Why do we need data types?
We explored a little bit about data types in the last chapter, but there's so much more to know! Why do you need data types in the first place, though?
First off, the only way to declare a variable in C# is to specify its type directly. Let's look at the following declaration of the variable count
:
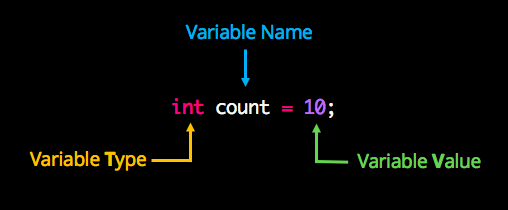
Start with the type, then the name, then the value. If you don't have a value to assign to your variable at the time of declaration, you can omit the assignment operator (=
) and value. If you want to count words in a sentence, but don't know which sentence yet, indicate the type and declare the variable for future use. However, when you declare a variable by specifying its type only, the variable cannot be used until it is assigned a value. In programming, it's often the case that you need to declare a variable knowing that you'll assign a value to it later in the program.
If we can't use a variable without a value, why would we need to specify the type at all? Why not just the value?
When running a program, your CPU (central processing unit) will need to know how much space to reserve for your variable. Declaring it with a type allows your CPU to save that space in memory for your variable depending on what type it is.
We will be exploring primitive data types which are types that exist on their own, kind of like atoms. The simplest types, they are used as a foundation for all computer operations. You can also combine them to construct more complex types, as you will see in the next chapter. We often use numeric types and strings. Let's check them out!
Numeric types
These are:
Integers, which are whole numbers, like the numbers you use to count (1, 2, 3).
Floating-points, like those you may use to store money values (2.50 or 5.99).
Let's start with one you've gotten a little familiar with: integer! Integers are declared like any other variable; with the type, then the name, then the value (if you have it):
int count = 10;
You recognize that type keyword int
from last chapter, right? That's because you declare integers with the type keyword int
. That's right, your variable count
has an integer type. As you can see, its value is 10, which is, of course, an integer. 😉
For floating-point, C# uses three types:
float
double
decimal
The float
type approximates up to 6-9 digits, double
approximates between 15-17 digits, and decimal
approximates between 28-29 digits. The decimal
type has more precision, but a smaller range as compared to double and float and is primarily used for financial transactions.
When should I use these?
Use float
/double
for measured values and decimal
for counted values. For example, you measure distance and count money.
These are declared and initialized as follows:
float length = 1876.79F;
double width = 1876.79797657;
decimal cost = 300.5M;
If you want a numeric literal to be treated as a float, use the suffix “F” or “f”. If you do not, you would get an error because C# uses double as the default numeric assignment.
Same goes for decimal
which uses the suffix “M” or “m” and double which uses “D” or “d” when you wish to have an integer treated as a double.
What happens if you supply larger values? For example:
double a = 1876.79797657765609870978709780987;
float b = 1876.79797657765609870978709780987F;
They will both lose some precision, but not the to the same degree:
// a -> 1876.79797657766
// b -> 1876.798
With smaller values, it makes no difference:
float length = 10.0F;
double width = 10.0;
In the example above, both variables hold small values. We are just using double
instead of float
in the second statement. As it doesn't make any functional difference, you would be encouraged to use the smaller one in many languages, since using a bigger box to store small values wastes memory!
Mixing numeric types
An important thing to keep in mind is how the types mix together and what consequences it may have. For example, if you divide two numbers: 5/2
, you would logically expect the result to be 2.5
. To the computer, however, it's not that obvious and you might unexpectedly get 2
instead!
Let's review a few examples in C#:
int a = 10;
int b = 4;
int c = a / b;
Guess what the result of the division will be?
Well, it's 2
- not necessarily what you'd expect. Variables a
and b
were assigned integer numbers (whole numbers), so the division operation can only provide an integer as an answer.
Let's explore this concept further.
What do you think the expected result of this variation would be?
int a = 10;
int b = 4;
double c = a / b;
This time around, c
is going able to store a double
number. However, the a/b
expression still divides an int
with another int
. So c
will be assigned the result of the integer division. That's 2, again.
The next thing you need to know is how to work with variables of different types that need to be used together for an action. For example:
int a = 10;
double b = 4;
int c = a / b;
See how one variable has been declared an int
and the other a double
?
The result of the
a/b
expression will be2.5
.However,
c
is declared as anint
and cannot be assigned adouble
value. This code just won't work at all!
What if you change your mind and want to make b
perform like an int
variable in the middle of the program?
You can make a variable of one type act like another type. This is called type casting. To solve the problem in the last example, make variable b
pretend that it's an integer by assigning it to c
like below:
int a = 10;
double b = 4;
int c = a / (int) b; //-> c contains 2, as a/(int) b is an integer division
See how variable b
acts like an integer? You can also make an integer variable b
pretend that it's value is a double
:
int a = 10;
int b = 4;
double c = a / (double) b; //-> c contains 2.5, as the value in b is casted to a double
Casting one variable to double
lets you perform a floating point division even if you are using variables with an int
type.
Try it out for yourself!
Ready to get started? To access the exercise, click this link.
Strings
Next, we are going to check out a more sophisticated type: string. Strings allow for storing text or, in other words, a set of characters. Here's how to declare a string variable in C#:
string city = "New York";
string movie = "Best ever";
string pet;
string emptyString = "";
One of the common actions you can perform on strings is merging one or more of them together. Let's join some strings together:
string firstFavoriteCity = "New York";
string secondFavoriteCity = "Buenos Aires";
string favorites = firstFavoriteCity + secondFavoriteCity; // -> "New YorkBuenos Aires"
Notice, there is no space in between. Let's make it more readable by concatenating strings and variables:
string firstFavoriteCity = "New York";
string secondFavoriteCity = "Buenos Aires";
string favorites = "My favorite cities are " + firstFavoriteCity + " and " + secondFavoriteCity; // -> "My favorite cities are New York and Buenos Aires"
Much better now! You can also concatenate other data types with strings, such as numbers. How do you do that?
string favoriteCity = "Buenos Aires";
int numberOfTrips = 5;
string story = "I've traveled to " + favoriteCity + " " + numberOfTrips + " times!"; // -> "I've traveled to Buenos Aires 5 times!"
In the last section, we used the +
operator to add two numbers. Here, with strings, the +
operator can be used to concatenate strings and integers together. Concatenation refers to joining strings or numbers and strings together.
Try it out for yourself!
Ready to get started? To access the exercise, click this link.
Type is required
As mentioned before: variables always have a type. C# requires you to either specify its type or use the variable keyword to let the compiler infer the type. If you need to use the content of a variable as if it was of another type, you can use type casting.
For example:
var limit; //-> will cause an error: Implicitly-typed variables must be initialized.
var limit = 3; //-> compiler infers this is an int.
Let's recap!
In this chapter, you've learned the specifics of a few simple data types:
integer (keyword
int
)floating-point (keywords
float
,double
, anddecimal
)string (keyword
string
)
You've also learned how to manipulate these types:
You can perform numeric operations on numbers of the same type.
To use numbers of different types in operations together, cast them to behave like the needed type.
Strings can be joined together. This is called concatenation.