What is a variable?
The goal of a program is to do something with data, or in other words, stuff you put into your program. Your program uses a variable to manipulate the data. More specifically, it is a container used to store a piece of data that your program may need for its processing.
As mentioned in the video, think of a variable as a box that contains a value. This box is stored on a shelf within a gigantic warehouse. The location of every box in the warehouse is carefully recorded, just like your computer records the location of your variable in memory.
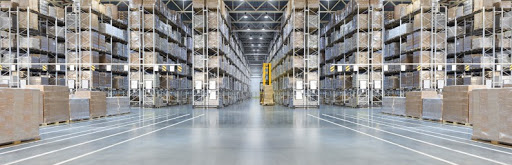
A piece of data placed in a variable is called a value. Using the box analogy, different boxes can store different values. For example, you can use a box to store money for ongoing expenditures and another to save up for a specific occasion, like going on a trip. You can also empty the boxes or change their content, like adding some money or taking some out.
To know what each box is for, you need to label them. With programming, it's the same: you assign a name to your variable. 😉
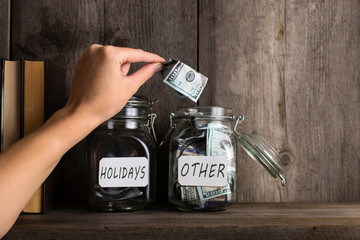
A variable's name should reflect the meaning of its contents like labels on boxes. Here are some general recommendations for creating names:
Use descriptive names throughout your code: It may get a bit lengthy! However, descriptive names will benefit you and your team in the long run because they provide better readability and make code maintenance easier. For example, if you want to store gluten free cookies, using a descriptive name such as
glutenFreeCookies
is much more comprehensive than, say, justcookies
or evenhealthyCookies
.Spell it out: Avoid abbreviation or shortening words when possible, even if a shorter version seems obvious. For example,
annualRevenue
is better thanannualRev
.Follow a common naming convention: One of the popular naming conventions is called camel case (also known as camel caps) - a compound phrase that consists of multiple words without spaces or punctuation. The first word is written in lowercase, and all other words are capitalized. For example,
myBudget
.
Create a variable with a variable declaration
To use a variable in code, you must create one. It's called making a declaration.
There are several types of variables in C#, and you use them according to the type of value they hold. In C#, variables containing numbers are declared by using a keyword such as int
followed by the name of a variable and its initial value. For example:
int ongoingAllowance = 500;
int savings = 1000;
Here we've declared two variables: ongoingAllowance
and savings
. They store values of 500 and 1000 respectively. Note that here, along with the declaration, we've also assigned the initial values to the variables.
Try it out for yourself!
Ready to get started? To access the exercise, click this link.
Change your variable's value with operators
As its name suggests, a variable may vary, that is, change its value. You can do this by performing a number of operations. Consider the variables ongoingAllowance
and savings
from the previous example. You could:
Add more money to savings.
Subtract some from your ongoing allowance.
Find out how long it will take to reach $5K if you save $500 every month.
Find out how much your spending allowance will be if you keep contributing $30 to it everyday for a week.
Find out how much your spending allowance will be if you also spend $10 daily.
Real world problems with programming solutions! 💻 Each operation works by applying arithmetic operators, which are:
+
addition-
subtraction*
multiplication/
division
Regular arithmetic rules apply! This includes the order of execution! Just like in math, you can use parentheses to decide what happens when. Let's see how you can accomplish your goal in C#:
// add 100 to our current savings (Yeah!)
savings = savings + 100;
// remove 50 from out ongoing allowance (Snif)
ongoingAllowance = ongoingAllowance - 50;
// update the number of days we need to save
int numberOfDaysToSave = (5000 - ongoingAllowance) / 500;
// update our ongoing allowance (again)
ongoingAllowance = ongoingAllowance + (30 - 10) * 7;
Look at that lovely block of code! Notice that the code doesn't all look the same. That's because it's composed of comments and statements:
Lines that start with
//
are comments. They are used to help another human understand the code.Lines of code that actually do things are called statements. As the name implies, each statement tells the computer to do something.
Here, each statement assigns a value to a variable. The assignment operator is =
.
On the right of the assignment operator, you write an expression. This is a statement that produces a value.
On the left of the assignment operator, you write the name of the variable you want the result to correspond to.
Let's reiterate. To assign a value to a variable, you write a statement. This statement consists of the name of the variable, followed by the assignment operator, and finally the expression that produces a value matching the type of the variable.
Write shorter code with shortcut assignment operators
You can also directly provide a value on the right of the assignment operator.
