The purpose of functional testing is to check that the functional behavior of the application is in accordance with the specification, by automatically simulating user actions. Functional testing is carried out to ensure that the features and services provided by the application will function correctly during use. For example, you’ll check that a user can sign up, log in, change their profile, change their password and log out.
Here are the steps involved in setting up functional testing:
Identify the function you wish to test: general scenario or error condition.
Configure the input data based on the requirements in the specification.
Define the expected output parameters based on the requirements in the specification.
Run the test.
Compare the test outputs with the expected results to check if the test has passed.
Test a Web Application
In this chapter, we’re going to see how to set up a functional test for a web application. As a minimum, we need to understand the expected HTTP response for each feature.
Here are the steps you need to follow for a web application:
Create an HTTP client using a webdriver.
Send an HTTP request to the page you want to test.
Retrieve the contents of the HTTP response.
Check that the page and the data are in accordance with the specification.
How do we do it?
When you do a bit of research on the internet, you’ll see that the word Selenium often pops up. Which is perfect, because we’re going to use this exact framework for our functional testing.
Selenium is one of the most popular test automation tools because it has a highly flexible framework:
Selenium tests can be written for all popular programming languages.
Tests can be run on any operating system.
Tests can be created for different browsers.
The framework can be linked to other testing tools.
Configure Selenium
You need to install Selenium before you can use it. To do this, use PyPI by running the following command on the terminal:
pip install selenium
The framework also requires a driver to be installed. This will interface with a web browser, such as Chrome, Firefox, Edge, or Safari. Each browser has its own driver. Here are the links to download the driver for whichever browser you’re using:
Chrome | |
Firefox | |
Safari | https://webkit.org/blog/6900/webdriver-support-in-safari-10/ |
Edge | https://developer.microsoft.com/en-us/microsoft-edge/tools/webdriver/ |
Just download the webdriver for now using one of the above links. We’ll see where to copy the executable code later on in the project. If you need more details, you can read the documentation.
Write a Functional Test
Let’s go back to the OC-commerce project using the Django framework to set up our first functional test—you can then create the rest of the tests on your own.
First of all, you need to create a package containing the driver that you downloaded for your browser as well as all of the test modules that you’re going to deploy.
For example, you could create a functional_tests
package in the test folder structure.
Next, you’re going to create an initial test module containing all testing relating to the authentication functionality. We’ll call this test_authentication.py
.
You should now see the following folder structure:
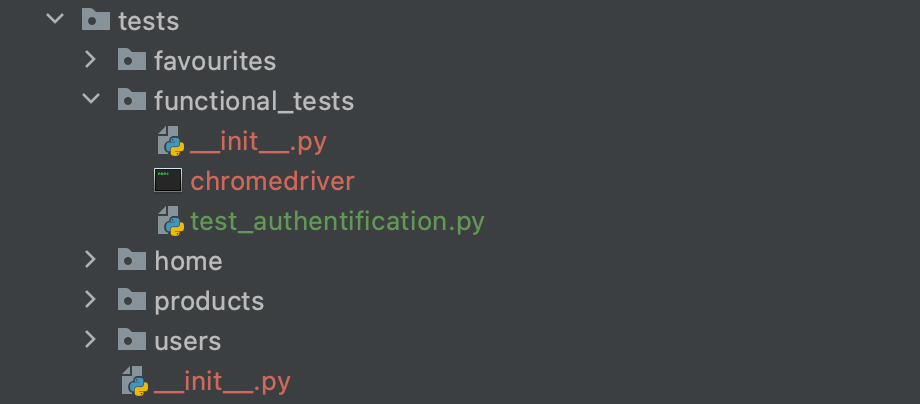
All functional tests will be deployed as methods of a sub-class of StaticLiveServerTestCase
. Here’s a test that will open Chrome on the homepage for our application and will check that everything’s working correctly using the webdriver.
from selenium import webdriver
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
import time
class TestAuthentification(StaticLiveServerTestCase):
def test_open_chrome_window(self):
self.browser = webdriver.Chrome("tests/functional_tests/chromedriver")
self.browser.get(self.live_server_url)
time.sleep(30)
self.browser.close()
Now you can run the following command on the terminal to run the full suite of tests: python manage.py test
.
Wow! As if by magic, the Chrome page has opened!
