When to Use Loops
In programming, there are sets of instructions you will need to repeat multiple times. For example, if you want to perform the same task on every item in a list. What if you have a list of campaigns, and you want to print every one of them?
When you need to repeat a set of instructions, sometimes you know the number of repetitions in advance; other times, you don't. There are also times when the number of repetitions does not matter, and you want to repeat the code until a certain condition is met.
For all of these purposes, you use loops.
The for
Loop
The for
loop is the core type of looping in Python. A for
loop is used to iterate over any sequence. That can be a list, tuple, dictionary, or even a string. With a for
loop, you can execute the same code for each element in that sequence.
Python makes it very easy to loop through every element of a sequence. If you want to print every element in a list, it will look like this:
dog_breeds = ["golden retriever", "chihuahua", "terrier", "pug"]
for dog in dog_breeds:
print(dog)
In this code, each element in dog_breeds
will be printed to the terminal. dog
is a variable name that updates to be the next element every time the loop repeats. Theoretically, you could change dog
to cockadoodledoo
and it would still perform the same functionality.
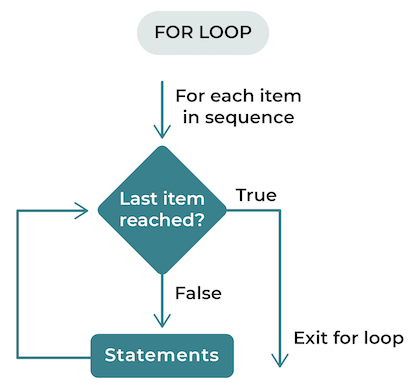
You can do the same type of for
loop if you want to loop over every character in a string.
To loop through a set of code a certain number of times, you can use the range()
function, which returns a list of numbers starting from 0 to the specified end number.
for x in range(5):
print(x)
This code will print 0, 1, 2, 3, 4 sequentially.
for x in range(100):
print(f"{x} bottles of beer on the wall!")
The curly brackets {}
here will take whatever value is in variable x
and put it in its place. So in this example, the code will print:
0 bottles of beer on the wall!
1 bottles of beer on the wall!
…
99 bottles of beer on the wall!
The range()
function defaults to 0 as a starting value, but you can change it by adding another integer like so: range(4, 10)
!
This range would return values from 4 to 10 (but not including 10).
The while
Loop
While the for
loop lets you execute code a certain specified number of times, the while
loop keeps executing until a certain condition is met.
In the previous chapter, you learned about conditions as statements that evaluate to true or false. The same applies here: code within a while
statement will keep executing until the condition becomes false.
The code snippet below checks the current capacity and increases it by 1 until the maximum capacity is reached (+= 1
increases the current value by 1).
maximum_capacity = 10
current_capacity = 3
while current_capacity <= maximum_capacity:
current_capacity += 1
Because the current_capacity
starts at 3, this code executes 7 times until current_capacity
hits 10.
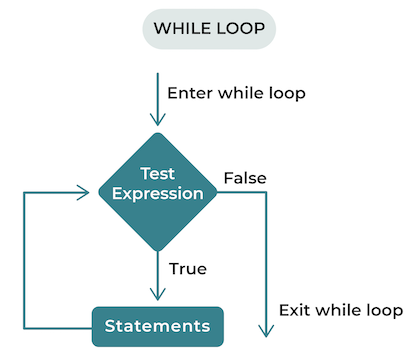
It’s important to be aware of infinite loops. If the condition you set is always true, the loop will continue to run forever!
For example:
x = 0
while x != 5:
x += 2
In this situation, x will never hit 5!
Level-Up: Loop-the-Loop
Context:
Now you are going to write a program that calculates various statistics for a set of numbers provided by the user. This task will allow you to review lists and address the new concept of this chapter: loops.
Instructions:
Ask the user to enter a list of numbers separated by commas (e.g., 1,2,3,4), and display this list.
Calculate and display the sum of the numbers in the list.
Calculate and display the average of the numbers in the list.
Calculate and display the number of numbers in the list that are greater than the average.
Calculate and display the number of numbers in the list that are even.
Once you have completed the exercise, you can run the following command in the VS code terminal pytest tests.py
Let’s Recap!
Loops let you easily repeat tasks or execute code over every element in a list.
A
for
loop enables you to repeat code a certain amount of time.A
while
loop lets you repeat code until a certain condition is met.
Loops are great to help you repeat code easily. Next, we’ll dive into functions; another way to help you bundle repeatable tasks.