Display a New Page in Five Lines of Code!
We’re going to create a new page on the site by writing just five lines of code. It will be a very basic page, for sure, but it will be a page nonetheless!
Start by opening up listings/views.py. At the moment, it looks like this:
# ~/projects/django-web-app/merchex/listings/views.py
from django.shortcuts import render
# Create your views here.
Delete the comment and replace it with a simple function. Also add an additional import statement at the top:
# ~/projects/django-web-app/merchex/listings/views.py
from django.http import HttpResponse
from django.shortcuts import render
def hello(request):
return HttpResponse('<h1>Hello Django!</h1>')
You just created a view - one of the building blocks of MVT architecture.
A view responds to a user’s website visit with a page they can see.
Or, in Pythonic terms:
A view is a function that accepts an HttpRequest
object as a parameter and returns a HttpResponse
object.
In the example view, you return an HTTP response with some simple HTML content: an H1 header saying “Hello Django!”
Now let’s open up merchex/urls.py. Right now, the code (minus the comments) looks like this:
# ~/projects/django-web-app/merchex/merchex/urls.py
from django.contrib import admin
from django.urls import path
urlpatterns = [
path('admin/', admin.site.urls),
]
Let’s import the views module edited in the previous step by adding an import statement. Then add a new item to the urlpatterns
list referencing the newly created view function.
# ~/projects/django-web-app/merchex/merchex/urls.py
from django.contrib import admin
from django.urls import path
from listings import views
urlpatterns = [
path('admin/', admin.site.urls),
path('hello/', views.hello)
]
You just created a URL pattern and mapped it to the view. A URL pattern is how you tell Django that it should listen for a particular URL request and then call a particular view to generate a page for the user.
Now any time someone visits the 'hello/'
URL on our website, the hello
view will generate a page for them to see. And that’s what you’re going to try now.
Open your browser and type in the URL http://127.0.0.1:8000/hello/.
And that’s your first Django page! How quick and easy was that?
Now that you’ve tried it, watch me go through the process to make sure you’ve understood:
Next, let’s add another view to your web app so that users can visit one more page. This time we’ll look at each step more closely. And then, at the end of the chapter, you’ll add another two views on your own, so you can practice what you’ve learned.
Understand That It All Begins With a URL
Every website interaction begins with a URL. You can type it into your address bar directly, choose it from a bookmark, or click a link from another page or site - but the result is the same: you have to get a URL into your address bar before anything else can happen. For example:
https://www.merchex.xyz/about-us/
Behind the scenes, your browser begins working out what to do next. First, it needs to know the IP address of the server that is hosting the website. It works this out by doing a DNS lookup on the domain name or hostname - that's everything between ‘://’ and the first ‘/’: www.merchex.xyz.
Now that the browser knows where the server is, it prepares an HTTP request to send there. The request contains:
A method - in this case,
GET
. You can think of this as meaning “get me this page please!”The path of the URL - that’s everything from the first single slash (‘/’) onwards: /about-us/.
When the request arrives at our server, the application’s job is to figure out what the request is asking for. It does this by looking at the path.
Here are some URL paths that you may see in HTTP requests to the web application:
/about-us/
/contact-us/
/help/
Django needs a way to distinguish between these paths so it can respond with the correct content.
And that’s where URL patterns come in. Let’s see how they work.
Match a URL With a URL Pattern
Open up the file merchex/urls.py
. In this file, you'll define a list of URL patterns. Every time Django receives an HTTP request, it will go through these patterns in order, one by one, and try to find a match.
# ~/projects/django-web-app/merchex/merchex/urls.py
urlpatterns = [
path('admin/', admin.site.urls),
path('hello/', views.hello),
]
Here, you can see the URL pattern created in the previous step matching the ‘hello/’
path .
Let’s add a third URL pattern to this list for a new about us page. Add a new line of code to the urlpatterns
list:
# ~/projects/django-web-app/merchex/merchex/urls.py
urlpatterns = [
path('admin/', admin.site.urls),
path('hello/', views.hello),
path('about-us/', views.about), # add this line
]
What are the purposes of the arguments passed to the path
function?
See if you can understand the purpose of each argument before reading on.
The first argument is a string. This is the URL path we’re going to match: about-us/.
