Remember, we listed 3 types of collections: Arrays, Dictionaries and Sets. You already know Arrays.
Next in line are dictionaries, which are, as you may guess, the subject of this chapter!
So, let’s refer back to our solution for Jenny using arrays. We’ve got 3 elements that we can access using indices: 0 for coffee, 1 for dessert and 2 for food.
// initial profits for all categories
// [0 - coffee, 1 - dessert, 2 - food]
var profits = [0.0, 0.0, 0.0]
// modifying profit for dessert
profits[1] += 50
It works just fine, except that we always have to remember 2 things:
Which category each element refers to
The order in which we originally arranged them
To increase the profit number for dessert we’ll have to remember to use index 1. It's easy to forget or to simply mistype, using 2 instead. Which, in turn, would increase the results for food, producing false information for Jenny :'(.
Luckily, Dictionaries come to the rescue!
Dictionary data type
A dictionary is a list of elements ordered by a key.
Each element is associated with a given key, instead of a number that it happens to follow in the general order of a list. This is called a KEY <> VALUE association:
[key1: value1, key2: value2]
A dictionary may contain any number of elements, including ZERO: an empty dictionary.
Key type
Keys can be of various types. The most commonly used type is String. Which is handy in our case, because we can replace index 0 with “coffee”, 1 with “dessert”, and, 2 with “food”.
Here's an example of a list of elements organized by key:
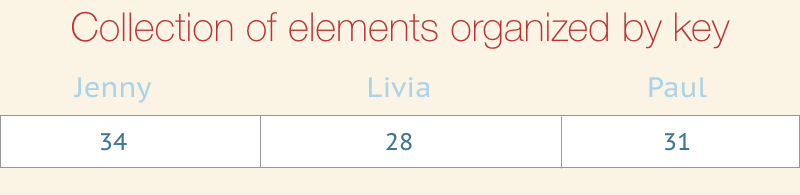
Dictionary syntax
Similarly to arrays, to declare a dictionary we also use square brackets. However, in addition to the element type we need to specify the key type. That's done by adding the key type in front of the element type followed by a colon: [String: Int]
:
// my friends' ages
var ages = ["Jenny": 34, "Livia": 28, "Paul": 31]
All keys in a dictionary must be unique.
While using strings as keys, we must remember that such keys are case sensitive. For example, "Jenny" and "jenny" are two different keys that will associate with two different elements in a dictionary. For example:
var ages = ["Jenny": 34, "Livia": 28, "Paul": 31, "jenny": 21]
print(ages["Jenny"]) // 34
print(ages["jenny"]) // 21
One of the tricks to protect against such situations is using constants to specify the keys once and then reusing them throughout the code:
// define keys as constants
let kJenny = "Jenny"
let kLivia = "Livia"
let kPaul = "Paul"
// use constants as keys
var ages = [kJenny: 34, kLivia: 28, kPaul: 31]
// access an element
print(ages[kJenny]) // 34
Swift will not allow for the mistyping of a constant's name (or a variable), but the contents of strings is solely the developer's responsibility.
Like for simple variable types and arrays, the same rules apply to dictionaries: it always has a type and it cannot change its type.
All elements in a dictionary must be of the same type. And all keys must be of the same type:
var ages = ["Jenny": 34, "Livia": 28, "Paul": 31] // Ok
var mixedInfo = ["Jenny": 34, "Livia": 28, "Paul": "Designer"] // Error
var confusingInfo = ["Jenny": 34, "Livia": 28, 10: 31] // Error
But the the type of keys may be different from the type of elements or may be the same:
var professions = ["Jenny": "Business owner", "Livia": "Developer", "Paul": "Designer"] // [String: String]
var truth = [true: "Truth", false: "Lie"] // [Bool: String]
Working with Dictionaries
Declaration and initialization
We've already experimented with a couple of variants, so now let's sum them up.
