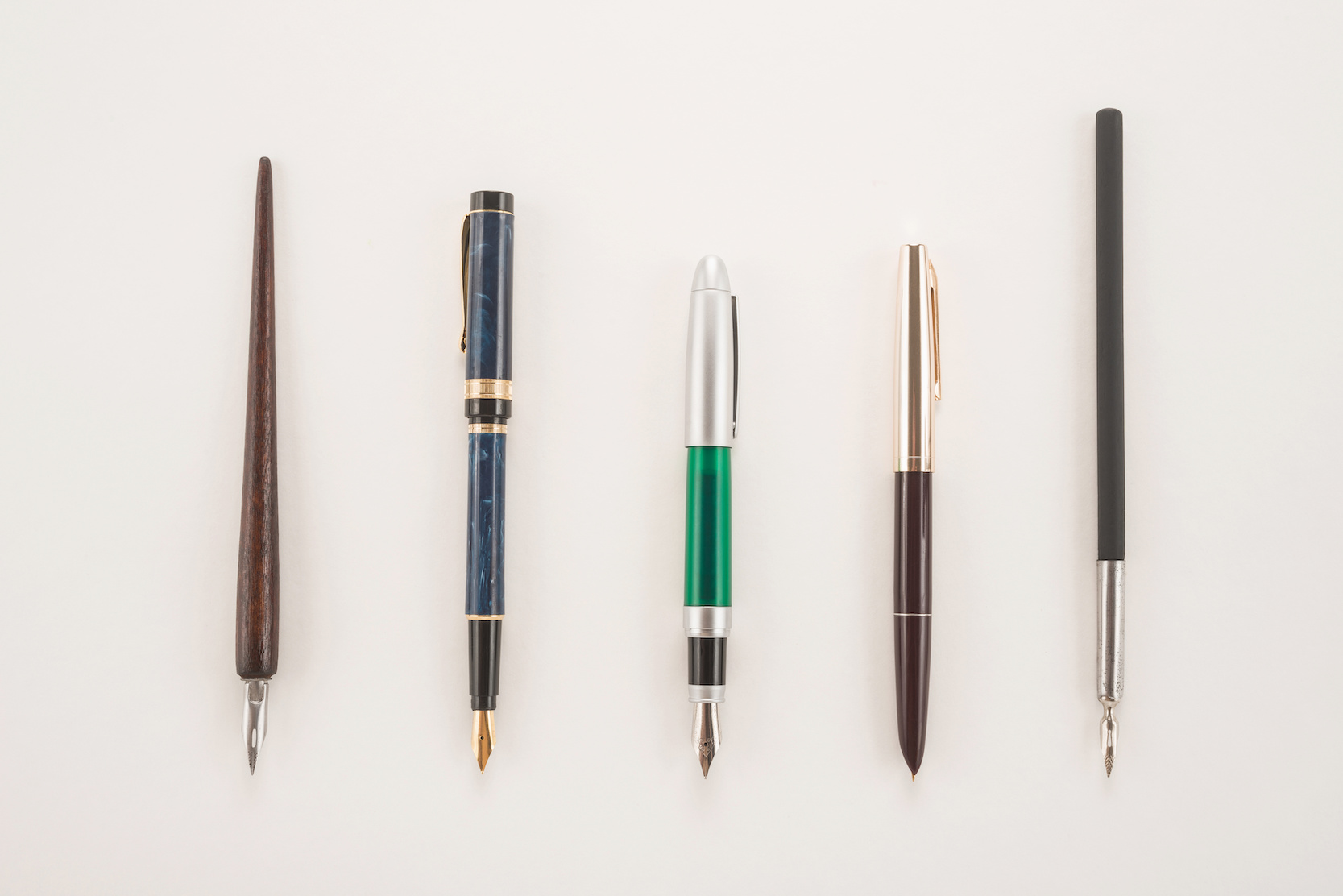
You recognize all pens — fountain, ballpoint, felt-tip, gel, and so on — as being part of a type of object: pens. You can write with them, they use ink, and they can be held in one hand.
The same goes for books: they have a cover, a number of pages, a title, and one or more authors.
You notice commonalities between different objects, collect the information, and create a mental representation for a category of objects.
This mental list of attributes acts as a blueprint for that object. In programming, it's called a class. When creating a class, you can come up with any custom name, which is why they are called a named type. As you'll see, they also allow you to group lots of details together, which is why they can be referred to as complex types.
Before diving into classes, let's have a look at a complex JavaScript type: the object.
Objects
JavaScript objects are written in JavaScript Object Notation (JSON) — a series of comma-separated key/value pairs between curly braces, which you can store in a variable:
let myBook = {
title: 'The Story of Tau',
author: 'Will Alexander',
numberOfPages: 250,
isAvailable: true
};
Each key is a string, and the associated values can be of any data type.