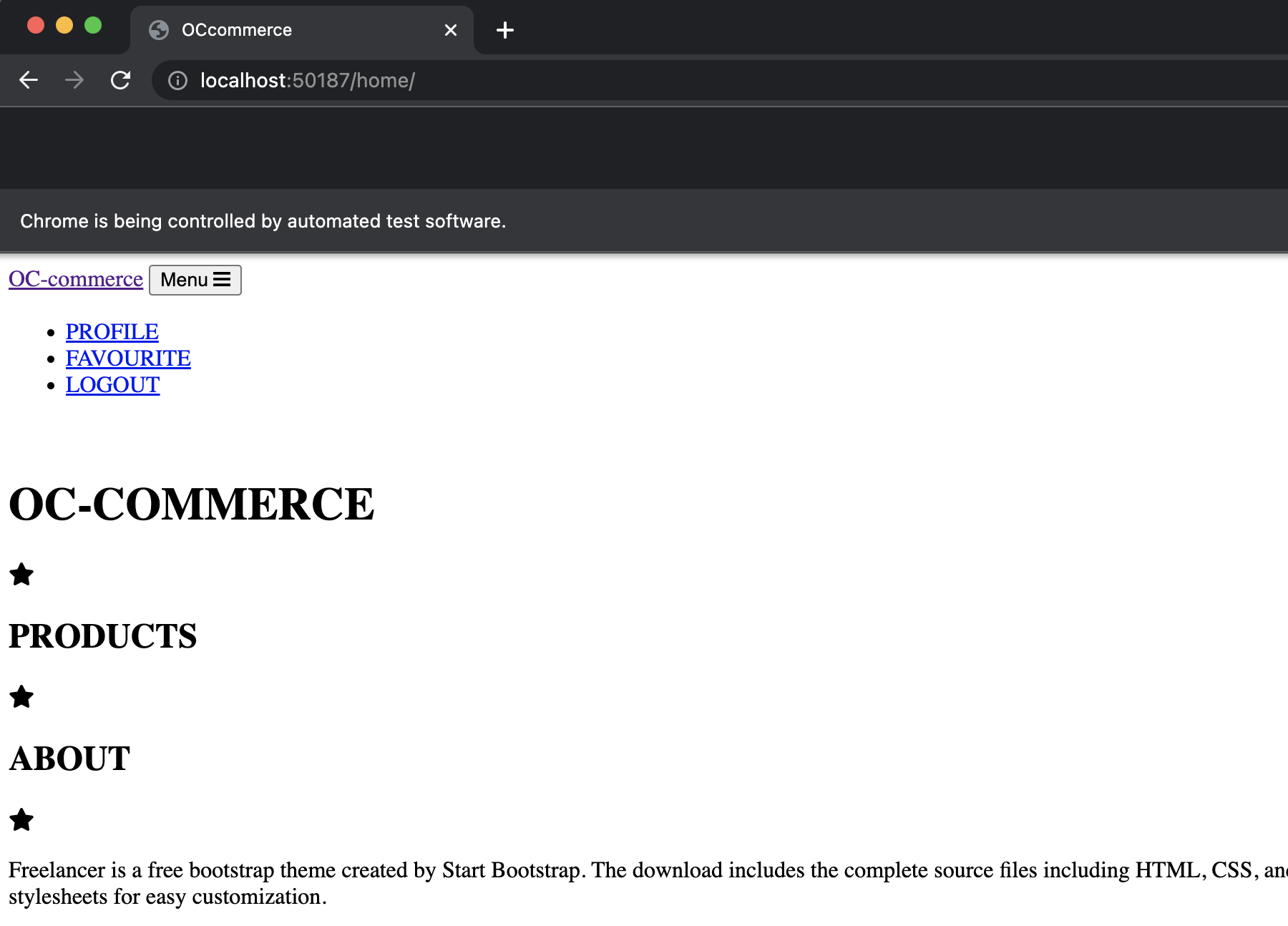
It’s all very well using Selenium to open web pages, but what can we do to actually test our functionality?
Well, now we’re going to create a functional test that will check if a new user can sign up for the application. To do this, we’ll need to access the signup page and fill in the form. Fortunately, the Selenium framework provides functions that will find the page elements that require inputs and submit the form.
Here’s the functional test that will test the user signup process:
from selenium import webdriver
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
from django.urls import reverse
class TestAuthentification(StaticLiveServerTestCase):
def test_signup(self):
#Open the browser using webdriver
self.browser = webdriver.Chrome("tests/functional_tests/chromedriver")
self.browser.get(self.live_server_url + reverse("signup"))
fname = self.browser.find_element_by_id("fname")
fname.send_keys("Ranga")
lname = self.browser.find_element_by_id("lname")
lname.send_keys("Gonnage")
username = self.browser.find_element_by_id("username")
username.send_keys("rgonnage")
email = self.browser.find_element_by_id("email")
email.send_keys("test@test.com")
password1 = self.browser.find_element_by_id("pass")
password1.send_keys("ranga12345")
password2 = self.browser.find_element_by_id("re_pass")
password2.send_keys("ranga12345")
agree_term = self.browser.find_element_by_id("agree-term")
agree_term.click()
signup = self.browser.find_element_by_id("signup")
signup.click()
self.assertEqual(self.browser.find_element_by_tag_name('h2').text, "Log In")
self.assertEqual(self.browser.current_url, self.live_server_url + reverse("login"))
#Close browser
self.browser.close()
Let’s look at these lines of code in more detail:
driver.get()
: navigates to a page passing the URL as a parameter (see documentation: Navigating).driver.find_element_by_tag_name()
: locates a page element using its id. However, there are other functions that will find page elements (see documentation : Locating elements).element.send_keys()
: inputs text or simulates a keystroke usingsend_keys()
on the selected element.element.click()
: simulates a mouse click on an element.
The first assertion checks that we’re correctly redirected to the login page, by checking that the title in the h2
tag isLog In
, while the second assertion checks that the current URL matches the login page URL.
Over to You!
Now that we’ve seen how to set up a functional test using Selenium, why not keep up the good work and create the following functional tests:
Log in to the application.
Open a product modal.
Add a product to favorites.
Delete a product from favorites.
Log out of the application.
Access profile information.
Feel free to add more tests if you have any ideas, because there are plenty more that could be created.
Find a suggested solution on GitHub!
Let’s Recap!
Before you can use Selenium, you need to download the relevant webdriver for the browser you’re using.
Functional testing ensures that the application functionality is in accordance with the specification.
The Selenium framework is used to automate functional testing for web applications.
The framework provides functions for navigating and interacting with the web application so that you can check its behavior.
This chapter wasn’t easy, especially the part about Selenium configuration, but you’ve seen how powerful the framework can be. Don’t worry, the next framework we’re going to see is much easier to use. Meet me in the next chapter, where we’ll look at performance testing.