Declaring dictionaries by specifying type:
var myDictionary: [String: Int] // declaration by specifying type - short option
var myDictionary: Dictionary<String, Int> // declaration by specifying type - full version
Declaring initializing by assigning a value, with or without specifying a type:
var myDictionary = ["Developers": 5, "Designers": 2] // assigning value and inferring the type
var myDictionary: [String: Int] = ["Developers": 5, "Designers": 2] // assigning value and speficying the type
Declaring and initializing empty dictionaries by assigning an empty dictionary:
var myDictionary = [String: Int]() // assigning a default value of type initialization
var myDictionary: [String: Int] = [:] // assigning an empty dictionary
Mutability
Mutability of dictionaries is very similar to arrays.
Dictionaries declared as variables vs constants, in terms of mutability follow the same rules as simpler data types:
Dictionaries declared using keyword
var
are variable, can change (can mutate), or are simply mutable.Dictionaries declared using keyword
let
are constant, cannot change (cannot mutate), or are simply immutable.
What mutations can we perform on dictionaries?
Here are the frequently used operators we can do with dictionaries:
access each element by its key
add a new element with a new key
remove an element for a specific key
Accessing an element
To access an element we can simply use square brackets and specify key of a desired element ( [key] ):
var myDictionary = ["Developers": 5, "Designers": 2]
print(myDictionary["Developers"]) // 5
Or we can modify an element using its index:
myDictionary["Developers"] += 1
print(myDictionary["Developers"]) // 6
Manipulating elements
To add a new element, we can simply assign a new element using a new key that doesn't exist yet:
myDictionary["Managers"] = 2 // ["Developers": 6, "Designers": 2, "Managers": 2]
To remove an element we can use a removeValue
method:
myDictionary.removeValue(forKey: "Designers") // ["Developers": 6, "Managers": 2]
Counting elements
Dictionaries, like arrays, also have the count
property to to get the number of elements in dictionaries:
print(myDictionary.count) // 2
Browsing elements
Browsing (or walking) a dictionary can be done using for-in loops. Here's an example to present my team. Test it in Playground:
var team = ["Developers": 5, "Designers": 2, "Managers": 2]
for (title, quantity) in team {
print("My team has \(quantity) \(title)")
}
And observe the result in console:
My team has 2 Managers My team has 5 Developers My team has 2 Designers
Practice time! List some countries you wish to visit next and one of the languages spoken there. State how many countries in total you wish to visit and then present the language for each country, one by one, in a sentence like this: "I wish to visit China, people there speak Mandarin. "
You know the drill.. your turn now:
// No scolling just yet!
// initialize the original dictionary
let countries = ["China": "Mandarin", "Croatia": "Croatian", "Switzerland": "Italian"]
// display the total
print("I wish to visit next \(countries.count) countries: ")
// display details about each destination
for (country, language) in countries {
print("I wish to visit \(country), people there speak \(language).")
}
I'm sure you came up with a few lines of valid code!
What about Jenny's challenge?
We still need to improve our profit calculations program. Try optimizing our existing code by using a dictionary instead of an array in order to store profits for the 3 categories. Let's start by declaring a suitable dictionary. Try it on your own:
// Don't look it up!
// profits
var profits = ["coffee": 0.0, "dessert": 0.0, "food": 0.0]
Anything else :euh:?
Not at this time, we'll save further improvements for the next chapter!
Let's Recap!
A dictionary is a list of elements ordered by a key.
All elements in a dictionary must be of the same type.
All keys in a dictionary must be of the same type.
The keys and values of a dictionary can be of the same or of different types.
The types of keys and values cannot change after they've been declared.
Declaring a dictionary type can be done in 2 ways:
By specifing a type in brackets:
[String: Int]
.By letting it be inferred via the assigned elements.
Initializing an empty dictionary can be done in 2 ways:
By assigning an empty array
[:]
if the types of keys and elements are knownBy assigning a default value of an dictionary initialization specifying the keys' and elements' types:
[String: Int]()
.
To access or modify the elements of a dictionary, use the key in brackets:
var myDictionary = ["Developers": 5, "Designers": 2] myDictionary["Developers"] += 1
To add a new element, assign it to a new key:
myDictionary["Managers"] = 2
To delete an element use the
removeValue
method and specify the key:myDictionary.removeValue(forKey: "Designers")
The
count
property is used to get the number of elements in a dictionaryYou can browse the elements of a dictionary using a for-in loop.