You've taken tests at school, for your health, or to get your driver's license. Tests confirm that something functions, whether it's your knowledge or anything else.
Tests in programming are no different, except they test that your code functions! ✅
They'll save you a major amount of development time and headaches from accidentally breaking features you didn't mean to touch.
Writing tests for code
Testing exists in nearly every programming language, though each developer's approach to testing can be very different.
Ultimately, should you write tests? Yes, absolutely! 👍
Many programmers just want to build a website that works. They test it by clicking around. If things do what they're supposed to do, that's deemed sufficient.
Who cares about testing your code when you can just test the website?
Imagine you're coding a new functionality that slightly changes a crucial part of your website, such as the ability upload a photo as part of a user's profile. 👥
Contrary to what we may think, simply being able to upload a photo isn't enough.
How do you confirm that an image over a certain size won't work? How can you be sure that the photo is associated with the correct user? How can you know that, if you change accepted image formats later, that it doesn't break all the existing profile photos?
You could waste your time trying these things manually each time you make a code change. Clicking around your website with a shoot-in-the-dark approach for identifying bugs is not scalable.
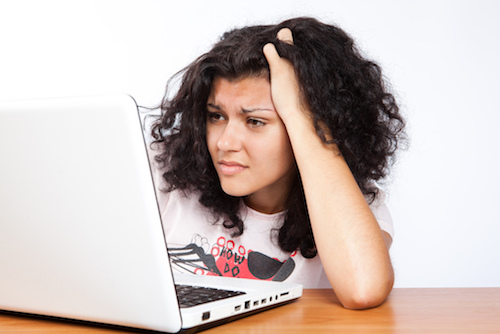
Protect your code
Protect your code from future changes that may unwittingly break it, whether it's written by one of your colleagues or future you!
If you write tests, you'll be able to run the tests after changing your code and instantly see if the code still behaves as expected. If you've got a failing test, you know your code doesn't work (or you've written a bad test! Either way, something needs fixed).
What can you test?
Any situation is testable, even simple ones. Let's say we're talking about the phenomenon of adding puppies to a basket (why not?).
You'd want to confirm that adding a puppy to a basket results in an additional puppy in the basket. It sounds deceptively simple, but In its most basic, non-language-specific form, you'd write out your test spec as follows:
Given there are 2 puppies in a basket
When I add another puppy to the basket
Then the number of puppies in the basket should be 3
If you're a front-end developer, you might write a test for the equivalent JavaScript code that looks like this:
describe("Puppies in a basket", function() {
describe("when adding 1 puppy to a basket with 2 puppies", function() {
var puppies = ['fido', 'spot'];
puppies.push('bowzer');
it("should change the puppy quantity to 3", function() {
expect(puppies.length).toEqual(3);
});
});
});
There are programs that will run this test, and the test will either pass or fail. Imagine a test program as a machine that automatically scans those slips of paper on which you bubble in A, B, C, or D on a test in school.

Now that you understand what a code test is conceptually, let's look at several different philosophies behind writing them!