Now things have gotten a bit complicated.
We initially calculated how many days Jenny would need to save to be able to purchase a new coffee making station.
Then we added some investment opportunity that will keep going for 30 days. During this time Jenny also tightened her belt and decided to add some extra savings on particular occasions. We then calculated how much she can save during 30 days.
And, she forgot to mention previously that unfortunately on Sundays she will not be able to contribute as the shop is closed one day a week and she is not making any profit. This also means she will be missing the investment opportunity on those days :o.
All this should speed up the saving process when compared to our very first calculation. However, considering all these adjustments, we are no longer able to say either when she will have collected enough funds or how many days she'll expect to wait in order to reach her goal of $1499 .
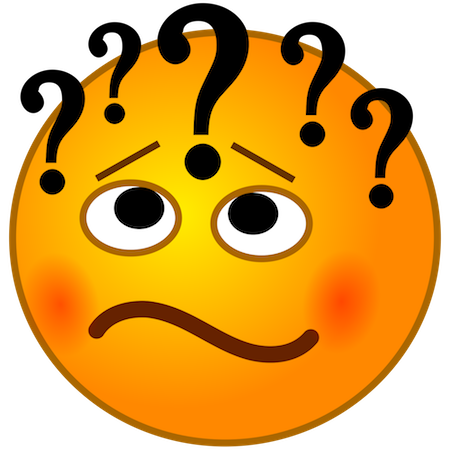
What now :o?
Not too big of a deal as it turns out. Just one more thing to learn: the while
loop!
Introducing the While loop
We can guess by the name, the loop needs to keep going while ... something... Or 'as long as'. It's a somewhat combination of a for-in loop and if statement: it can repeat instructions a number of times like a for-in loop and incorporate a condition like an if statement. The number of repetitions, however, is defined not by a range like in a for-in loop, but by a condition like that of an if statement. Here's what the syntax looks like:
while logicalExpression {
// instructions
}
It can be interpreted as "As long as the logical expression is true, repeat the instructions".
This is how it works in detail:
The program verifies that
logicalExpression
is trueIf it is false: the instructions in brackets
{}
are ignored. We move on along to the next program.If it is true: the instructions in brackets
{}
are executed.Once the instructions are executed, we return to point 1.
Let's take an example to try:
var numberCappuccinos = 0
while numberCappuccinos < 10 {
numberCappuccinos += 1
print("I had \(numberCappuccinos) cappuccinos")
}
print("I can't sleep now!")
Copy this to your Playground. The console displays following:
I had 1 cappuccinos I had 2 cappuccinos I had 3 cappuccinos I had 4 cappuccinos I had 5 cappuccinos I had 6 cappuccinos I had 7 cappuccinos I had 8 cappuccinos I had 9 cappuccinos I had 10 cappuccinos I can't sleep now!
At each loop turnnumberCappuccinos
is incremented by 1. When the variable reaches the value 10
. The numberCappuccinos < 10
is no longer true. So we terminate the loop and we go on with the rest of the code in the program. In this case, it prints "I can't sleep now!".
Nothing's better than practice! Using a while loop, write a program that displays the following:
We are in 1950. I was not born!
We are in 1951. I was not born!
.
.
.
We are in 1991. I was not born!
We are in 1992. I was born!
Go ahead!
// Don't scroll yet! Try on your own...
var year = 1950
var dateOfBirth = 1992
while year < dateOfBirth {
print("We are in \(year). I wasn't born yet!")
year += 1
}
print("We are in \(year). I was born!")
Once more, well done! Congrats!
That's all for the while loop!
Here is a diagram to illustrate the while loop:
What's in it for Jenny?
We are now able to tell her how many days it will take to accumulate the needed funds! We'll do it by replacing our main for-in loop with the while loop. A pseudocode would look something like this:
While the money is less than $1499:
Add a day
Process cashflow operations - saving, expenses, investments.
Display the results
It's your turn to experiment!
// Try !
