What Is a Variable?
Let’s say you’re moving to a new home and you need to pack up the stuff in your house into boxes. You’d want a box for dishes, one for clothes, and one for the television. When you pack the boxes, the smart thing to do would be to add a label - that way, you know what’s inside.
A variable is like a box - it’s just a way to store your data. As a developer, you’ll need to save certain bits of information and assign it a label so that you can retrieve the information later. A user name, available tickets left for a flight, the day of the week, inventory - and all of this data are stored in variables.
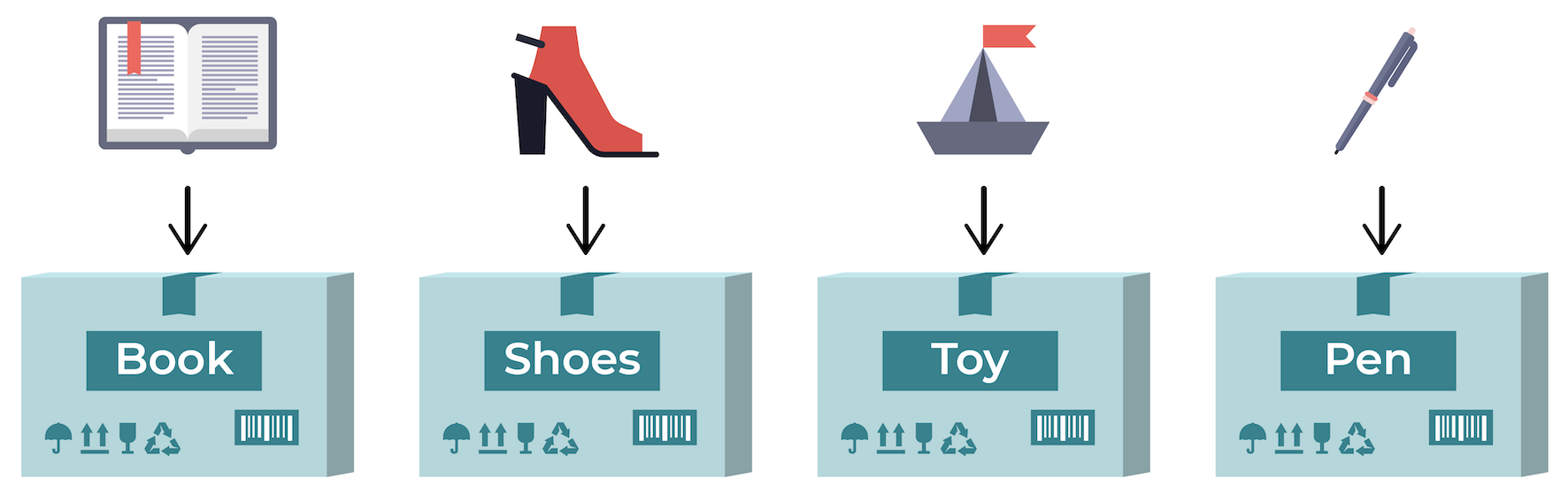
Declaring a variable is super easy in Python. The code below declares a variable.
book = "The Great Gatsby"
Three components describe a variable in Python: name, type, and value. We’ll cover name and value in this chapter, and type in the next.
In this situation, book
would be the variable name or the label of the box, and "The Great Gatsby"
would be the variable value, or what’s in the box. Then, if you wanted to retrieve the title of the book using Python, you could type book
, and you would get "The Great Gatsby"
in return.
Modify a Variable
Modifying the value of a variable is super easy. It’s as simple as assigning the same variable name a new value. Here’s a code snippet that declares book
as The Great Gatsby
and then modifies the variable value to Beloved
.
>> book = "The Great Gatsby"
>> print(book)
The Great Gatsby
>> book = "Beloved"
>> print(book)
Beloved
Now try out this quick exercise to make sure you’re following along:
>> favorite_food = "ice cream"
>> new_favorite_food = "pizza"
>> favorite_food = "pasta"
>> print(favorite_food)
What will this program output? Take a minute to think about it before looking below!
>> print(favorite_food)
pasta
If you guessed pasta
, you are correct! The code snippet declares two variables, favorite_food
and new_favorite_food
. It then sets favorite_food
to be equal to pasta
, so it doesn’t matter what favorite_food
was declared as earlier.
Name a Variable
A variable's name should reflect the meaning of its contents like labels on boxes. Here are some general recommendations for creating names:
Use descriptive names throughout your code.
Did you ever fish an old folder out of a box labeled "admin stuff" on the cover? Remember how frustrating that was? Being descriptive and specific in variable names makes life simpler, and your code easier to read and maintain. Instead ofamount
(or even worse,amt
), add some detail:quantity_in_stock
,current_balance
, etc.Spell it out.
Avoid abbreviating or shortening words when possible, even if a shorter version seems obvious. For example,annual_revenue
is better thanannual_rev
.Follow a common naming convention.
One of the popular naming conventions for Python is called snake case — names made up of multiple words with underscores between the words, e.g.,number_of_cats, final_answer
,the_best_python_programmer_ever
, etc.Start with a letter or the underscore character.
A variable name cannot start with a number.Use only alphanumeric characters and underscores.
For example,hello_1
but nothello_#1
.Be aware that variable names are case-sensitive.
age
,Age
, andAGE
are three different variables.
Level-Up: Use Basic Python Syntax to Declare a Variable
Context:
In this exercise, you will explore variable manipulation in Python focusing on a specific case. You will learn how to create a variable to store an age, modify it if needed, and display it using the powerful tool known as f-string. This approach will help you understand how to define, modify, and display variables effectively and elegantly using the features provided by Python.
Instructions:
Create a variable named
name
and assign your name to it.Create a variable named
age
and assign your age to it.Display a sentence containing your name and age using the variables you just created. For example,
"My name is John and I am 25 years old."
Modify the value of the
age
variable to add 10 years to your current age.Display the sentence containing your name and updated age using the updated variables. For example,
"My name is John and I am now 35 years old."
Let’s Recap!
A variable has a name and value, similar to a box with a label and the stuff inside.
You can declare a variable with the variable name (e.g.
book
), an equals sign=
, and the value (e.g.,"The Great Gatsby"
). The value may or may not need to be in quotation marks, depending on what type of data it is (which we'll cover in the next chapter).Keep best practices in mind when naming a variable.
Now that you know you can store your data with variables, you’ll need to know how to classify your data with data types.