The second argument should be a view function we defined in views.py. The request will be passed onto this view, and the view will generate a page.
When a URL has been matched to a URL pattern, responsibility moves on to the next stage: the HTTP request is passed along to the specified view.
Return an HTTP Response With a View
It’s now the view’s job to generate an HTTP response that can be sent back to the browser.
Let’s create the view that we referenced in our URL pattern. Open listings/views.py
. Add another view function underneath the one created earlier in this chapter:
# ~/projects/django-web-app/merchex/listings/views.py
from django.http import HttpResponse
from django.shortcuts import render
def hello(request):
return HttpResponse('<h1>Hello Django!</h1>')
def about(request):
return HttpResponse('<h1>About Us</h1> <p>We love merch!</p>')
Views are Python functions that must fulfill these criteria:
The function's first parameter is a variable containing the
HttpRequest
object, and by convention, you always name itrequest
. This object contains some useful attributes related to the request. We’ll look into those in Capture User Input With Django Forms (P4C2).The return value of the function is always an
HttpResponse
object. What it contains depends on the type of application - in our app, we’re going to package HTML into our responses.
Great. Our view function has returned an HTTP response. The last thing Django does is send this response back to the browser that made the original request. Django has done its job, and now the browser to displays the page. Shall we test it out?
Check to see if your server is running (start or restart if necessary), and then visit http://127.0.0.1:8000/about-us.
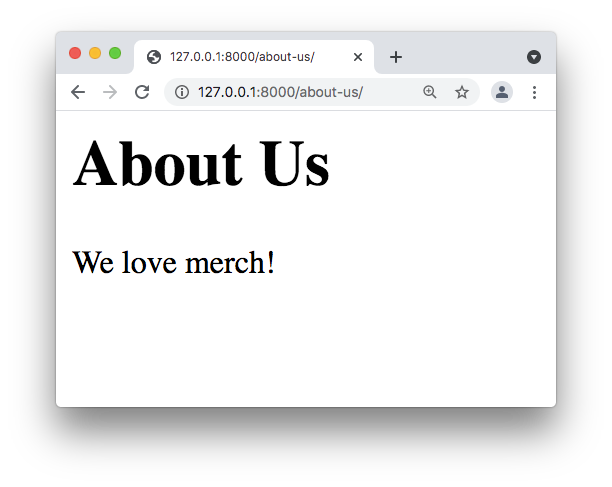
And we have another page on our site!
If you’re still confused about how URL patterns and views work together, this video should help to explain:
So, I understand that views are functions, and we’ve written two of them, but I don’t remember calling these functions in any of the code. So how is this even working?
That’s a great question. The answer is that the Django framework is calling these view functions on your behalf. So all you had to do was specify (in urls.py) the function that should be used for each URL pattern. Django takes care of the rest.
This is an example of what we call magic in programming frameworks - the things the framework does “under the hood” that, on the surface, seem to break the programming rules. They can seem unintuitive, to begin with, but they will soon become second nature.
This seems strange to me: we visited http://127.0.0.1:8000/about-us in the browser, but there is no file in my project called about-us. Usually there must be a file somewhere, perhaps called about.html or about.php, but not in Django. How does this work?
If you’re familiar with directly accessing .html or .php files in the browser, then Django might seem odd. But stick with it. Throughout the course, you will see how Django serves content without the need for a physical file for each page.
Now You Try! Add Pages to Your Site With URL Patterns and Views
I’ve shown you how to add new pages to your site using URL patterns and views. Now use what you’ve learned to add two new pages to your app. These are pages that you’ll build on in later chapters.
The two pages will be a “listings” page (where you’ll eventually show a list of merch listings) and a “contact us” page (where you’ll build a contact web form). Right now, these pages can be very simple - just a <h1> tag and a <p> tag. You’ll build the full content later in the course.
Visit the pages you've created in the browser to check they display correctly.
Let’s Recap!
When Django receives an HTTP request, it attempts to find a match for the path of that request in a list of URL patterns, defined in urls.py.
If the path matches a URL pattern, Django passes the request to the corresponding view, which you define in views.py.
The view is a function that accepts the request as a parameter and returns an
HttpResponse
as its return value. This response contains the HTML that the browser uses to display the page.
Now that you have the view - the ‘V’ of our MVT architecture - you're ready to display data in your pages with a model.