// replace the whole savings variable with the new amount
savings = 10000;
By the way, when you need to change the value of a variable with basic operators and assign it back to that variable, you can use a shorter version! For example, instead of using savings + 100
and the assignment operator =
, you can use an assignment operator joined with the arithmetic operator +=
:
// spelled out version
savings = savings + 100;
// short version
savings += 100;
The rest of the short variations are:
+=
addition-=
subtraction*=
multiplication/=
division
Try it out for yourself!
Ready to get started? To access the exercise, click this link.
Go beyond number arithmetic
In the current example, all the variables store amounts of money, which are numbers.
What kind of other values you can assign to variables?
The good news is that you can store any kind of data in variables! 😀
So far, you've seen the following components of a variable: name and value. Finally, to store different content in the boxes (or jars), you need to define the variable's type. Just like storing money requires a different container from the one needed for a book, different variables also use different storage space according to their type.
Let's say you are working on a writing application and need to analyze some text and calculate the following:
The number of vowels present in the text.
The percentage of vowels.
You could break the process down into the following:
Ask user for some text. 🆒
Examine the text supplied by the user character by character. 🔍
Increment your total every time you find a vowel. ➕
Divide the final number of vowels by the total number of characters in the string to get the percentage.➗
Multiply the result by 100 for the final percentage. ✖️
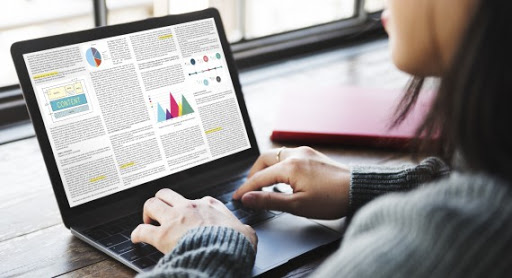
How many variables do you need to make this happen? Think about how many individual pieces of information you need to store:
The initial sequence of characters you ask the user for (a string).
The total of the number of vowels in the string.
The percentage of vowels.
It looks like you need three variables! To define each of them, you need the following components:
A type, which will define what kind of variable you have: string (text), integer (whole number), or a decimal number (floating point).
A name, so you can find the information later.
An initial value, which will give you a starting point.
From the looks of it, you'll need all three!
In C#, you can declare your three variables like this:
string text = "A wonderful string that consists of multiple characters";
int numberOfVowels = 0;
double percentageOfVowels = 0.0;
Notice that when you assign a value to a variable at its declaration, you need to explicitly indicate which type the variable is. Do this by adding the type in your declaration using keywords int
, string
, double
.
Values that never change
So far, most of the values have changed depending on circumstances (adding money to savings and increasing the vowel count). However, certain values stay as they were initially defined and can't be altered.
A named value that cannot change is called a constant. Just like variables, constants have three components: type, name, and value. The primary difference is that once you define it, the value of a constant cannot be changed.
Using constants is helpful for two reasons:
They allow programs to go faster. The computer knows how much space a constant takes. That means that when it performs operations, it doesn't have to check for alternative values.
Make sure that certain values don't change, either intentionally or by accident. For example, you wouldn't want to alter the days of the week or the number of days in a year. 📅
Let's declare some constants and see how they function. To declare a constant in C#, you need to use the keyword const
:
const int numberOfWeekdays = 7;
const string myFavoriteFood = "Ice cream";
int numberOfPets = 1;
string currentSeason = "Winter";
There are variables and constants in the example above. If you try to change the values of all of them, only variables will pass, and constants will generate errors:
numberOfWeekdays = numberOfWeekdays + 1; // Error
myFavoriteFood = "Cake"; // Error
numberOfPets = 3; // Ok
currentSeason = "Summer"; // Ok
A constant can be assigned the value of another constant but cannot be assigned the value of a variable.
const string iceCream = "Ice cream";
const string myFavoriteFood = iceCream;
string iceCream = "Ice cream";
const string myFavoriteFood = iceCream; // Error
If you compare how variables and constants work in more than one language, you'll probably notice some differences. Those are important to know as you start programming in a specific environment. However, you'll also observe lots of similarities. Though variables and constants can look different from one language to another, the concepts remain the same. Keep this in mind if you decide to start programming in a different language.
Try it out for yourself!
Ready to get started? To access the exercise, click this link.
Let's recap!
In this chapter, you learned the basics of variables and constants:
Variables and a constants are composed of three elements: type, name, and value.
The value of a variable can be modified.
A constant is a named value that cannot change.
Naming variables and constants should follow common naming conventions.
In the next chapter, we'll dive into the subject of data types! 💫