This morning, as usual, you got your delightful cappuccino to start your day. You also had a quick chat with Jenny, and she shared an idea with you about how she could perhaps collect the necessary amount a bit faster. She met with an owner of a new bakery who's testing out new baked goods and is offering them at a lower price for a period of time to small cafes, like Jenny's.
This sweet and savoury dessert is called 'Munchy cruller'. The bakery offers them at a cost of $2.50 each for a 30 day trial period. Jenny can invest the $10 that she allocates for savings each day and sell them for $5.50 to make some profit and also to see if her customers like the new item and would pay this price.
Hm.. .this is interesting information! Why not to try it out for 30 days?! But first, we need to calculate the potential benefits!
In addition, we could reinvest daily profit and buy more pastries, compounding the profit! :zorro: Hold your horses! One step at a time :pirate:!
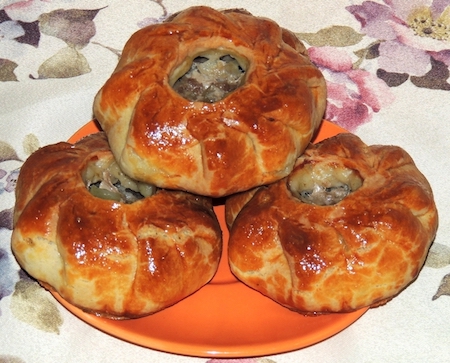
Let's get on it!
Print function and console
Print is an assisting function of Swift that helps developers make sure the code is functioning the way that they intend and that it is operating with the expected data. In other words, most of the time certain variables have expected (and correct) values. This function outputs data in the Xcode console, which is the bottom panel of your project window.
The fastest way to get the hang of it is to test it out in Playground. Let's go ahead and try the following examples:
print("Hello!")
And we can observe the output on the Console panel (as well as the Output panel - the one on the right):

Let's experiment a bit more, but using variables this time:
var x = 3
print(x) // 3
print(54) // 54
print("Hey there!") // "Hey there!"
"For-in" loop
To help Jenny with the investment prediction, we need to learn a new concept - loops!
A loop, in programming, is a technique that allows us to repeat one or more instructions without having to retype the same set of instructions multiple times.
For example, if we want make 5 cappuccinos we could use following code:
Make cappuccino.
Make cappuccino.
Make cappuccino.
Make cappuccino.
Make cappuccino.
And what if I need to make a thousand?! It would be pretty tedious. This is where loops come to the rescue. Using a loop, we can alter our code:
Repeat 5 times:
Make cappuccino.
And now we have 5 cappuccinos made one after another (remember, Jenny only has one coffee making station.. for now ;)). Much better, right?
So, how do we implement this with Swift? - Using the for-in
instruction:
for i in 1...5 {
print("Make cappuccino.")
}
You can observe in the console the text "Make cappuccino." printed 5 times.
So let's take a closer look at how it works exactly:
The program creates a variable named
i
of typeInt
and initializes it with the value1
:var i = 1
Then it performs the instruction between the curly brackets {}:
print ("Make cappuccino.")
Then, increases
i
by 1, so it now holds value2
:i = 2
It then performs the same instruction again:
print ("Make cappuccino.")
i
receives the value3
. The same instruction gets executed.i
receives the value4
. The same instruction gets executed.i
receives the value5
. The same instruction gets executed.
And how does the program know whether to increase the value of i
and continue the loop or stop?
At each iteration, after completing the instruction the value of i
increases no matter what. Then, before proceeding to execute the instructions within the loop, its new value is compared to 5 (in our case). If it's not greater than 5 yet, it moves on to the instructions. Alternatively, once it is, it finishes the loop and moves on to the code that follows the loop (we don't have any at the moment).
Get the idea?! To clarify it further, let's display the value of i
at every iteration:
for i in 1...5 {
print("Make cappuccino.")
print("This is a cup #\(i).")
}
You should see this in the console:
Make cappuccino. This is a cup #1. Make cappuccino. This is a cup #2. Make cappuccino. This is a cup #3. Make cappuccino. This is a cup #4. Make cappuccino. This is a cup #5.
Here is a diagram to illustrate the "for-in" loop:
Range type
We already know a few numeric types and string. Next in line we've got the type: Range. You've already seen them in the examples above, they look like this: 1 ... 5
. We used them in "for-in" loops.
To declare a Range, we specify lower and upper limit with triple dot in between ...
:
var myRange = 1 ... 1000
There are two types of ranges:
Closed range. This type of range includes both ends - lower and upper limits. We've used only this type so far:
for i in 1 ... 3 { print (i) }
This would print the following in the console:
1 2 3
Semi-open ranges. This type of range does not include the upper limit and instead of
...
we must use..<
:for i in 1 ..< 3 { print (i) }
This would print following in the console:
1 2
Let's summarize:
var closedRange = 0 ... 4 // includes values: 0, 1, 2, 3, 4 (4 is also part of it!)
var semiOpenRange = 0 ..< 4 // includes values: 0, 1, 2, 3 (4 is NOT part of it!)
To reinforce this knowledge, let's practice by creating a loop that shows you eating your birthday cake for X years. Specifically, print the following sentence in the console X number of times:
I'm turning X years of age and going to eat this delicious cake to celebrate my birthday!
As a constraint for this exercise, you must use a semi-open range. Try to do it on your own!
// No scrolling!
for age in 1 ..< 101 {
print("I'm turning \(age) years of age and going to eat this delicious cake to celebrate my birthday!")
