How do you build a program? Just like a building—brick by brick and step by step!
The first stage involves breaking the main problem down into subproblems. Imagine you want to build a LEGO castle. You’ve got all the pieces you need, but you don’t know how to go about assembling them. Rather than building the whole thing in one go, which would be impossible, you decide to start with one small part. Maybe you decide to build the foundations first, or the windows, or even the roof. Whichever you choose to start with, you break the building process down into several smaller stages.
This is exactly what we’re going to do in this chapter, by thinking about the different rules that apply to a maze.
Find out the Rules of the Program
These are the rules of our program:
The player begins the game on “Start” and needs to get to “End.”
The player can only move in four directions—up, down, right, and left.
The player must pick up objects as they move through the maze to be able to exit it.
The player can choose from two options—an unlimited number of moves (easy mode), or a limited number of moves (hard mode).
Let’s forget computers for a moment, and think about how we could put this game together. An algorithm is only a solution to a problem, so for the moment we don’t need our AI friends.
Break a Problem Down Into Subproblems
What operations can the player perform?
Let’s make a list:
Manage their moves (up, down, right, and left)
Save the number of moves
Count the number of moves (an unlimited or limited number of moves)
Store objects they’ve picked up
Great, that gives us a better idea of what we need to do!
Describe an Algorithm With Pseudocode
Now we’ve broken our problem down, how can we describe the algorithms we need to solve these subproblems?
This is where pseudocode comes in. Pseudocode is an informal way of describing a program, which doesn’t require any strict programming language syntax or any technological considerations. It’s used to create a draft of a program.
All throughout the course, you’ll see how to describe each new programming concept using pseudocode. For the moment, let’s start with a simple example of pseudocode to describe the player’s moves. This will help you get used to the syntax.
Here’s the pseudocode describing the player’s moves from the start to the end:
Moves algorithm
Start
Move right
Move up
Move right
Move up
End
How should we read this pseudocode?
The name of the algorithm is followed by the word “algorithm.”
Two keywords are used to show the start and end of the algorithm:
Start
andEnd
.The instructions of the algorithm are in between the keywords
Start
andEnd
.
Over to You!
Context
As you go through the different chapters of this course, you’ll be developing programs to allow the player to take certain actions within the maze. At this stage, the objective is to allow the player to move from the start to the end.
Here’s the maze:
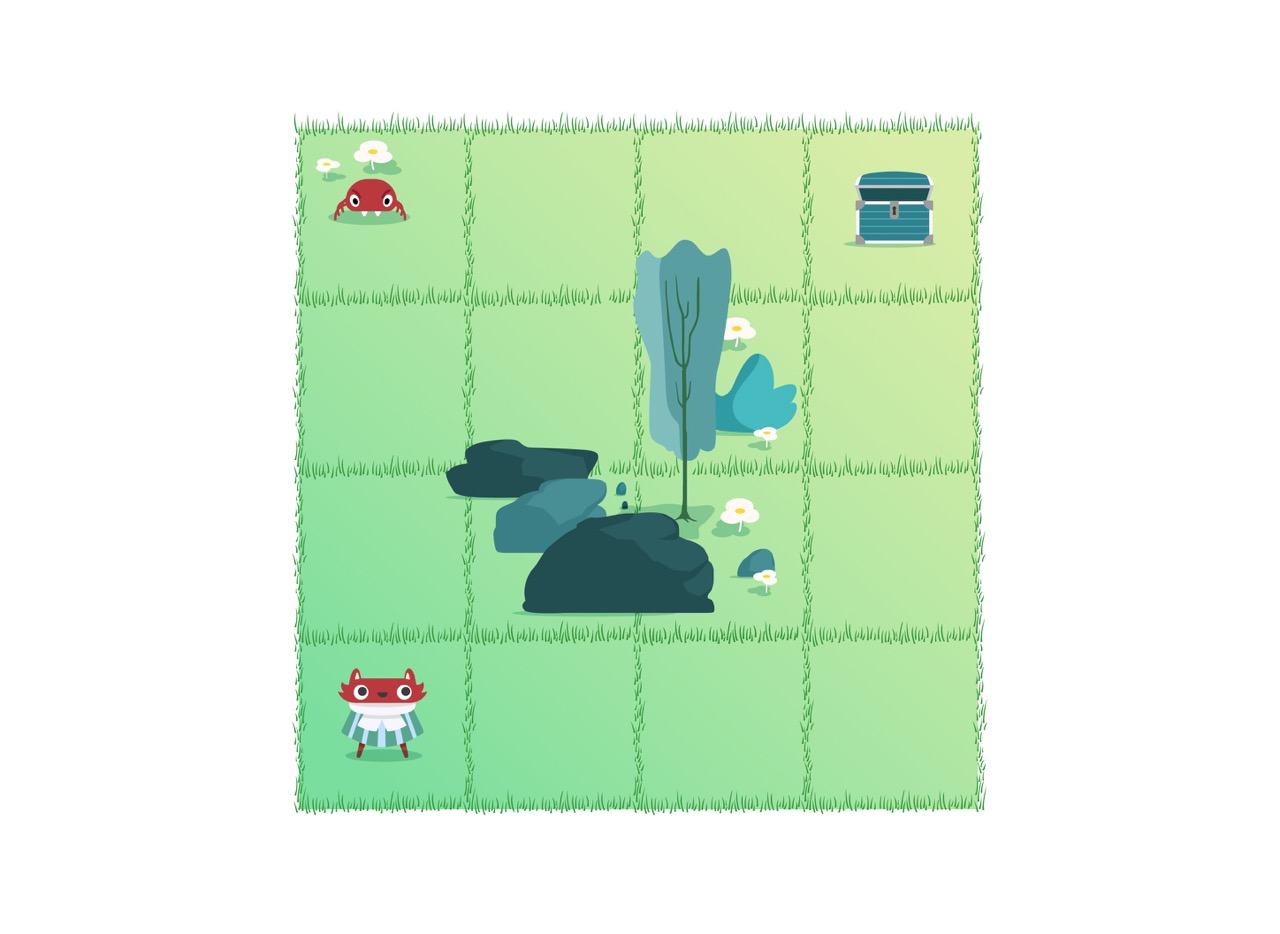
Instructions
You can use the following instructions in your program to describe the moves:
Move right
Move left
Move up
Move down
Try writing the two possible algorithms to describe the route from start to end!
Check Your Work
Here are the two possible solutions:
Solution 1:
Solution 1 algorithm
Start
Move right
Move right
Move right
Move up
Move up
Move up
End
Solution 2:
Solution 2 algorithm
Start
Move up
Move up
Move right
Move up
Move right
Move right
End
Let’s Recap!
Break a complex problem down into subproblems which are simpler to solve.
Use pseudocode to describe your program.
Pseudocode is simply a high-level description of an algorithm in the form of annotations and informative text, written in clear language. This means it is NOT yet actual code syntax.
Pseudocode instructions are contained within the keywords
Start
andEnd
.
How can we communicate with a computer?
Using a programming language, of course! Go to the next chapter to find out how.