The major advantage of being able to build objects is that you can group the attributes of a single thing in one place, be it a book, a user profile, or the configuration for an app, for example.
Practice building an Object!
Let's revisit a previous exercise, and build a single object variable instead of three separate ones. Head to CodePen Exercise P1CH4a and follow the instructions below.
In a previous exercise, we created three variables for an episode's title, duration, and whether or not the user had already watched the episode. Doesn't that sound like a situation where a single episode object would make more sense? Let's build it!
Create an Object and store it in a variable called episode, using curly-brace notation (JSON), with three keys:
title
duration
hasBeenWatched
Associate appropriate values to each of these keys.
Remember that the syntax for a key value pair is: key: value, and they are separated by commas.
If you didn't get it right first time, don't worry! That's what programming is about. Keep trying until you're comfortable with the notation. 😎
Solution:
Once you've given it a go, watch me code a solution and see how your approach compares. And here is a new CodePen with a solution to the exercice.
Accessing an Object's data
Now that you know how to create an object in JavaScript, let's look at how to access the data within an object with dot notation:
Once you have an object stored in a variable, you can access its data like so:
let myBook = {
title: 'The Story of Tau',
author: 'Will Alexander',
numberOfPages: 250,
isAvailable: true
};
let bookTitle = myBook.title; // 'The Story of Tau'
let bookPages = myBook.numberOfPages // 250
Use the name of the variable containing the object, a dot (.
), and then the name of the key for which you wish to retrieve the value.
Practice retrieving values from an Object!
Head to CodePen Exercise P1CH4b and follow the instructions below to practice using dot notation to retrieve values from an Object.
Let's look at the previous exercise from the other side! When you built the episode Object variable in the last exercise, your colleague's code extracted the data from it and displayed it in the episode component. Now it's your turn to extract the data to make it available to the same component using dot notation.
This is exactly how the component extracted data in the previous exercise! 😉
Create the three following variables:
episodeTitle
episodeDuration
episodeHasBeenWatched
Assign the appropriate values from the episode Object to each variable, using dot notation.
Solution:
Once you've given it a go, watch me code a solution and see how your approach compares. And here is a new CodePen with a solution to the exercice.
Classes
Building an object by hand using curly-brace notation is fine for simple, single objects. However, you will often need many objects of the same type. This is where classes come in handy.
As mentioned earlier, a class is a blueprint for an object in code. It allows you to build multiple objects of the same type (called instances of the same class) more quickly, easily, and reliably.
Let's have a look at how you build a class in code:
To create a class in JavaScript, use the class
keyword, followed by a name. Then enclose the class code in curly braces:
class Book {
}
For this class, we would like every Book
to have a title
, an author
, and number of pages
. To do this, you use what is called a constructor:
class Book {
constructor(title, author, pages) {
}
}
There is a set of instructions to follow within the constructor
when you create a new instance of the Book
class. To assign the title, author and number of pages you receive to this instance, use the this
keyword and dot notation:
class Book {
constructor(title, author, pages) {
this.title = title;
this.author = author;
this.pages = pages;
}
}
Here, the this
keyword refers to the new instance. Therefore, it is using dot notation to assign the received values to its corresponding keys.
Now that the class is complete, you can create new instances of it using the new
keyword:
let myBook = new Book('The Story of Tau', 'Will Alexander', 250);
This line creates the following object:
{
title: 'The Story of Tau',
author: 'Will Alexander',
pages: 250
}
Having a Book
class allows you to create new Book
objects quickly and easily.
Right! Now it's your turn. The best way to learn about classes is to make one yourself.
Practice using classes!
Head to CodePen Exercise P1CH4c and follow the instructions below.
Now that you know about classes, it's time to use one for our episode component. This time, there are three episode components, so the logical way of doing things is to create an Episode
class, and then three instances of it — one for each episode.
Create a class called Episode using the class keyword.
Create a constructor for the Episode class. It should accept three arguments:
title
duration
hasBeenWatched
Using the this
keyword, make sure the constructor assigns the received arguments to the corresponding fields in the new instance. Using the new
keyword, create three instances of the Episode
and store them in the variables:
firstEpisode
secondEpisode
thirdEpisode
Remember to pass appropriate arguments to each!
Solution:
Once you've given it a go, watch me code a solution and see how your approach compares. And here is a new CodePen with a solution to the exercice.
Let's recap!
We've covered a lot of ground in this chapter!
Objects — key/value pairs in JSON notation which allow you to store multiple pieces of related data in one variable.
Dot notation — accessing and modifying the values in an object.
Classes — how using classes can allow you to create objects more easily, and in a more readable way.
Next up, you're going to see how to keep multiple variables grouped together with collections.