}
If you are 100 years old (not bad for an iOS developer I must say :p!), you ate your birthday cake 100 times- from 1 to 100.
We could use the open range with the upper limit 100: 1 ... 100
. Open range would include 100 and we would get 100 repetitions - which is what we need.
However as a condition for this exercise we have to use a semi-open range, so the upper limit will not be included. And if we use 100, we will only get 99 repetitions. So, in order to reach 100 using a semi-open range we need to increase the upper limit by 1, resulting into 101 instead of 100: 1 ..< 100
.
What about Jenny's investment plan?
We still have a problem to solve.
[1] Jenny's intending to invest her savings allocation of $10 to purchase new dessert at $2.50 each - as many as she can afford. Then sell them the same day for $5.50. She hopes this will speed up her saving process towards the purchase of a new coffee making station as well as introduce more variety of products to her customers. At a discount price she can test it for 30 days and see if customers like it and willing to pay the price she set.
[2] Here's our initial data:
Daily investment: $10
A new pastry, Munchy cruller, available for purchase: $2.50 each
Sale price for the new pastry: $5.50 each
Investment period: 30 days
Well, how Jenny could benefit from this investment? Let's calculate!
Assume we start with $0 savings in the first day:
var money = 0.0
Try to come up with the logic yourself and implement it using the "for-in" loop!
// Game on!
var money = 0.0
for i in 1 ... 30 {
// Jenny tops up savings money from her daily profit
money = money + 10
// Jenny spends daily allowance to purchase as many pasteries as she can
money = money - 2.50 * 10/2.50
// Jenny sells new pastries to her clients
money = money + 10/2.50 * 5.50
}
print("$\(money)") // $660.0
Here's a breakdown of the logic:
A loop is created to cover 30 days.
Each day we add $10 as savings, which becomes our investment money for the day.
We use that daily amount (10) to buy as many pastries as we can (10/2.50 => 4) at $2.50 (- 2.50 * 10/2.50), then we sell the number of purchased pastries at $5.50 and add it to the total savings (+ 10/2.50 * 5.50).
The result is impressive! In 30 days we are able to save $660.0, that's more than a 3d of the needed amount!
Bonus challenge: Identify a problem in the code above. Why won't it work correctly in all cases? Name a case for which it would produce incorrect results.
Here are some tips to improve the code:
Tip #1: Using for-in loop without an enumerating variable
We are declaring a variable i
which is an enumerator for the loop and it holds the value within the specified range at each iteration: 1, 2, 3 etc. and the last value would be 30. However in the context of our task we are not using it. To keep the code clean, we can remove it altogether and replace it with underscore _
:
for _ in 1 ... 30 {
// our instructions
}
Tip #2: Modifying a variable using its current value
Often we need to modify the value of a variable by using its current value as a part of the operation, for example:
money = money + 10
In this line, we are adding 10 to what we currently have stored in the money
variable. The instructions are to take the current value, add 10 to it and put it back under the same name with a new value.
There's a short cut for writing such an instruction:
money += 10
The same shortcut is possible for the rest of the basic operators: subtraction, multiplication and division:
Addition |
|
Subtraction |
|
Multiplication |
|
Division |
|
To practice, declare a variable x
. Assign a value of your choice. Then apply the following modifications:
Multiply by 4
Add 8
Divide by 2
Subtract 4
Divide by 2
Of course, do this using the operators you have just learned!
Applying this new knowledge to our code, it will look like this:
var money = 0.0
for _ in 1 ... 30 {
// Jenny tops up savings money from her daily profit
money += 10
// Jenny spends daily allowance to purchase as many pasteries as she can
money -= 2.50 * 10/2.50
// Jenny sells new pastries to her clients
money += 10/2.50 * 5.50
}
print("$\(money)") // $660.0
Tip #3: Using variables instead of hard coded values
When to use variables:
If a number or a calculation is used more than once. Ex.: we are calculating how many pastries we can afford every day.
If a number may need manual adjustment. Ex.: We may renegotiate with the bakery and extend the trial period. With a variable, we can easily find the number we need to adjust without confusing it with some other number that may happen to have the same value.
Initial data is best kept in one place, and it also may be subject to adjustment. Ex.: Pastry cost and selling price, as well as daily investment allowance.
When to use values:
Some obvious constants that will be true forever (relatively ;)). Ex.: number of days in a week: it will be 7 for a while.
To quickly test out a piece of code while unsure about its final form.
Looks like we can clean up our code even further:
//initial data
var money = 0.0
let trialPeriod = 30
let dailyInvestment = 10.0
let pastryCost = 2.50
let pastryPrice = 5.50
let numberOfPastries = dailyInvestment / pastryCost
for _ in 1 ... trialPeriod {
// Jenny tops up savings money from her daily profit
money += dailyInvestment
// Jenny spends daily allowance to purchase as many pasteries as she can
money -= pastryCost * numberOfPastries
// Jenny sells new pastries to her clients
money += numberOfPastries * pastryPrice
}
print("$\(money)") // $660.0
Let's Recap!
The "for-in" loops allow you to repeat an instruction a number of times. They are created with the following syntax:
for i in 0 ... 10 { // the instructions to be repeated }
The variable
i
above can be omitted by using_
character instead.There are 2 types of intervals/ranges:
Closed range:
0 ... 10
, they include both ends.Semi-open intervals:
0 ..< 10
, they do not include the upper end.
Intervals are used to construct "for-in" loops.
You can modify a variable by adding, subtracting, multiplying, or dividing it by a number using following operators:
+=
-=
*=
/=