//initial data
let goal = 1499.0
var money = 0.0
let trialPeriod = 30
let dailyInvestment = 10.0
let pastryCost = 2.50
let pastryPrice = 5.50
let numberOfPastries = dailyInvestment / pastryCost
var day = 1
while money < goal {
if day <= trialPeriod {
// Jenny tops up savings money from her daily profit
if day == 1 {
money += dailyInvestment * 2
} else if day == 10 || day == 20 {
money += dailyInvestment * 3
} else {
money += dailyInvestment
}
// Jenny spends daily allowance to purchase as many pasteries as she can
money -= pastryCost * numberOfPastries
// Jenny sells new pastries to her clients
money += numberOfPastries * pastryPrice
}
else {
money += dailyInvestment
}
day += 1
}
print("It will take \(day - 1) days to save $\(money)") // $1500.0
This will give us the result:
It will take 109 days to save $1500.0
Let's review what we've done here:
Added the
goal
variableReplaced the for-in loop with the while loop
Added the
day
variable (since we removed the for-in loop that provided the day variable)Added an if/else statement to separate the logic for the days during the bakery trial period from the rest of the time needed.
It's starting to looking good; we are getting closer to the resolution. The only thing we haven't addressed is Jenny's day off. It's every Sunday or, to simplify the condition, every 7th day of the week.
And how do we figure that out?
Remainder operator can assist!
Remainder operator
The remainder operator is only applicable to numbers of the type Int
, and is defined using %
. It allows us to calculate a reminder when dividing two integer numbers.
To better demonstrate its functionality, let's refer to Euclidean division.
Here's an example:
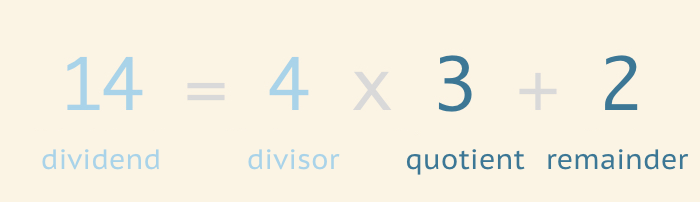
Test it out by typing 14/4
in your playground project: you'll get 3. The whole division in Swift produces a quotient.
Now, type 14%4
. You'll get 2. The remainder operator gives - the remainder!
If you are not very comfortable with the Euclidean division, try to find the quotient for the rest of the following Euclidean divisions:
3 by 2
14 by 7
65 by 11
Use the Playground to verify.
Now, practice by implementing a snippet of code:
Over the period of 30 days, depending on the day of the week, display following statements:
If the day falls on a weekend - Saturday or Sunday: "Great weekend getaway!"
Week day: "Go to work! Exciting projects are waiting!"
Assume the 1st day of our 30 day period falls on a Monday.
Get going!
// No cheating!
//while loop
var day = 0 // 0 corresponds to Monday
while day < 30 {
if day % 7 == 5 || day % 7 == 6 {
// the 6th and the 7th days of the week
print("Great weekend getaway!")
} else {
print("Go to work! Exciting projects are waiting!")
}
day += 1
}
//for-in loop
for day in 0 ..< 30 {
if day % 7 == 5 || day % 7 == 6 {
// the 6th and the 7th days of the week
print("Great weekend getaway!")
} else {
print("Go to work! Exciting projects are waiting!")
}
}
It wasn't a trivial task. Let's look into details (we'll review only the while loop example, because as you will see, for-in is very similar):
We start by defining a 30 day loop:
var day = 0 // 0 corresponds to Monday
while day < 30 {
day += 1
}
Then, we need to evaluate each day to decide what statement to print:
if day % 7 == 5 || day % 7 == 6 {
Here's where the numbers 5 and 7 come from. Considering 0 corresponds to Monday, the following table illustrates the numbers associated with each day of the week:
0 | 1 | 2 | 3 | 4 | 5 | 6 |
Monday | Tuesday | Wednesday | Thursday | Friday | Saturday | Sunday |
For the first week we could compare the variable day
directly with the numbers associated with days of the week. This applies to the first week. All the following weeks, however, will fail to produce the correct results. Let's take Saturdays as an example; they fall on days 12, 19 and 26.
If we do the Euclidean division of 5, 12, 19 and 26 by 7 we'll have:
5 = 7 x 0 + 5
12 = 7 x 1 + 5
19 = 7 x 2 + 5
26 = 7 x 3 + 5
The remainder is always 5! In other words using the remainder operator will ensure we always produce correct results: day % 7 == 5
will be true every Saturday. And similarly, for Sundays: day % 7 == 6
.
Jenny's waiting!
Using the remainder operator will help us isolate Sundays during the whole saving period and exclude those days:
// Experiment!
// initial data
let goal = 1499.0
var money = 0.0
let trialPeriod = 30
let dailyInvestment = 10.0
let pastryCost = 2.50
let pastryPrice = 5.50
let numberOfPastries = dailyInvestment / pastryCost
var day = 1
while money < goal {
if day % 7 != 6 {
if day <= trialPeriod {
// Jenny tops up savings money from her daily profit
if day == 1 {
money += dailyInvestment * 2
} else if day == 10 || day == 20 {
money += dailyInvestment * 3
} else {
money += dailyInvestment
}
// Jenny spends daily allowance to purchase as many pasteries as she can
money -= pastryCost * numberOfPastries
// Jenny sells new pastries to her clients
money += numberOfPastries * pastryPrice
}
else {
money += dailyInvestment
}
}
day += 1
}
print("It will take \(day - 1) days to save $\(money)") // $1502.0
And the result:
It will take 135 days to save $1502.0
All we did is add another else/if statement in order to evaluate the day of the week.
Strange, we simply excluded a couple days. Shouldn't it affect only the number of day and not the total amount of accumulated funds (money)?
This would be logical to conclude. If we look closer, though, we'll notice that one of the Sundays falls on day 20, which was when Jenny planned to do an extra contribution to her savings. Here, we're losing some extra savings. This, in fact, is responsible for the altered final amount of funds.
Well done! Even though, while helping Jenny to develop her saving strategy, we could offer her a faster saving strategy, it's important that she also accounts for her lifestyle!
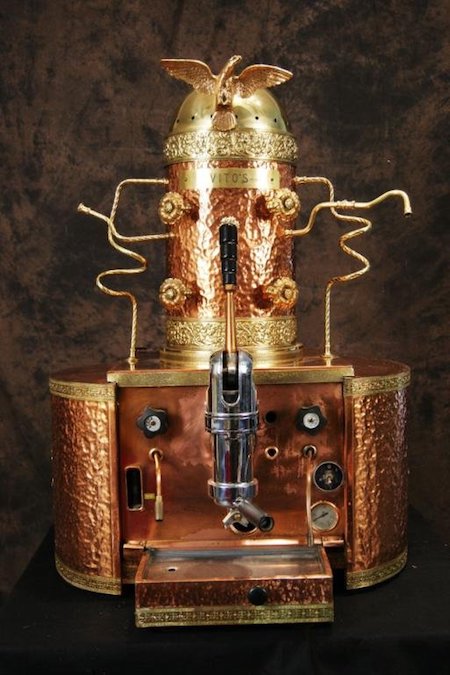
Summarizing the control flow
Let's step back and take a look at the whole composition of control flow in a program.
We have learned about 3 elements of control flow:
Two loops:
for-in - used to repeat instructions a specified number of times
while - used to repeat instructions until specified condition is met
if / else if / else statement - used to redirect the flow according to a specified condition
Imagine your program as a river and these three control elements influence its course:
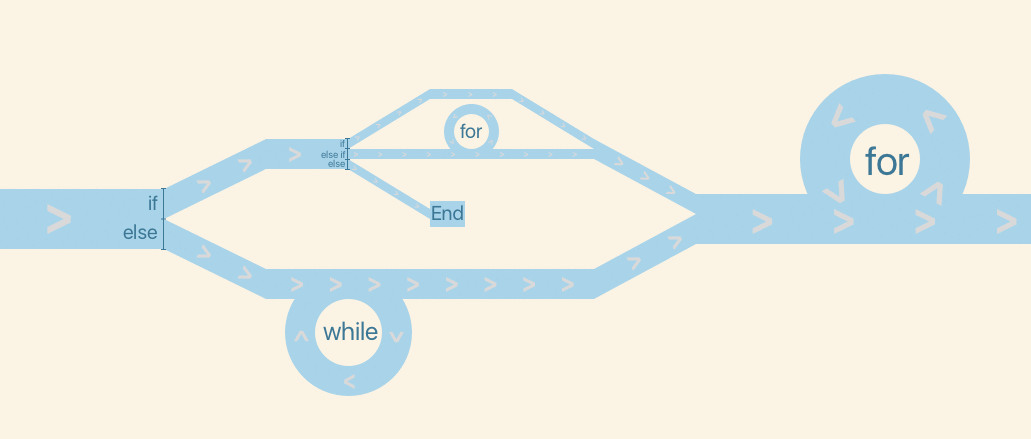
Let's Recap!
We have discovered the while loop which allows for repeating instructions as long as a specified condition is true:
while condition { // instructions }
We learned about a new operator: remainder
%
It makes it possible to obtain the remainder of a Euclidean division
By definition, it is only applicable to numbers of type
Int
We now know 3 control elements out of the 4 that exist in Swift:
For-in and while loops
if / else if / else statement